Circular Buffer template for any data type
Dependents: serqet serqet2 EMGvoorjan kopija_NUCLEO_CELL_LOCKER_copy ... more
circular_buffer.cpp
00001 00002 #ifndef CIRCULAR_BUFFER_CPP 00003 #define CIRCULAR_BUFFER_CPP 00004 00005 #include <stdio.h> 00006 #include "circular_buffer.h" 00007 00008 /* 00009 template<class T> 00010 circular_buffer<T>::circular_buffer() 00011 { 00012 capacity = 0; 00013 size = 0; 00014 00015 buffer = 0; 00016 00017 reset(); 00018 } 00019 */ 00020 00021 template<class T> 00022 circular_buffer<T>::circular_buffer(int tcapacity) 00023 { 00024 capacity = tcapacity; 00025 buffer = new T[capacity]; 00026 00027 reset(); 00028 } 00029 00030 template<class T> 00031 circular_buffer<T>::~circular_buffer() 00032 { 00033 if (buffer != 0) 00034 delete[] buffer; 00035 } 00036 00037 template<class T> 00038 void circular_buffer<T>::reset() 00039 { 00040 start_pos = 0; 00041 end_pos = 0; 00042 size = 0; 00043 } 00044 00045 template<class T> 00046 int circular_buffer<T>::get_capacity() 00047 { 00048 return capacity; 00049 } 00050 00051 template<class T> 00052 int circular_buffer<T>::get_size() 00053 { 00054 return size; 00055 } 00056 00057 template<class T> 00058 void circular_buffer<T>::push_back(T item) 00059 { 00060 if (size == capacity) 00061 pop_front(); 00062 00063 buffer[end_pos] = item; 00064 increment(end_pos); 00065 size++; 00066 } 00067 00068 /* 00069 template<class T> 00070 void circular_buffer<T>::push_front(T item) 00071 { 00072 if (size == capacity) 00073 pop_back(); 00074 00075 buffer[start_pos] = item; 00076 decrement(start_pos); 00077 size++; 00078 } 00079 00080 */ 00081 00082 template<class T> 00083 void circular_buffer<T>::pop_back() 00084 { 00085 if (size != 0) 00086 { 00087 size--; 00088 decrement(end_pos); 00089 } 00090 } 00091 00092 template<class T> 00093 void circular_buffer<T>::pop_front() 00094 { 00095 if (size != 0) 00096 { 00097 size--; 00098 increment(start_pos); 00099 } 00100 } 00101 00102 template<class T> 00103 void circular_buffer<T>::increment(int& index) 00104 { 00105 index++; 00106 if (index >= capacity) 00107 index = 0; 00108 } 00109 00110 template<class T> 00111 void circular_buffer<T>::decrement(int& index) 00112 { 00113 index--; 00114 if (index < 0) 00115 index = capacity - 1; 00116 } 00117 00118 00119 template<class T> 00120 int circular_buffer<T>::if_increment(int index) 00121 { 00122 index++; 00123 if (index >= capacity) 00124 index = 0; 00125 00126 return index; 00127 } 00128 00129 template<class T> 00130 int circular_buffer<T>::if_decrement(int index) 00131 { 00132 index--; 00133 if (index < capacity) 00134 index = capacity - 1; 00135 00136 return index; 00137 } 00138 00139 template<class T> 00140 T& circular_buffer<T>::front() 00141 { 00142 return buffer[start_pos]; 00143 } 00144 00145 template<class T> 00146 T& circular_buffer<T>::back() 00147 { 00148 return buffer[if_decrement(end_pos)]; 00149 } 00150 00151 00152 template<class T> 00153 T& circular_buffer<T>::operator[](int index) 00154 { 00155 int real_index = 0; 00156 00157 // if (size == 0) // no item 00158 // return NULL; 00159 00160 real_index = index + start_pos; 00161 if (real_index >= capacity) 00162 real_index -= capacity; 00163 00164 return buffer[real_index]; 00165 } 00166 00167 00168 template<class T> 00169 T& circular_buffer<T>::at(int index) 00170 { 00171 int real_index = 0; 00172 00173 real_index = index + start_pos; 00174 if (real_index >= capacity) 00175 real_index -= capacity; 00176 00177 return buffer[real_index]; 00178 } 00179 00180 #endif // CIRCULAR_BUFFER_CPP
Generated on Sat Jul 23 2022 11:54:41 by
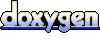