
hashem
Dependencies: mbed TextLCD keypad
main.cpp
00001 #include "mbed.h" 00002 #include "Keypad.h" 00003 #include "TextLCD.h" 00004 #include <string> 00005 00006 #define PW_SIZE 5 00007 #define PASSWORD "1234A" 00008 00009 Serial pc(USBTX, USBRX); 00010 00011 //initialize LCD display 00012 TextLCD lcd(p5, p7, p6, p11, p12, p13, p14, TextLCD::LCD16x2); // rs, rw, e, d0-d3 00013 00014 // Define keypad values 00015 char Keytable[] = { '1', '2', '3', 'A', // r0 00016 '4', '5', '6', 'B', // r1 00017 '7', '8', '9', 'C', // r2 00018 '*', '0', '#', 'D' // r3 00019 }; 00020 // c0 c1 c2 c3 00021 00022 //Initialize variables 00023 uint32_t Index; 00024 std::string pword; 00025 const char * parray; 00026 int psize=0; 00027 00028 uint32_t cbAfterInput(uint32_t index) { 00029 Index = index; 00030 00031 //print entered value on LCD and save password in string 00032 if(Keytable[Index] != '#' && Keytable[Index] != '*'){ 00033 if(psize < PW_SIZE){ 00034 pword.push_back(Keytable[Index]); 00035 lcd.putc(Keytable[Index]); 00036 parray = pword.c_str(); 00037 psize++; 00038 } 00039 } 00040 00041 if(Keytable[Index] == '*'){ 00042 pword = ""; 00043 lcd.cls(); 00044 wait(0.001); 00045 lcd.printf("Pass:"); 00046 lcd.locate(0,1); 00047 psize=0; 00048 } 00049 if(Keytable[Index] == '#'){ 00050 lcd.cls(); 00051 wait(0.001); 00052 if(pword == PASSWORD) 00053 lcd.printf("Correct"); 00054 //lcd.printf("pword"); 00055 //lcd.locate(0,1); 00056 //lcd.printf(parray); 00057 else 00058 lcd.printf("Incorrect"); 00059 } 00060 return 0; 00061 } 00062 00063 00064 int main() { 00065 00066 lcd.cls(); 00067 wait(0.001); 00068 lcd.printf("Pass:"); 00069 lcd.locate(0,1); 00070 00071 // r0 r1 r2 r3 c0 c1 c2 c3 00072 Keypad keypad(p28, p27, p26, p25, p21, p22, p23, p24); 00073 keypad.attach(&cbAfterInput); 00074 keypad.start(); // energize the keypad via c0-c3 00075 00076 while (1) { 00077 __wfi(); 00078 pc.printf("Interrupted\r\n"); 00079 pc.printf("Index:%d => Key:%c\r\n", Index, Keytable[Index]); 00080 } 00081 }
Generated on Fri Aug 26 2022 07:29:27 by
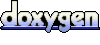