
X-CUBE-SPN1-20150128 example source code for one motor compiled under mbed. Tested OK on Nucleo F401. l6474.cpp is modified from original with defines in l6474_target_config.h to select the original behaviour (motor de-energised when halted), or new mode to continue powering with a (reduced) current in the coils (braking/position hold capability). On F401 avoid using mbed's InterruptIn on pins 10-15 (any port). Beware of other conflicts! L0 & F0 are included but untested.
stm32l0xx_nucleo_ihm01a1.h
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l0xx_nucleo_ihm01a1.h 00004 * @author IPC Rennes 00005 * @version V1.5.0 00006 * @date November 12, 2014 00007 * @brief Header for BSP driver for x-nucleo-ihm01a1 Nucleo extension board 00008 * (based on L6474) 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2014 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Define to prevent recursive inclusion -------------------------------------*/ 00040 #ifndef __STM32L0XX_NUCLEO_IHM01A1_H 00041 #define __STM32L0XX_NUCLEO_IHM01A1_H 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 /* Includes ------------------------------------------------------------------*/ 00048 //#include "stm32l0xx_nucleo.h" 00049 00050 /** @addtogroup BSP 00051 * @{ 00052 */ 00053 00054 /** @addtogroup STM32L0XX_NUCLEO_IHM01A1 00055 * @{ 00056 */ 00057 00058 /** @defgroup IHM01A1_Board_Private_Function_Prototypes 00059 * @{ 00060 */ 00061 00062 void BSP_MotorControlBoard_Delay(uint32_t delay); //Delay of the requested number of milliseconds 00063 void BSP_MotorControlBoard_DisableIrq(void); //Disable Irq 00064 void BSP_MotorControlBoard_EnableIrq(void); //Enable Irq 00065 void BSP_MotorControlBoard_GpioInit(uint8_t nbDevices); //Initialise GPIOs used for L6474s 00066 void BSP_MotorControlBoard_Pwm1SetFreq(uint16_t newFreq); //Set PWM1 frequency and start it 00067 void BSP_MotorControlBoard_Pwm2SetFreq(uint16_t newFreq); //Set PWM2 frequency and start it 00068 void BSP_MotorControlBoard_Pwm3SetFreq(uint16_t newFreq); //Set PWM3 frequency and start it 00069 void BSP_MotorControlBoard_PwmInit(uint8_t deviceId); //Init the PWM of the specified device 00070 void BSP_MotorControlBoard_PwmStop(uint8_t deviceId); //Stop the PWM of the specified device 00071 void BSP_MotorControlBoard_ReleaseReset(void); //Reset the L6474 reset pin 00072 void BSP_MotorControlBoard_Reset(void); //Set the L6474 reset pin 00073 void BSP_MotorControlBoard_SetDirectionGpio(uint8_t deviceId, uint8_t gpioState); //Set direction GPIO 00074 uint8_t BSP_MotorControlBoard_SpiInit(void); //Initialise the SPI used for L6474s 00075 uint8_t BSP_MotorControlBoard_SpiWriteBytes(uint8_t *pByteToTransmit, uint8_t *pReceivedByte, uint8_t nbDevices); //Write bytes to the L6474s via SPI 00076 00077 00078 /* Exported Constants --------------------------------------------------------*/ 00079 00080 /** @defgroup IHM01A1_Exported_Constants 00081 * @{ 00082 */ 00083 00084 /******************************************************************************/ 00085 /* USE_STM32L0XX_NUCLEO */ 00086 /******************************************************************************/ 00087 00088 /** @defgroup Constants_For_STM32L0XX_NUCLEO 00089 * @{ 00090 */ 00091 /// Interrupt line used for L6474 FLAG 00092 #define EXTI_MCU_LINE_IRQn (EXTI4_15_IRQn) 00093 00094 /// Timer used for PWM1 00095 #define BSP_MOTOR_CONTROL_BOARD_TIMER_PWM1 (TIM22) 00096 00097 /// Timer used for PWM2 00098 #define BSP_MOTOR_CONTROL_BOARD_TIMER_PWM2 (TIM2) 00099 00100 /// Timer used for PWM3 00101 #define BSP_MOTOR_CONTROL_BOARD_TIMER_PWM3 (TIM21) 00102 00103 /// Channel Timer used for PWM1 00104 #define BSP_MOTOR_CONTROL_BOARD_CHAN_TIMER_PWM1 (TIM_CHANNEL_2) 00105 00106 /// Channel Timer used for PWM2 00107 #define BSP_MOTOR_CONTROL_BOARD_CHAN_TIMER_PWM2 (TIM_CHANNEL_2) 00108 00109 /// Channel Timer used for PWM3 00110 #define BSP_MOTOR_CONTROL_BOARD_CHAN_TIMER_PWM3 (TIM_CHANNEL_1) 00111 00112 /// HAL Active Channel Timer used for PWM1 00113 #define BSP_MOTOR_CONTROL_BOARD_HAL_ACT_CHAN_TIMER_PWM1 (HAL_TIM_ACTIVE_CHANNEL_2) 00114 00115 /// HAL Active Channel Timer used for PWM2 00116 #define BSP_MOTOR_CONTROL_BOARD_HAL_ACT_CHAN_TIMER_PWM2 (HAL_TIM_ACTIVE_CHANNEL_2) 00117 00118 /// HAL Active Channel Timer used for PWM3 00119 #define BSP_MOTOR_CONTROL_BOARD_HAL_ACT_CHAN_TIMER_PWM3 (HAL_TIM_ACTIVE_CHANNEL_1) 00120 00121 /// Timer Clock Enable for PWM1 00122 #define __BSP_MOTOR_CONTROL_BOARD_TIMER_PWM1_CLCK_ENABLE() __TIM22_CLK_ENABLE() 00123 00124 /// Timer Clock Enable for PWM2 00125 #define __BSP_MOTOR_CONTROL_BOARD_TIMER_PWM2_CLCK_ENABLE() __TIM2_CLK_ENABLE() 00126 00127 /// Timer Clock Enable for PWM1 00128 #define __BSP_MOTOR_CONTROL_BOARD_TIMER_PWM3_CLCK_ENABLE() __TIM21_CLK_ENABLE() 00129 00130 /// Timer Clock Disable for PWM1 00131 #define __BSP_MOTOR_CONTROL_BOARD_TIMER_PWM1_CLCK_DISABLE() __TIM22_CLK_DISABLE() 00132 00133 /// Timer Clock Disable for PWM2 00134 #define __BSP_MOTOR_CONTROL_BOARD_TIMER_PWM2_CLCK_DISABLE() __TIM2_CLK_DISABLE() 00135 00136 /// Timer Clock Disable for PWM3 00137 #define __BSP_MOTOR_CONTROL_BOARD_TIMER_PWM3_CLCK_DISABLE() __TIM21_CLK_DISABLE() 00138 00139 /// PWM1 global interrupt 00140 #define BSP_MOTOR_CONTROL_BOARD_PWM1_IRQn (TIM22_IRQn) 00141 00142 /// PWM2 global interrupt 00143 #define BSP_MOTOR_CONTROL_BOARD_PWM2_IRQn (TIM2_IRQn) 00144 00145 /// PWM3 global interrupt 00146 #define BSP_MOTOR_CONTROL_BOARD_PWM3_IRQn (TIM21_IRQn) 00147 00148 /// PWM1 GPIO alternate function 00149 #define BSP_MOTOR_CONTROL_BOARD_AFx_TIMx_PWM1 (GPIO_AF0_TIM22) 00150 00151 /// PWM2 GPIO alternate function 00152 #define BSP_MOTOR_CONTROL_BOARD_AFx_TIMx_PWM2 (GPIO_AF2_TIM2) 00153 00154 #ifndef BSP_MOTOR_CONTROL_BOARD_USE_SPI2 00155 /// SPI SCK AF 00156 #define SPIx_SCK_AF (GPIO_AF0_SPI1) 00157 #else /* #ifndef BSP_MOTOR_CONTROL_BOARD_USE_SPI2 */ 00158 /// SPI SCK AF 00159 #define SPIx_SCK_AF (GPIO_AF0_SPI2) 00160 #endif /* #ifndef BSP_MOTOR_CONTROL_BOARD_USE_SPI2 */ 00161 00162 /// PWM1 frequency rescaler (1 for HW PWM, 2 for SW PWM) 00163 #define BSP_MOTOR_CONTROL_BOARD_PWM1_FREQ_RESCALER (1) 00164 /// PWM2 frequency rescaler (1 for HW PWM, 2 for SW PWM) 00165 #define BSP_MOTOR_CONTROL_BOARD_PWM2_FREQ_RESCALER (1) 00166 /// PWM3 frequency rescaler (1 for HW PWM, 2 for SW PWM) 00167 #define BSP_MOTOR_CONTROL_BOARD_PWM3_FREQ_RESCALER (2) 00168 00169 /** 00170 * @} 00171 */ 00172 00173 /******************************************************************************/ 00174 /* Independent plateform definitions */ 00175 /******************************************************************************/ 00176 00177 /** @defgroup Constants_For_All_Nucleo_Platforms 00178 * @{ 00179 */ 00180 00181 /// GPIO Pin used for the L6474 flag pin 00182 #define BSP_MOTOR_CONTROL_BOARD_FLAG_PIN (GPIO_PIN_10) 00183 /// GPIO port used for the L6474 flag pin 00184 #define BSP_MOTOR_CONTROL_BOARD_FLAG_PORT (GPIOA) 00185 00186 /// GPIO Pin used for the L6474 step clock pin of device 0 00187 #define BSP_MOTOR_CONTROL_BOARD_PWM_1_PIN (GPIO_PIN_7) 00188 /// GPIO Port used for the L6474 step clock pin of device 0 00189 #define BSP_MOTOR_CONTROL_BOARD_PWM_1_PORT (GPIOC) 00190 00191 /// GPIO Pin used for the L6474 step clock pin of device 1 00192 #define BSP_MOTOR_CONTROL_BOARD_PWM_2_PIN (GPIO_PIN_3) 00193 /// GPIO port used for the L6474 step clock pin of device 1 00194 #define BSP_MOTOR_CONTROL_BOARD_PWM_2_PORT (GPIOB) 00195 00196 /// GPIO Pin used for the L6474 step clock pin of device 2 00197 #define BSP_MOTOR_CONTROL_BOARD_PWM_3_PIN (GPIO_PIN_10) 00198 /// GPIO port used for the L6474 step clock pin of device 2 00199 #define BSP_MOTOR_CONTROL_BOARD_PWM_3_PORT (GPIOB) 00200 00201 /// GPIO Pin used for the L6474 direction pin of device 0 00202 #define BSP_MOTOR_CONTROL_BOARD_DIR_1_PIN (GPIO_PIN_8) 00203 /// GPIO port used for the L6474 direction pin of device 0 00204 #define BSP_MOTOR_CONTROL_BOARD_DIR_1_PORT (GPIOA) 00205 00206 /// GPIO Pin used for the L6474 direction pin of device 1 00207 #define BSP_MOTOR_CONTROL_BOARD_DIR_2_PIN (GPIO_PIN_5) 00208 /// GPIO port used for the L6474 direction pin of device 1 00209 #define BSP_MOTOR_CONTROL_BOARD_DIR_2_PORT (GPIOB) 00210 00211 /// GPIO Pin used for the L6474 direction pin of device 2 00212 #define BSP_MOTOR_CONTROL_BOARD_DIR_3_PIN (GPIO_PIN_4) 00213 /// GPIO port used for the L6474 direction pin of device 2 00214 #define BSP_MOTOR_CONTROL_BOARD_DIR_3_PORT (GPIOB) 00215 00216 /// GPIO Pin used for the L6474 reset pin 00217 #define BSP_MOTOR_CONTROL_BOARD_RESET_PIN (GPIO_PIN_9) 00218 /// GPIO port used for the L6474 reset pin 00219 #define BSP_MOTOR_CONTROL_BOARD_RESET_PORT (GPIOA) 00220 00221 /// GPIO Pin used for the L6474 SPI chip select pin 00222 #define BSP_MOTOR_CONTROL_BOARD_CS_PIN (GPIO_PIN_6) 00223 /// GPIO port used for the L6474 SPI chip select pin 00224 #define BSP_MOTOR_CONTROL_BOARD_CS_PORT (GPIOB) 00225 00226 /* Definition for SPIx clock resources */ 00227 00228 #ifndef BSP_MOTOR_CONTROL_BOARD_USE_SPI2 00229 /* Default SPI is SPI1 */ 00230 00231 /// Used SPI 00232 #define SPIx (SPI1) 00233 00234 /// SPI clock enable 00235 #define SPIx_CLK_ENABLE() __SPI1_CLK_ENABLE() 00236 00237 /// SPI SCK enable 00238 #define SPIx_SCK_GPIO_CLK_ENABLE() __GPIOA_CLK_ENABLE() 00239 00240 /// SPI MISO enable 00241 #define SPIx_MISO_GPIO_CLK_ENABLE() __GPIOA_CLK_ENABLE() 00242 00243 /// SPI MOSI enable 00244 #define SPIx_MOSI_GPIO_CLK_ENABLE() __GPIOA_CLK_ENABLE() 00245 00246 /// SPI Force reset 00247 #define SPIx_FORCE_RESET() __SPI1_FORCE_RESET() 00248 00249 /// SPI Release reset 00250 #define SPIx_RELEASE_RESET() __SPI1_RELEASE_RESET() 00251 00252 /// SPI SCK pin 00253 #define SPIx_SCK_PIN (GPIO_PIN_5) 00254 00255 /// SPI SCK port 00256 #define SPIx_SCK_GPIO_PORT (GPIOA) 00257 00258 00259 /// SPI MISO pin 00260 #define SPIx_MISO_PIN (GPIO_PIN_6) 00261 00262 /// SPI MISO port 00263 #define SPIx_MISO_GPIO_PORT (GPIOA) 00264 00265 /// SPI MOSI pin 00266 #define SPIx_MOSI_PIN (GPIO_PIN_7) 00267 00268 /// SPI MOSI port 00269 #define SPIx_MOSI_GPIO_PORT (GPIOA) 00270 00271 #else /* USE SPI2 */ 00272 00273 /// Used SPI 00274 #define SPIx (SPI2) 00275 00276 /// SPI clock enable 00277 #define SPIx_CLK_ENABLE() __SPI2_CLK_ENABLE() 00278 00279 /// SPI SCK enable 00280 #define SPIx_SCK_GPIO_CLK_ENABLE() __GPIOB_CLK_ENABLE() 00281 00282 /// SPI MISO enable 00283 #define SPIx_MISO_GPIO_CLK_ENABLE() __GPIOB_CLK_ENABLE() 00284 00285 /// SPI MOSI enable 00286 #define SPIx_MOSI_GPIO_CLK_ENABLE() __GPIOB_CLK_ENABLE() 00287 00288 /// SPI Force reset 00289 #define SPIx_FORCE_RESET() __SPI2_FORCE_RESET() 00290 00291 /// SPI Release reset 00292 #define SPIx_RELEASE_RESET() __SPI2_RELEASE_RESET() 00293 00294 /// SPI SCK pin 00295 #define SPIx_SCK_PIN (GPIO_PIN_13) 00296 00297 /// SPI SCK port 00298 #define SPIx_SCK_GPIO_PORT (GPIOB) 00299 00300 /// SPI MISO pin 00301 #define SPIx_MISO_PIN (GPIO_PIN_14) 00302 00303 /// SPI MISO port 00304 #define SPIx_MISO_GPIO_PORT (GPIOB) 00305 00306 /// SPI MISO AF 00307 #define SPIx_MISO_AF (SPIx_SCK_AF) 00308 00309 /// SPI MOSI pin 00310 #define SPIx_MOSI_PIN (GPIO_PIN_15) 00311 00312 /// SPI MOSI port 00313 #define SPIx_MOSI_GPIO_PORT (GPIOB) 00314 00315 #endif 00316 00317 /// SPI MISO AF 00318 #define SPIx_MISO_AF (SPIx_SCK_AF) 00319 00320 /// SPI MOSI AF 00321 #define SPIx_MOSI_AF (SPIx_SCK_AF) 00322 00323 /** 00324 * @} 00325 */ 00326 00327 /** 00328 * @} 00329 */ 00330 00331 #ifdef __cplusplus 00332 } 00333 #endif 00334 00335 #endif /* __STM32L0XX_NUCLEO_IHM01A1_H */ 00336 00337 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 22:53:31 by
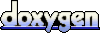