
hattori&ide
Embed:
(wiki syntax)
Show/hide line numbers
TextOLED.cpp
00001 /* mbed TextOLED Library, for a 4-bit LCD based on Winstar WEH000000 series 00002 * Originl: http://mbed.org/users/simon/libraries/TextLCD/latest 00003 * Modified by Suga 00004 */ 00005 /* mbed TextLCD Library, for a 4-bit LCD based on HD44780 00006 * Copyright (c) 2007-2010, sford, http://mbed.org 00007 * 00008 * Permission is hereby granted, free of charge, to any person obtaining a copy 00009 * of this software and associated documentation files (the "Software"), to deal 00010 * in the Software without restriction, including without limitation the rights 00011 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00012 * copies of the Software, and to permit persons to whom the Software is 00013 * furnished to do so, subject to the following conditions: 00014 * 00015 * The above copyright notice and this permission notice shall be included in 00016 * all copies or substantial portions of the Software. 00017 * 00018 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00019 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00020 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00021 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00022 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00023 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00024 * THE SOFTWARE. 00025 */ 00026 00027 #include "TextOLED.h" 00028 #include "mbed.h" 00029 00030 TextOLED::TextOLED(PinName rs, PinName e, PinName d4, PinName d5, 00031 PinName d6, PinName d7, LCDType type) : _rs(rs), 00032 _e(e), _d(d4, d5, d6, d7), 00033 _type(type) { 00034 int i; 00035 00036 _rs = 0; // command mode 00037 _e = 1; 00038 00039 wait_ms(100); // Wait 15ms to ensure powered up 00040 00041 for (i = 0; i < 5; i ++) { 00042 write4bit(0); 00043 } 00044 /* 00045 write4bit(0x3); // 8-bit mode 00046 write4bit(0x3); // 8-bit mode 00047 write4bit(0x0); 00048 */ 00049 write4bit(0x2); // 4-bit mode 00050 00051 writeCommand(0x28); // Function set 001 BW N F - - 00052 writeCommand(0x0C); // 0000 1 D C B 00053 writeCommand(0x06); // Entry mode : 0000 01 I/D S 00054 writeCommand(0x10); // Cursor and Display Shift 0001 S/C R/L 0 0 00055 writeCommand(0x17); // DCDC on 00056 wait_ms(10); 00057 writeCommand(0x02); // Home 00058 cls(); 00059 } 00060 00061 void TextOLED::character(int column, int row, int c) { 00062 int a = address(column, row); 00063 writeCommand(a); 00064 writeData(c); 00065 } 00066 00067 void TextOLED::cls() { 00068 writeCommand(0x01); // cls, and set cursor to 0 00069 wait_ms(10); // This command takes 1.64 ms 00070 locate(0, 0); 00071 } 00072 00073 void TextOLED::locate(int column, int row) { 00074 _column = column; 00075 _row = row; 00076 } 00077 00078 int TextOLED::_putc(int value) { 00079 if (value == '\n') { 00080 _column = 0; 00081 _row++; 00082 if (_row >= rows()) { 00083 _row = 0; 00084 } 00085 } else { 00086 character(_column, _row, value); 00087 _column++; 00088 if (_column >= columns()) { 00089 _column = 0; 00090 _row++; 00091 if (_row >= rows()) { 00092 _row = 0; 00093 } 00094 } 00095 } 00096 return value; 00097 } 00098 00099 int TextOLED::_getc() { 00100 return -1; 00101 } 00102 00103 void TextOLED::write4bit(int value) { 00104 _e = 1; 00105 _d = value; 00106 wait_us(1); 00107 _e = 0; 00108 wait_ms(10); 00109 } 00110 00111 void TextOLED::writeByte(int value) { 00112 _e = 1; 00113 _d = (value >> 4) & 0x0f; 00114 wait_us(1); 00115 _e = 0; 00116 wait_us(1); 00117 _e = 1; 00118 _d = value & 0x0f; 00119 wait_us(1); 00120 _e = 0; 00121 wait_us(50); 00122 } 00123 00124 void TextOLED::writeCommand(int command) { 00125 _rs = 0; 00126 wait_us(1); 00127 writeByte(command); 00128 } 00129 00130 void TextOLED::writeData(int data) { 00131 _rs = 1; 00132 wait_us(1); 00133 writeByte(data); 00134 } 00135 00136 int TextOLED::address(int column, int row) { 00137 switch (_type) { 00138 case LCD20x4: 00139 switch (row) { 00140 case 0: 00141 return 0x80 + column; 00142 case 1: 00143 return 0xc0 + column; 00144 case 2: 00145 return 0x94 + column; 00146 case 3: 00147 return 0xd4 + column; 00148 } 00149 case LCD16x2B: 00150 return 0x80 + (row * 40) + column; 00151 case LCD16x2: 00152 case LCD20x2: 00153 case LCD8x2: 00154 default: 00155 return 0x80 + (row * 0x40) + column; 00156 } 00157 } 00158 00159 int TextOLED::columns() { 00160 switch (_type) { 00161 case LCD8x2: 00162 return 8; 00163 case LCD20x4: 00164 case LCD20x2: 00165 return 20; 00166 case LCD16x2: 00167 case LCD16x2B: 00168 default: 00169 return 16; 00170 } 00171 } 00172 00173 int TextOLED::rows() { 00174 switch (_type) { 00175 case LCD20x4: 00176 return 4; 00177 case LCD16x2: 00178 case LCD16x2B: 00179 case LCD20x2: 00180 case LCD8x2: 00181 default: 00182 return 2; 00183 } 00184 } 00185 00186 void TextOLED::cursor (bool flg) { 00187 if (flg) { 00188 writeCommand(0x0F); // 0000 1 D C B 00189 } else { 00190 writeCommand(0x0C); // 0000 1 D C B 00191 } 00192 }
Generated on Sun Dec 18 2022 08:16:47 by
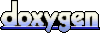