This is a class for using the DS1307 Real Time Clock chip from Dallas Semiconductor. This class uses mbeds i2c class to talk to the chip. I have tested this currently on the LPC1768 mbed device with the Spark fun breakout board from http://www.sparkfun.com/products/99
Dependents: Mbell Astromed_build20121123 Raymon_pub_ver CCRMonitor12_sp07_120ver ... more
DS1307 Class Reference
DS1307 control and communication class using mbed's i2c class. More...
#include <ds1307.h>
Public Member Functions | |
DS1307 (PinName sda, PinName slc) | |
Create object connected to DS1307 pins ( remember both pins need pull up resisters) | |
int | read (int addr, int quantity, char *data) |
Bulk read of several registers at a time. | |
int | read (int addr, int *data) |
Read one register of DS1307 device. | |
int | write (int addr, int quantity, char *data) |
Bulk write of several registers at a time. | |
int | write (int addr, int data) |
Write one register of DS1307 device. | |
int | start_clock (void) |
Start DS1307 clock. | |
int | stop_clock (void) |
Stop DS1307 clock. | |
int | twelve_hour (void) |
Set twelve hour mode on DS1307 (note this also converts 24 hour time to 12 time if needed on DS1307) | |
int | twentyfour_hour (void) |
Set twenty four hour mode on DS1307. | |
int | settime (int sec, int min, int hour, int day, int date, int month, int year) |
Set the time to some current or other value ( note that this will start the clock after it is set!) | |
int | gettime (int *sec, int *min, int *hour, int *day, int *date, int *month, int *year) |
Read the current time of the DS1307. |
Detailed Description
DS1307 control and communication class using mbed's i2c class.
Example:
// show how the DS1307 class works #include "ds1307.h" #include "mbed.h" Serial pc(USBTX, USBRX); // tx, rx for debug and usb pc comunications DS1307 my1307(p9,p10); // start DS1307 class and give it pins for connections of the DS1307 device int sec = 0; int min = 0; int hours = 0; int day = 0; int date = 0; int month = 0; int year = 0; void test_rw(int test) { if (test == 0) pc.printf("Last R/W operaion passed!\n\r"); else pc.printf("Last R/W operation failed!\n\r"); } int main() { int junk = 0; sec = 24; // 24 seconds min = 13; // 13 min hours = 13; // 1 pm day = 4; // wednesday date = 20; // June 20 month = 6; year = 12; // 2012 // set time to these values on the ds1307 connected device test_rw(my1307.settime( sec, min, hours, day, date, month, year)); pc.printf("seconds set are %.2D \n\r",sec); pc.printf("min set are %.2D \n\r",min); pc.printf("hour set are %.2D \n\r",hours); pc.printf("day set are %.2D \n\r",day); pc.printf("date set are %.2D \n\r",date); pc.printf("month set are %.2D \n\r",month); pc.printf("year set are %.2D \n\r",year); wait(3); // now read the time of the DS1307 device and see what time it is // note that because of the 3 second wait this time should be 3 seconds past what it was set to earlier test_rw(my1307.gettime( &sec, &min, &hours, &day, &date, &month, &year)); pc.printf("seconds read are %.2D \n\r",sec); pc.printf("min read are %.2D \n\r",min); pc.printf("hour read are %.2D \n\r",hours); pc.printf("day read are %.2D \n\r",day); pc.printf("date read are %.2D \n\r",date); pc.printf("month read are %.2D \n\r",month); pc.printf("year read are %.2D \n\r",year); junk = 0x39; // just a junk value do read and write test to DS1307 ram test_rw(my1307.write( 0x20, junk)); // this should write the value of junk to register 0x20 (a ram location) in the ds1307. pc.printf("Value written to register 0x20 %.2X \n\r",junk); junk = 0; // clear junk to show that when the register is read from the correct value is obtained test_rw(my1307.read( 0x20, &junk)); // this should read register 0x20 pc.printf("Value read from register 0x20 %.2X \n\r",junk); }
Definition at line 109 of file ds1307.h.
Constructor & Destructor Documentation
DS1307 | ( | PinName | sda, |
PinName | slc | ||
) |
Create object connected to DS1307 pins ( remember both pins need pull up resisters)
Ensure the pull up resistors are used on these pins. Also note there is no checking on if you use thes pins p9, p10, p27, p28 so ensure you only use these ones on the LPC1768 device
- Parameters:
-
sda pin that DS1307 connected to (p9 or p28 as defined on LPC1768) slc pin that DS1307 connected to (p10 or p27 ad defined on LPC1768)
Definition at line 3 of file ds1307.cpp.
Member Function Documentation
int gettime | ( | int * | sec, |
int * | min, | ||
int * | hour, | ||
int * | day, | ||
int * | date, | ||
int * | month, | ||
int * | year | ||
) |
Read the current time of the DS1307.
- Parameters:
-
sec the seconds value (0 - 59) min the minute value (0 - 59) hour the hour value (0 - 23) always in 24 hour day the day value ( sunday is 1 ) date the date value (1 - 31) month the month value (1-12) year the year value (00 - 99) this is for 2000 to 2099 only as i understand it! returns 0 if time is read correctly 1 if the time was not recieved correctly for some reason
Definition at line 217 of file ds1307.cpp.
int read | ( | int | addr, |
int | quantity, | ||
char * | data | ||
) |
Bulk read of several registers at a time.
Ensure the variable data pointer passed to this function has the room needed to recieve the quantity!
- Parameters:
-
addr the address to read from quantity the amount of registers to read from data the place to put the values read returns 0 if read worked 1 if the read of DS1307 failed for some reason
Definition at line 10 of file ds1307.cpp.
int read | ( | int | addr, |
int * | data | ||
) |
Read one register of DS1307 device.
- Parameters:
-
addr the address to read from data read from the one register returns 0 if read worked 1 if the read of DS1307 failed for some reason
Definition at line 34 of file ds1307.cpp.
int settime | ( | int | sec, |
int | min, | ||
int | hour, | ||
int | day, | ||
int | date, | ||
int | month, | ||
int | year | ||
) |
Set the time to some current or other value ( note that this will start the clock after it is set!)
Note this will return 1 if any of the values passed to this function are not as listed below!
- Parameters:
-
sec the seconds value (0 - 59) min the minute value (0 - 59) hour the hour value (0 - 23) always in 24 hour day the day value ( sunday is 1 ) date the date value (1 - 31) month the month value (1-12) year the year value (00 - 99) this is for 2000 to 2099 only as i understand it! returns 0 if time is set 1 if the time setting failed in some way
Definition at line 165 of file ds1307.cpp.
int start_clock | ( | void | ) |
Start DS1307 clock.
- Parameters:
-
returns 0 if clock started 1 if the write command to DS1307 failed for some reason
Definition at line 81 of file ds1307.cpp.
int stop_clock | ( | void | ) |
Stop DS1307 clock.
- Parameters:
-
returns 0 if clock stopped 1 if the write command to DS1307 failed for some reason
Definition at line 93 of file ds1307.cpp.
int twelve_hour | ( | void | ) |
Set twelve hour mode on DS1307 (note this also converts 24 hour time to 12 time if needed on DS1307)
Note this will convert DS1307 time values in registers to 12 hour values from 24 hour values if needed
- Parameters:
-
returns 0 if DS1307 is now in 12 hour mode 1 if the command to DS1307 failed for some reason
Definition at line 106 of file ds1307.cpp.
int twentyfour_hour | ( | void | ) |
int write | ( | int | addr, |
int | quantity, | ||
char * | data | ||
) |
Bulk write of several registers at a time.
- Parameters:
-
addr the address to write to quantity the amount of registers to write to data that contains the values to be written to the registers returns 0 if write worked 1 if the write to DS1307 failed for some reason
Definition at line 44 of file ds1307.cpp.
int write | ( | int | addr, |
int | data | ||
) |
Write one register of DS1307 device.
- Parameters:
-
addr the address to write to data to write to register returns 0 if write worked 1 if the write to DS1307 failed for some reason
Definition at line 67 of file ds1307.cpp.
Generated on Wed Jul 13 2022 15:31:01 by
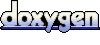