DMA-enabled high data rate driver for Heroic Robotics LED strips.
Embed:
(wiki syntax)
Show/hide line numbers
FastPixelLPD8806.h
00001 /***************************************************************************/ 00002 // DMA-enabled high data rate driver for Heroic Robotics FastPixel strips. 00003 // Written by Jas Strong <jasmine@heroicrobotics.com> 00004 // Copyright (c) 2013-2014 Heroic Robotics, Inc. 00005 // 00006 // FastPixel is a family of high performance LED strips available from 00007 // Heroic Robotics, Inc. http://heroicrobotics.com/ - check them out! 00008 // 00009 // These strips are capable of supporting 18 Mbit/sec when run on PixelPusher 00010 // hardware; your performance on mbed is likely to be somewhat lower due to 00011 // the lack of high performance driver chips on the SPI lines. 00012 // 00013 // Heroic Robotics also sells a family of dedicated LED controllers called 00014 // PixelPusher- almost certainly the best LED controller in the world. 00015 // 00016 // Permission is hereby granted, free of charge, to any person obtaining a copy 00017 // of this software and associated documentation files (the "Software"), to deal 00018 // in the Software without restriction, including without limitation the rights 00019 // to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00020 // copies of the Software, and to permit persons to whom the Software is 00021 // furnished to do so, subject to the following conditions: 00022 // 00023 // The above copyright notice and this permission notice shall be included in 00024 // all copies or substantial portions of the Software. 00025 // 00026 // THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00027 // IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00028 // FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00029 // AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00030 // LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00031 // OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00032 // THE SOFTWARE. 00033 // 00034 // Some portions originally from: 00035 // 00036 // Mbed library to control LPD8806-based RGB LED Strips 00037 // (c) 2011 Jelmer Tiete 00038 // This library is ported from the Arduino implementation of Adafruit Industries 00039 // found at: http://github.com/adafruit/LPD8806 00040 // and their strips: http://www.adafruit.com/products/306 00041 // Released under the MIT License: http://mbed.org/license/mit 00042 // 00043 // Parameterized and modified to use soft SPI. 00044 // Jas Strong <jasmine@electronpusher.org> 00045 // Then remonstered to use hardware SPI for blast mode. 00046 /*****************************************************************************/ 00047 00048 // Heavily modified by Jas Strong, 2012-10-04 00049 // Changed to use a virtual base class and to use software SPI. 00050 00051 #include "mbed.h" 00052 #include "LedStrip.h" 00053 #include "MODDMA.h" 00054 00055 #ifndef MBED_FastPixelLPD8806_H 00056 #define MBED_FastPixelLPD8806_H 00057 00058 class FastPixelLPD8806 : public LedStrip { 00059 00060 public: 00061 00062 FastPixelLPD8806(PinName dataPin, PinName clockPin, int n); 00063 virtual void begin(void); 00064 virtual void show(void); 00065 virtual void blank(void); 00066 virtual void setPixelColor(uint16_t n, uint8_t r, uint8_t g, uint8_t b); 00067 virtual void setPackedPixels(uint8_t * buffer, uint32_t n); 00068 virtual void setPixelB(uint16_t n, uint8_t b); 00069 virtual void setPixelG(uint16_t n, uint8_t g); 00070 virtual void setPixelR(uint16_t n, uint8_t r); 00071 virtual void setPixelColor(uint16_t n, uint32_t c); 00072 virtual uint16_t numPixels(void); 00073 virtual uint32_t Color(uint8_t, uint8_t, uint8_t); 00074 virtual uint32_t total_luminance(void); 00075 00076 private: 00077 SPI _spi; 00078 void write(uint8_t byte); 00079 uint8_t *pixels; // Holds LED color values 00080 uint16_t numLEDs; // Number of RGB LEDs in strand 00081 MODDMA_Config * dma_config; 00082 int strip_num; 00083 00084 00085 uint32_t clock_mask; 00086 uint32_t data_mask; 00087 00088 }; 00089 #endif
Generated on Sat Jul 16 2022 03:35:31 by
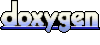