BERTL library, Kevin Heinrich
Dependents: BertlPingPong_UEbung3_EB
bertl14.cpp
00001 #include <mbed.h> 00002 #include "bertl14.h" 00003 //********************************************************************************* 00004 // Define Motor Routines 00005 DigitalOut engineLR(P1_0); //IN1, EP10, MG1A => MG1 engine-Pin 2, left_Reverse 00006 DigitalOut engineLF(P1_1); //IN2, EP11, MG1B => MG1 engine-Pin 1, left_Forward 00007 PwmOut engineLEN(p34); //EN1, P34, left_ENABLE 00008 DigitalOut engineRR(P1_4); //IN4, EP13, MG2A => MG2 engine-Pin 2, right_Reverse 00009 DigitalOut engineRF(P1_3); //IN3, EP14, MG2B => MG2 engine-Pin 1, right_Forward 00010 PwmOut engineREN(p36); //EN2, P36, right_ENABLE 00011 //********************************************************************************** 00012 // Define PC9555 Routines 00013 I2C pc9555(P0_5, P0_4);// SDA, SCL 00014 00015 //********************************************************************************** 00016 //Start PC9555 Routines 00017 void bertl_PC9555_init() 00018 { 00019 char data[2]; 00020 // I2C-Initialisierung 00021 pc9555.frequency(PC9555_FREQUENCY); 00022 // Port 0 = Leds, Port 1 = Switches 00023 // Adresse, R/W# = 0, Config PORT 0 (6) = 0x00(= Output), Stop 00024 data[0] = PC9555_PORT0_DIRCONFIG; 00025 data[1] = 0x00; 00026 pc9555.write(PC9555_ADDR_W, data, 2); 00027 // Adresse, R/W# = 0, Config PORT 1 (7) = 0xFF(= Input), Stop 00028 data[0] = PC9555_PORT1_DIRCONFIG; 00029 data[1] = 0xFF; 00030 pc9555.write(PC9555_ADDR_W, data, 2); 00031 } 00032 00033 void bertl_PC9555_leds(unsigned char leds) 00034 { 00035 char data[2]; //Two Bits for Transmitt via I2C 00036 // Send leds to PORT0 of PC9555 via I2C: 00037 // Start, Address, RW# = 0, CMD PC9555_PORT0_OUT, leds, STOP 00038 data[0] = PC9555_PORT0_OUT; 00039 data[1] = ~leds; //bitwise inversion since Hardware is switched on with 0 (inverse logic) 00040 pc9555.write(PC9555_ADDR_W, data, 2); 00041 } 00042 00043 unsigned char bertl_PC9555_switches() 00044 { 00045 char taster[1]; 00046 00047 pc9555.start(); // Start PC9555 to write the Adress 00048 pc9555.write(PC9555_ADDR_W); // Write the WRITE-Adress 00049 pc9555.write(0x01); // To define the Switches => 0x02: LEDs 00050 pc9555.start(); // Start PC9555 again to read the Values 00051 pc9555.write(PC9555_ADDR_R); // Set the READ_bit 00052 taster[0] = pc9555.read(0); // Read the Value of the Switches (HEX_MASK) 00053 pc9555.stop(); // Stop the I2C 00054 00055 return (taster[0]); // Return the value 00056 00057 } 00058 // END PC9555 Routines 00059 //********************************************************************************** 00060 //********************************************************************************** 00061 // Begin Motor Routines 00062 void bertl_engine(int left, int right) 00063 { 00064 // If the left engines-value is greater than 0, the left engine turn FORWARD 00065 if(left > 0) 00066 { 00067 engineLF=1; 00068 engineLR=0; 00069 } 00070 // Or if the left engines-value is less than 0, the left engine turn REVERSE 00071 else if(left < 0) 00072 { 00073 engineLF=0; 00074 engineLR=1; 00075 left = left * (-1); // For PWM the Value have to be positive 00076 } 00077 // If the right engines-value is greater than 0, the right engine turn FORWARD 00078 if(right > 0) 00079 { 00080 engineRF=1; 00081 engineRR=0; 00082 } 00083 // Or if the right engines-value is less than 0, the right engine turn REVERSE 00084 else if(right < 0) 00085 { 00086 engineRF=0; 00087 engineRR=1; 00088 right = right * (-1); // For PWM the Value have to be positive 00089 } 00090 //pc.printf("left: %4d\r\n", left); 00091 //pc.printf("right: %4d\r\n", right); 00092 // Or if the right- or/and the left engines value equals 0, the ENABLE-Pin turn off (ENABLE Pin equals 0) 00093 engineLEN=(left/255.0); // PWM Value : 0 ... 1 00094 engineREN=(right/255.0); // PWM Value : 0 ... 1 00095 } 00096 //Begin Testing bertl-engines 00097 void bertl_engine_test() 00098 { 00099 bertl_engine(100, 0); //The left engine must turn FORWARD for 2 seconds 00100 wait(2); 00101 bertl_engine(0, 100); //The right engine must turn FORWARD for 2 seconds 00102 wait(2); 00103 bertl_engine(-100, 0); //The left engine must turn REVERSE for 2 seconds 00104 wait(2); 00105 bertl_engine(0, -100); //The right engine must turn REVERSE for 2 seconds 00106 wait(2); 00107 } 00108 //End Testing bertl-engines 00109 // END Motor Routines
Generated on Thu Aug 18 2022 10:46:08 by
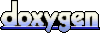