Port of the nRF24l01+ library of these dudes. Not GPLed, so yeah, you can use it. Copyright (c) 2007 Stefan Engelke <mbox@stefanengelke.de> Some parts copyright (c) 2012 Eric Brundick <spirilis [at] linux dot com>
Enrf24.h
00001 /* nRF24L01+ I/O for mbed 00002 * Ported by Jas Strong <jasmine@heroicrobotics.com> 00003 * 00004 * Copyright (c) 2013 Eric Brundick <spirilis [at] linux dot com> 00005 * Permission is hereby granted, free of charge, to any person 00006 * obtaining a copy of this software and associated documentation 00007 * files (the "Software"), to deal in the Software without 00008 * restriction, including without limitation the rights to use, copy, 00009 * modify, merge, publish, distribute, sublicense, and/or sell copies 00010 * of the Software, and to permit persons to whom the Software is 00011 * furnished to do so, subject to the following conditions: 00012 * 00013 * The above copyright notice and this permission notice shall be 00014 * included in all copies or substantial portions of the Software. 00015 * 00016 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, 00017 * EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00018 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00019 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT 00020 * HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, 00021 * WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00022 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER 00023 * DEALINGS IN THE SOFTWARE. 00024 */ 00025 00026 #ifndef _ENRF24_H 00027 #define _ENRF24_H 00028 00029 #define ENRF24_LIBRARY_VERSION 1.5 00030 00031 #include "mbed.h" 00032 #include <stdint.h> 00033 #include "nRF24L01.h" 00034 00035 #ifndef BITF 00036 #define BIT0 (0x0001) 00037 #define BIT1 (0x0002) 00038 #define BIT2 (0x0004) 00039 #define BIT3 (0x0008) 00040 #define BIT4 (0x0010) 00041 #define BIT5 (0x0020) 00042 #define BIT6 (0x0040) 00043 #define BIT7 (0x0080) 00044 #define BIT8 (0x0100) 00045 #define BIT9 (0x0200) 00046 #define BITA (0x0400) 00047 #define BITB (0x0800) 00048 #define BITC (0x1000) 00049 #define BITD (0x2000) 00050 #define BITE (0x4000) 00051 #define BITF (0x8000) 00052 #endif 00053 00054 /* Constants for speed, radio state */ 00055 #define ENRF24_STATE_NOTPRESENT 0 00056 #define ENRF24_STATE_DEEPSLEEP 1 00057 #define ENRF24_STATE_IDLE 2 00058 #define ENRF24_STATE_PTX 3 00059 #define ENRF24_STATE_PRX 4 00060 00061 /* Internal IRQ handling */ 00062 #define ENRF24_IRQ_TX 0x20 00063 #define ENRF24_IRQ_RX 0x40 00064 #define ENRF24_IRQ_TXFAILED 0x10 00065 #define ENRF24_IRQ_MASK 0x70 00066 00067 #define ENRF24_CFGMASK_IRQ 0 00068 00069 typedef uint8_t boolean; 00070 00071 00072 00073 /* Class definition--inherits from Print so we have .print() functions */ 00074 class Enrf24 { 00075 public: 00076 boolean lastTXfailed; 00077 00078 Enrf24(PinName cePin, PinName csnPin, PinName irqPin, PinName miso, PinName mosi, PinName sck); 00079 void begin(uint32_t datarate=1000000, uint8_t channel=0); // Specify bitrate & channel 00080 void end(); // Shut it off, clear the library's state 00081 00082 // I/O 00083 boolean available(boolean checkIrq=false); // Check if incoming data is ready to be read 00084 size_t read(void *inbuf, uint8_t maxlen=32); /* Read contents of RX buffer up to 00085 * 'maxlen' bytes, return final length. 00086 * 'inbuf' should be maxlen+1 since a 00087 * null '\0' is tacked onto the end. 00088 */ 00089 virtual size_t write(uint8_t); // Single-byte write, implements TX ring-buffer & auto-send 00090 //using Print::write; // Includes the multi-byte write for repeatedly hitting write(uint8_t) 00091 void flush(); // Force transmission of TX ring buffer contents 00092 void purge(); // Ignore TX ring buffer contents, return ring pointer to 0. 00093 00094 // Power-state related stuff- 00095 uint8_t radioState(); // Evaluate current state of the transceiver (see ENRF24_STATE_* defines) 00096 void deepsleep(); // Enter POWERDOWN mode, ~0.9uA power consumption 00097 void enableRX(); // Enter PRX mode (~14mA) 00098 void disableRX(); /* Disable PRX mode (PRIM_RX bit in CONFIG register) 00099 * Note this won't necessarily push the transceiver into deep sleep, but rather 00100 * an idle standby mode where its internal oscillators are ready & running but 00101 * the RF transceiver PLL is disabled. ~26uA power consumption. 00102 */ 00103 00104 // Custom tweaks to RF parameters, packet parameters 00105 void autoAck(boolean onoff=true); // Enable/disable auto-acknowledgements (enabled by default) 00106 void setChannel(uint8_t channel); 00107 void setTXpower(int8_t dBm=0); // Only a few values supported by this (0, -6, -12, -18 dBm) 00108 void setSpeed(uint32_t rfspeed); // Set 250000, 1000000, 2000000 speeds. 00109 void setCRC(boolean onoff, boolean crc16bit=false); /* Enable/disable CRC usage inside nRF24's 00110 * hardware packet engine, specify 8 or 00111 * 16-bit CRC. 00112 */ 00113 // Set AutoACK retry count, timeout params (0-15, 250-4000 respectively) 00114 void setAutoAckParams(uint8_t autoretry_count=15, uint16_t autoretry_timeout=2000); 00115 00116 // Protocol addressing -- receive, transmit addresses 00117 void setAddressLength(size_t len); // Valid parameters = 3, 4 or 5. Defaults to 5. 00118 void setRXaddress(void *rxaddr); // 3-5 byte RX address loaded into pipe#1 00119 void setTXaddress(void *txaddr); // 3-5 byte TX address loaded into TXaddr register 00120 00121 // Miscellaneous feature 00122 boolean rfSignalDetected(); /* Read RPD register to determine if transceiver has presently detected an RF signal 00123 * of -64dBm or greater. Only works in PRX (enableRX()) mode. 00124 */ 00125 00126 private: 00127 uint8_t rf_status; 00128 uint8_t rf_channel; 00129 uint8_t rf_speed; // Stored in native RF_SETUP register format 00130 uint8_t rf_addr_width; 00131 uint8_t txbuf_len; 00132 uint8_t txbuf[32]; 00133 uint8_t lastirq, readpending; 00134 DigitalOut _cePin, _csnPin, _mosi, _sck; 00135 DigitalIn _miso, _irqPin; 00136 SPI _spi; 00137 00138 uint8_t _readReg(uint8_t addr); 00139 void _readRegMultiLSB(uint8_t addr, uint8_t *buf, size_t len); 00140 void _writeReg(uint8_t addr, uint8_t val); 00141 void _writeRegMultiLSB(uint8_t addr, uint8_t *buf, size_t len); 00142 void _issueCmd(uint8_t cmd); 00143 void _readCmdPayload(uint8_t addr, uint8_t *buf, size_t len, size_t maxlen); 00144 void _issueCmdPayload(uint8_t cmd, uint8_t *buf, size_t len); 00145 uint8_t _irq_getreason(); 00146 uint8_t _irq_derivereason(); // Get IRQ status from rf_status w/o querying module over SPI. 00147 void _irq_clear(uint8_t irq); 00148 boolean _isAlive(); 00149 void _readTXaddr(uint8_t *buf); 00150 void _writeRXaddrP0(uint8_t *buf); 00151 void _maintenanceHook(); // Handles IRQs and purges RX queue when erroneous contents exist. 00152 00153 /* Private planning: 00154 Need to keep track of: 00155 RF status (since we get it after every SPI communication, might as well store it) 00156 RF channel (to refresh in order to reset PLOS_CNT) 00157 RF speed (to determine if autoAck makes any sense, since it doesn't @ 250Kbps) 00158 Address Length (to determine how many bytes to read out of set(RX|TX)address()) 00159 32-byte TX ring buffer 00160 Ring buffer position 00161 */ 00162 }; 00163 00164 #endif
Generated on Fri Jul 15 2022 01:38:37 by
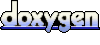