
This demonstrates some of the capabilities of the MAX44000. It is written to work with the ARD2PMD adapter board and the MAX44000PMB1. It uses the standard Arduino pin names and will compile for most Arduino form-factor mbed boards.
main.cpp
00001 /* MAX44000 Ambient Light Sensor / Proximity Sensor Demo 00002 * This demonstrates some of the capabilities of the MAX44000. 00003 * 00004 * This is written to work with the ARD2PMD adapter board and 00005 * the MAX44000PMB1. It uses the standard Arduino pin names 00006 * and it will compile for most arduino form-factor mbed boards. 00007 * 00008 * LED1 toggles when something is first detected by the proximity sensor. 00009 * LED2 indicates when the Ambient Light Sensor reads grater than alsLim. 00010 * LED3 will stay on whenever something is detected by the proximity sensor 00011 * and stay on for an additional 5s after it is no longer detected. 00012 * 00013 * The following boards have been tested to work: 00014 * MAX32600MBED 00015 * FRDM-KL25Z 00016 * FRDM-K64F 00017 * Seeeduino Arch (LEDs inverted) 00018 * Seeeduino Arch Pro 00019 * ST Nucleo F401RE (only one LED, inverted) 00020 * 00021 * Some boards use D13 for an LED signal, so this example uses the second 00022 * row (row B, pins 7 through 12) of the Pmod connector. 00023 */ 00024 00025 #include "mbed.h" 00026 #include "ard2pmod.h" 00027 00028 I2C i2c(D14, D15); 00029 DigitalOut tog(LED1); 00030 DigitalOut als(LED2); 00031 DigitalOut dly(LED3); 00032 DigitalIn pb3(D6); // Set as input to remove load from PB3 00033 DigitalIn pb4(D7); // Set as input to remove load from PB4 00034 00035 const int alsAddr = 0x94; 00036 const int alsLim = 0x0008; 00037 00038 int main() 00039 { 00040 char cmd[2]; 00041 int alsData; 00042 00043 Ard2Pmod ard2pmd(Ard2Pmod::PMOD_TYPE_I2C_B); // Configure Ard2Pmod board for I2C on row B 00044 00045 cmd[0] = 0x02; // Receive Register 00046 cmd[1] = 0xFC; // 1.56ms, 1x 00047 i2c.write(alsAddr, cmd, 2); 00048 00049 cmd[0] = 0x03; // Transmit Register 00050 cmd[1] = 0x0F; // 110mA 00051 i2c.write(alsAddr, cmd, 2); 00052 00053 cmd[0] = 0x01; // Config Register 00054 cmd[1] = 0x10; // ALS/Prox Mode 00055 i2c.write(alsAddr, cmd, 2); 00056 00057 bool lastProx = false; 00058 int offDelay = 0; 00059 tog = false; 00060 als = false; 00061 dly = false; 00062 00063 while (true) { 00064 wait (0.02); 00065 cmd[0] = 0x05; // ALS Data Register Low 00066 i2c.write(alsAddr, cmd, 1, true); 00067 i2c.read(alsAddr, cmd, 1); 00068 alsData = cmd[0]; 00069 als = (alsData < alsLim); 00070 00071 cmd[0] = 0x16; // Prox Data Register 00072 i2c.write(alsAddr, cmd, 1, true); 00073 i2c.read(alsAddr, cmd, 1); 00074 if (cmd[0]) { 00075 if (!lastProx) { 00076 tog = !tog; 00077 } 00078 lastProx = true; 00079 offDelay = 250; 00080 dly = false; 00081 } else { 00082 lastProx = false; 00083 } 00084 if (offDelay > 0) { offDelay -= 1; } 00085 else { dly = true; } 00086 } 00087 }
Generated on Mon Jul 18 2022 09:57:07 by
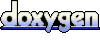