
Linking but does not give binary - Error L6320W
Dependencies: mbed wave_player SDFileSystem PinDetect
main.cpp
00001 #include "mbed.h" 00002 #include "SDFileSystem.h" 00003 #include "wave_player.h" 00004 #include "PinDetect.h" 00005 #include <vector> 00006 #include <string> 00007 00008 #define UP PC_13 00009 #define DOWN PB_1 00010 #define BACK PB_5 00011 #define MENU PB_2 00012 #define SELECT PB_9 00013 #define VOLUP PB_7 00014 #define VOLDOWN PB_6 00015 00016 #define LONG_PRESS_TIME 80 //Value * 20ms = debounce time 00017 #define SHORT_PRESS_TIME 5 //Value * 20ms = debounce time 00018 00019 using namespace std; 00020 00021 SDFileSystem sd(PB_15, PB_14, PB_13, PB_12, "TEST"); 00022 00023 PinDetect up(UP, PullUp); 00024 PinDetect down(DOWN, PullUp); 00025 PinDetect back(BACK, PullUp); 00026 PinDetect menu(MENU, PullUp); 00027 PinDetect select(SELECT, PullUp); 00028 PinDetect volup(VOLUP, PullUp); 00029 PinDetect voldown(VOLDOWN, PullUp); 00030 bool buttonStatus = 0; 00031 00032 AnalogOut DACout(PA_4); 00033 wave_player waver(&DACout); 00034 int pos = 0; // index of the song 00035 int vol = 0; // volume controller 00036 00037 bool playing = false; //variable for pause/play since we only have 1 pb for that 00038 vector<string> filenames; //filenames are stored in a vector string 00039 00040 void read_file_names(char *dir) // function that reads in file names from sd cards 00041 { 00042 DIR *dp; 00043 struct dirent *dirp; 00044 dp = opendir(dir); 00045 //read all directory and file names in current directory into filename vector 00046 while((dirp = readdir(dp)) != NULL) { 00047 filenames.push_back(string(dirp->d_name)); 00048 } 00049 } 00050 00051 void upPressedCallback(void){ 00052 //printf("\nUP Pressed\n"); 00053 00054 int l = filenames.size(); 00055 if (pos > 0) { 00056 pos--; 00057 } else if (pos == 0 ) { 00058 pos = l-1; 00059 } 00060 00061 } 00062 void upHeldCallback(void){ 00063 printf("\nUP Held\n"); 00064 } 00065 00066 void downPressedCallback(void){ 00067 //printf("\ndown Pressed\n"); 00068 // it checks for the total number of songs in the sd card..then increments the index until it reaches the last one, then resets to 0 00069 00070 int l = filenames.size(); 00071 if (pos < (l-1)) { 00072 pos++; 00073 } else if (pos == (l-1)) { 00074 pos = 0; 00075 } 00076 00077 } 00078 void downHeldCallback(void){ 00079 printf("\ndown Held\n"); 00080 } 00081 00082 void backPressedCallback(void){ 00083 printf("\nback Pressed\n"); 00084 } 00085 void backHeldCallback(void){ 00086 printf("\nback Held\n"); 00087 } 00088 00089 void menuPressedCallback(void){ 00090 //printf("\nmenu Pressed\n"); 00091 00092 //this interrupt handler changes the play to pause mode or vice versa 00093 //this is done using the boolean playing 00094 00095 if (playing == false) { 00096 playing = true; 00097 } else if (playing == true) { 00098 playing = false; 00099 } 00100 00101 } 00102 void menuHeldCallback(void){ 00103 //printf("\nmenu Held\n"); 00104 } 00105 00106 void volupPressedCallback(void){ 00107 printf("\nvolup Pressed\n"); 00108 } 00109 void volupHeldCallback(void){ 00110 printf("\nvolup Held\n"); 00111 } 00112 00113 void voldownPressedCallback(void){ 00114 //printf("\nvoldown Pressed\n"); 00115 // this pb changes the volume by lowering the volume until it reaches 0. then it resets to the max volume 00116 // the volume range has been divided into 16 possible ranges. and hence, it toggles through those 16 values 00117 // this only changes the variable vol, which is then used in the wave player file to actually adjust the volume 00118 vol = (vol+1) % 16; 00119 } 00120 void voldownHeldCallback(void){ 00121 printf("\nvoldown Held\n"); 00122 } 00123 00124 bool buttons_init(void) { 00125 up.attach_deasserted( &upPressedCallback ); 00126 //up.attach_deasserted( &upReleasedCallback ); 00127 up.attach_asserted_held( &upHeldCallback ); 00128 up.setSamplesTillAssert( SHORT_PRESS_TIME ); 00129 up.setSamplesTillHeld( LONG_PRESS_TIME ); 00130 up.setAssertValue( 0 ); 00131 up.setSampleFrequency(); 00132 //up.fall(callback(this, &upCallback)); 00133 00134 down.attach_deasserted( &downPressedCallback ); 00135 //down.attach_deasserted( &downReleasedCallback ); 00136 down.attach_asserted_held( &downHeldCallback ); 00137 down.setSamplesTillAssert( SHORT_PRESS_TIME ); 00138 down.setSamplesTillHeld( LONG_PRESS_TIME ); 00139 down.setAssertValue( 0 ); 00140 down.setSampleFrequency(); 00141 00142 back.attach_deasserted( &backPressedCallback ); 00143 //back.attach_deasserted( &backReleasedCallback ); 00144 back.attach_asserted_held( &backHeldCallback ); 00145 back.setSamplesTillAssert( SHORT_PRESS_TIME ); 00146 back.setSamplesTillHeld( LONG_PRESS_TIME ); 00147 back.setAssertValue( 0 ); 00148 back.setSampleFrequency(); 00149 00150 menu.attach_deasserted( &menuPressedCallback ); 00151 //menu.attach_deasserted( &menuReleasedCallback ); 00152 menu.attach_asserted_held( &menuHeldCallback ); 00153 menu.setSamplesTillAssert( SHORT_PRESS_TIME ); 00154 menu.setSamplesTillHeld( LONG_PRESS_TIME ); 00155 menu.setAssertValue( 0 ); 00156 menu.setSampleFrequency(); 00157 00158 volup.attach_deasserted( &volupPressedCallback ); 00159 //volup.attach_deasserted( &volupReleasedCallback ); 00160 volup.attach_asserted_held( &volupHeldCallback ); 00161 volup.setSamplesTillAssert( SHORT_PRESS_TIME ); 00162 volup.setSamplesTillHeld( LONG_PRESS_TIME ); 00163 volup.setAssertValue( 0 ); 00164 volup.setSampleFrequency(); 00165 00166 voldown.attach_deasserted( &voldownPressedCallback ); 00167 //voldown.attach_deasserted( &voldownReleasedCallback ); 00168 voldown.attach_asserted_held( &voldownHeldCallback ); 00169 voldown.setSamplesTillAssert( SHORT_PRESS_TIME ); 00170 voldown.setSamplesTillHeld( LONG_PRESS_TIME ); 00171 voldown.setAssertValue( 0 ); 00172 voldown.setSampleFrequency(); 00173 00174 /* 00175 down.fall(callback(&downCallback)); 00176 back.fall(callback(&backCallback)); 00177 menu.fall(callback(&menuCallback)); 00178 select.fall(callback(&selectCallback)); 00179 volup.fall(callback(&volupCallback)); 00180 voldown.fall(callback(&voldownCallback)); 00181 */ 00182 00183 printf("\nButtons Initialized\n"); 00184 return 1; 00185 } 00186 00187 int main() 00188 { 00189 printf("\n\n\nHello, wave world =======!\n"); 00190 buttonStatus = buttons_init(); 00191 read_file_names("/TEST/Music"); 00192 00193 /* 00194 //FILE *wave_file; 00195 //wave_file=fopen("/TEST/queen.wav","r"); 00196 //wave_player waver(&DACout, wave_file); 00197 //waver.play(); 00198 00199 wave_file=fopen("/TEST/queen.wav","r"); 00200 waver.play(); 00201 fclose(wave_file); 00202 */ 00203 00204 while(1) { 00205 //while pb3 is low, then we can start playing the song 00206 while(playing == true) { //we have 2 while loops..one while loop makes sure the music player is always on, the other one is for the song 00207 string songname = filenames[pos]; 00208 string a = "/TEST/"; 00209 string fname = a + songname; //retrieves the file name 00210 FILE *wave_file; 00211 wave_file = fopen(fname.c_str(),"r"); //opens the music file 00212 waver.play(wave_file); //plays the music file 00213 fclose(wave_file); 00214 } 00215 } 00216 00217 } 00218
Generated on Fri Jul 22 2022 14:41:29 by
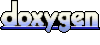