
HTTP server for GSwifi see: http://mbed.org/users/gsfan/notebook/gainspan_wifi/
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 00002 * HTTP server for GSwifi 00003 * 00004 * create the index.htm for LocalFileSystem. 00005 * html access http://IP address/ , http://IP address/test/ 00006 * 00007 * CGI access http://IP address/cgi-bin/hoge?hage 00008 */ 00009 00010 #include "mbed.h" 00011 #include "GSwifi.h" 00012 00013 #define PORT 80 00014 00015 #define SECURE GSwifi::GSSEC_WPA_PSK 00016 #define SSID "SSID" 00017 #define PASS "PASSPHRASE" 00018 00019 GSwifi gs(p13, p14, p20); // TX, RX, Reset (no flow control) 00020 //GSwifi gs(p13, p14, p12, P0_22, p20, NC, 115200); // TX, RX, CTS, RTS, Reset, Alarm 00021 00022 LocalFileSystem local("local"); 00023 Serial pc(USBTX, USBRX); 00024 DigitalOut led1(LED1), led2(LED2); 00025 00026 00027 void cgi (int cid, GSwifi::GS_httpd *gshttpd) { 00028 int i; 00029 00030 led2 = 1; 00031 pc.printf("CGI %d: %s ? %s '%s' %d\r\n", cid, gshttpd->file, gshttpd->query, gshttpd->buf, gshttpd->len); 00032 00033 gs.send(cid, "HTTP/1.1 200 OK\r\n", 17); 00034 gs.send(cid, "Content-type: text/plain\r\n", 26); 00035 gs.send(cid, "\r\n", 2); 00036 00037 gs.send(cid, "REQUEST_METHOD: ", 16); 00038 if (gshttpd[cid].type == GSwifi::GSPROT_HTTPGET) { 00039 gs.send(cid, "GET\r\n", 5); 00040 } else { 00041 gs.send(cid, "POST\r\n", 6); 00042 } 00043 gs.send(cid, "SCRIPT_NAME: ", 13); 00044 gs.send(cid, gshttpd->file, strlen(gshttpd->file)); 00045 gs.send(cid, "\r\n", 2); 00046 gs.send(cid, "QUERY_STRING: ", 14); 00047 gs.send(cid, gshttpd->query, strlen(gshttpd->query)); 00048 gs.send(cid, "\r\n", 2); 00049 gs.send(cid, "POST_BODY: ", 11); 00050 gs.send(cid, gshttpd->buf, strlen(gshttpd->buf)); 00051 gs.send(cid, "\r\n", 2); 00052 00053 } 00054 00055 int main () { 00056 IpAddr ipaddr, netmask, gateway, nameserver; 00057 00058 led1 = 1; 00059 pc.baud(115200); 00060 00061 pc.printf("connecting...\r\n"); 00062 if (gs.connect(SECURE, SSID, PASS)) { 00063 return -1; 00064 } 00065 gs.getAddress(ipaddr, netmask, gateway, nameserver); 00066 pc.printf("ip %d.%d.%d.%d\r\n", ipaddr[0], ipaddr[1], ipaddr[2], ipaddr[3]); 00067 00068 led2 = 1; 00069 pc.printf("httpd\r\n"); 00070 gs.httpd(PORT); 00071 gs.attach_httpd("/test/", "/local/"); 00072 gs.attach_httpd("/cgi-bin/", &cgi); 00073 gs.attach_httpd("/", "/local/"); 00074 00075 for (;;) { 00076 gs.poll(); 00077 00078 wait_ms(50); 00079 led1 = !led1; 00080 led2 = 0; 00081 } 00082 }
Generated on Sun Jul 31 2022 10:59:03 by
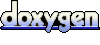