
X-CUBE-SPN1-20150128 example source code for one motor compiled under mbed. Tested OK on Nucleo F401. l6474.cpp is modified from original with defines in l6474_target_config.h to select the original behaviour (motor de-energised when halted), or new mode to continue powering with a (reduced) current in the coils (braking/position hold capability). On F401 avoid using mbed's InterruptIn on pins 10-15 (any port). Beware of other conflicts! L0 & F0 are included but untested.
stm32l0xx_it.cpp
00001 /** 00002 ****************************************************************************** 00003 * @file Multi/Examples/MotionControl/IHM01A1_ExampleFor1Motor/Src/stm32l0xx_it.c 00004 * @author IPC Rennes 00005 * @version V1.5.0 00006 * @date November 12, 2014 00007 * @brief Main Interrupt Service Routines. 00008 * This file provides template for all exceptions handler and 00009 * peripherals interrupt service routine. 00010 ****************************************************************************** 00011 * @attention 00012 * 00013 * <h2><center>© COPYRIGHT(c) 2014 STMicroelectronics</center></h2> 00014 * 00015 * Redistribution and use in source and binary forms, with or without modification, 00016 * are permitted provided that the following conditions are met: 00017 * 1. Redistributions of source code must retain the above copyright notice, 00018 * this list of conditions and the following disclaimer. 00019 * 2. Redistributions in binary form must reproduce the above copyright notice, 00020 * this list of conditions and the following disclaimer in the documentation 00021 * and/or other materials provided with the distribution. 00022 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00023 * may be used to endorse or promote products derived from this software 00024 * without specific prior written permission. 00025 * 00026 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00027 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00028 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00029 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00030 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00031 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00032 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00033 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00034 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00035 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00036 * 00037 ****************************************************************************** 00038 */ 00039 #ifdef TARGET_STM32L0 00040 /* Includes ------------------------------------------------------------------*/ 00041 #include "mbed.h" 00042 #include "stm32l0xx_it.h" 00043 #include "motorcontrol.h" 00044 00045 /** @addtogroup Interrupt_Handlers 00046 * @{ 00047 */ 00048 00049 /* Private typedef -----------------------------------------------------------*/ 00050 /* Private define ------------------------------------------------------------*/ 00051 /* Private macro -------------------------------------------------------------*/ 00052 /* Private variables ---------------------------------------------------------*/ 00053 extern TIM_HandleTypeDef hTimPwm1; 00054 extern TIM_HandleTypeDef hTimPwm2; 00055 extern TIM_HandleTypeDef hTimPwm3; 00056 00057 /* Private function prototypes -----------------------------------------------*/ 00058 /* Private functions ---------------------------------------------------------*/ 00059 00060 00061 /******************************************************************************/ 00062 /* Cortex-M0+ Processor Exceptions Handlers */ 00063 /******************************************************************************/ 00064 00065 /** 00066 * @brief This function handles NMI exception. 00067 * @param None 00068 * @retval None 00069 */ 00070 void NMI_Handler(void) 00071 { 00072 } 00073 00074 /** 00075 * @brief This function handles Hard Fault exception. 00076 * @param None 00077 * @retval None 00078 */ 00079 void HardFault_Handler(void) 00080 { 00081 /* Go to infinite loop when Hard Fault exception occurs */ 00082 while (1) 00083 { 00084 } 00085 } 00086 00087 /** 00088 * @brief This function handles SVCall exception. 00089 * @param None 00090 * @retval None 00091 */ 00092 void SVC_Handler(void) 00093 { 00094 } 00095 00096 /** 00097 * @brief This function handles Debug Monitor exception. 00098 * @param None 00099 * @retval None 00100 */ 00101 void DebugMon_Handler(void) 00102 { 00103 } 00104 00105 /** 00106 * @brief This function handles PendSVC exception. 00107 * @param None 00108 * @retval None 00109 */ 00110 void PendSV_Handler(void) 00111 { 00112 } 00113 00114 /** 00115 * @brief This function handles SysTick Handler. 00116 * @param None 00117 * @retval None 00118 */ 00119 void SysTick_Handler(void) 00120 { 00121 HAL_IncTick(); 00122 } 00123 00124 /******************************************************************************/ 00125 /* STM32L0xx Peripherals Interrupt Handlers */ 00126 /* Add here the Interrupt Handler for the used peripheral(s) (PPP), for the */ 00127 /* available peripheral interrupt handler's name please refer to the startup */ 00128 /* file (startup_stm32l0xx.s). */ 00129 /******************************************************************************/ 00130 00131 /** 00132 * @brief This function handles interrupt for External lines 4 to 15 00133 * @param None 00134 * @retval None 00135 */ 00136 void EXTI4_15_IRQHandler(void) 00137 { 00138 HAL_GPIO_EXTI_IRQHandler(GPIO_PIN_10); 00139 } 00140 00141 /** 00142 * @brief This function handles TIM2 interrupt request. 00143 * @param None 00144 * @retval None 00145 */ 00146 void TIM2_IRQHandler(void) 00147 { 00148 HAL_TIM_IRQHandler(&hTimPwm2); 00149 } 00150 00151 /** 00152 * @brief This function handles TIM22 interrupt request. 00153 * @param None 00154 * @retval None 00155 */ 00156 void TIM22_IRQHandler(void) 00157 { 00158 HAL_TIM_IRQHandler(&hTimPwm1); 00159 } 00160 00161 /** 00162 * @brief This function handles TIM21 interrupt request. 00163 * @param None 00164 * @retval None 00165 */ 00166 void TIM21_IRQHandler(void) 00167 { 00168 HAL_TIM_IRQHandler(&hTimPwm3); 00169 } 00170 /** 00171 * @brief This function handles PPP interrupt request. 00172 * @param None 00173 * @retval None 00174 */ 00175 /*void PPP_IRQHandler(void) 00176 { 00177 }*/ 00178 00179 /** 00180 * @} 00181 */ 00182 #endif 00183 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 22:53:31 by
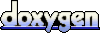