
X-CUBE-SPN1-20150128 example source code for one motor compiled under mbed. Tested OK on Nucleo F401. l6474.cpp is modified from original with defines in l6474_target_config.h to select the original behaviour (motor de-energised when halted), or new mode to continue powering with a (reduced) current in the coils (braking/position hold capability). On F401 avoid using mbed's InterruptIn on pins 10-15 (any port). Beware of other conflicts! L0 & F0 are included but untested.
stm32f4xx_nucleo_ihm01a1.h
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f4xx_nucleo_ihm01a1.h 00004 * @author IPC Rennes 00005 * @version V1.5.0 00006 * @date November 12, 2014 00007 * @brief Header for BSP driver for x-nucleo-ihm01a1 Nucleo extension board 00008 * (based on L6474) 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2014 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Define to prevent recursive inclusion -------------------------------------*/ 00040 #ifndef __STM32F4XX_NUCLEO_IHM01A1_H 00041 #define __STM32F4XX_NUCLEO_IHM01A1_H 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 /* Includes ------------------------------------------------------------------*/ 00048 //mbed #include "stm32f4xx_nucleo.h" 00049 00050 /** @addtogroup BSP 00051 * @{ 00052 */ 00053 00054 /** @addtogroup STM32F4XX_NUCLEO_IHM01A1 00055 * @{ 00056 */ 00057 00058 /** @defgroup IHM01A1_Board_Private_Function_Prototypes 00059 * @{ 00060 */ 00061 00062 void BSP_MotorControlBoard_Delay(uint32_t delay); //Delay of the requested number of milliseconds 00063 void BSP_MotorControlBoard_DisableIrq(void); //Disable Irq 00064 void BSP_MotorControlBoard_EnableIrq(void); //Enable Irq 00065 void BSP_MotorControlBoard_GpioInit(uint8_t nbDevices); //Initialise GPIOs used for L6474s 00066 void BSP_MotorControlBoard_Pwm1SetFreq(uint16_t newFreq); //Set PWM1 frequency and start it 00067 void BSP_MotorControlBoard_Pwm2SetFreq(uint16_t newFreq); //Set PWM2 frequency and start it 00068 void BSP_MotorControlBoard_Pwm3SetFreq(uint16_t newFreq); //Set PWM3 frequency and start it 00069 void BSP_MotorControlBoard_PwmInit(uint8_t deviceId); //Init the PWM of the specified device 00070 void BSP_MotorControlBoard_PwmStop(uint8_t deviceId); //Stop the PWM of the specified device 00071 void BSP_MotorControlBoard_ReleaseReset(void); //Reset the L6474 reset pin 00072 void BSP_MotorControlBoard_Reset(void); //Set the L6474 reset pin 00073 void BSP_MotorControlBoard_SetDirectionGpio(uint8_t deviceId, uint8_t gpioState); //Set direction GPIO 00074 uint8_t BSP_MotorControlBoard_SpiInit(void); //Initialise the SPI used for L6474s 00075 uint8_t BSP_MotorControlBoard_SpiWriteBytes(uint8_t *pByteToTransmit, uint8_t *pReceivedByte, uint8_t nbDevices); //Write bytes to the L6474s via SPI 00076 00077 /* Exported Constants --------------------------------------------------------*/ 00078 00079 /** @defgroup IHM01A1_Exported_Constants 00080 * @{ 00081 */ 00082 00083 /******************************************************************************/ 00084 /* USE_STM32F4XX_NUCLEO */ 00085 /******************************************************************************/ 00086 00087 /** @defgroup Constants_For_STM32F4XX_NUCLEO 00088 * @{ 00089 */ 00090 /// Interrupt line used for L6474 FLAG 00091 #define EXTI_MCU_LINE_IRQn (EXTI15_10_IRQn) 00092 00093 /// Timer used for PWM1 00094 #define BSP_MOTOR_CONTROL_BOARD_TIMER_PWM1 (TIM3) 00095 00096 /// Timer used for PWM2 00097 #define BSP_MOTOR_CONTROL_BOARD_TIMER_PWM2 (TIM2) 00098 00099 /// Timer used for PWM3 00100 #define BSP_MOTOR_CONTROL_BOARD_TIMER_PWM3 (TIM4) 00101 00102 /// Channel Timer used for PWM1 00103 #define BSP_MOTOR_CONTROL_BOARD_CHAN_TIMER_PWM1 (TIM_CHANNEL_2) 00104 00105 /// Channel Timer used for PWM2 00106 #define BSP_MOTOR_CONTROL_BOARD_CHAN_TIMER_PWM2 (TIM_CHANNEL_2) 00107 00108 /// Channel Timer used for PWM3 00109 #define BSP_MOTOR_CONTROL_BOARD_CHAN_TIMER_PWM3 (TIM_CHANNEL_3) 00110 00111 /// HAL Active Channel Timer used for PWM1 00112 #define BSP_MOTOR_CONTROL_BOARD_HAL_ACT_CHAN_TIMER_PWM1 (HAL_TIM_ACTIVE_CHANNEL_2) 00113 00114 /// HAL Active Channel Timer used for PWM2 00115 #define BSP_MOTOR_CONTROL_BOARD_HAL_ACT_CHAN_TIMER_PWM2 (HAL_TIM_ACTIVE_CHANNEL_2) 00116 00117 /// HAL Active Channel Timer used for PWM3 00118 #define BSP_MOTOR_CONTROL_BOARD_HAL_ACT_CHAN_TIMER_PWM3 (HAL_TIM_ACTIVE_CHANNEL_3) 00119 00120 /// Timer Clock Enable for PWM1 00121 #define __BSP_MOTOR_CONTROL_BOARD_TIMER_PWM1_CLCK_ENABLE() __TIM3_CLK_ENABLE() 00122 00123 /// Timer Clock Enable for PWM2 00124 #define __BSP_MOTOR_CONTROL_BOARD_TIMER_PWM2_CLCK_ENABLE() __TIM2_CLK_ENABLE() 00125 00126 /// Timer Clock Enable for PWM1 00127 #define __BSP_MOTOR_CONTROL_BOARD_TIMER_PWM3_CLCK_ENABLE() __TIM4_CLK_ENABLE() 00128 00129 /// Timer Clock Disable for PWM1 00130 #define __BSP_MOTOR_CONTROL_BOARD_TIMER_PWM1_CLCK_DISABLE() __TIM3_CLK_DISABLE() 00131 00132 /// Timer Clock Disable for PWM2 00133 #define __BSP_MOTOR_CONTROL_BOARD_TIMER_PWM2_CLCK_DISABLE() __TIM2_CLK_DISABLE() 00134 00135 /// Timer Clock Disable for PWM3 00136 #define __BSP_MOTOR_CONTROL_BOARD_TIMER_PWM3_CLCK_DISABLE() __TIM4_CLK_DISABLE() 00137 00138 /// PWM1 global interrupt 00139 #define BSP_MOTOR_CONTROL_BOARD_PWM1_IRQn (TIM3_IRQn) 00140 00141 /// PWM2 global interrupt 00142 #define BSP_MOTOR_CONTROL_BOARD_PWM2_IRQn (TIM2_IRQn) 00143 00144 /// PWM3 global interrupt 00145 #define BSP_MOTOR_CONTROL_BOARD_PWM3_IRQn (TIM4_IRQn) 00146 00147 /// PWM1 GPIO alternate function 00148 #define BSP_MOTOR_CONTROL_BOARD_AFx_TIMx_PWM1 (GPIO_AF2_TIM3) 00149 00150 /// PWM2 GPIO alternate function 00151 #define BSP_MOTOR_CONTROL_BOARD_AFx_TIMx_PWM2 (GPIO_AF1_TIM2) 00152 00153 #ifndef BSP_MOTOR_CONTROL_BOARD_USE_SPI2 00154 /// SPI SCK AF 00155 #define SPIx_SCK_AF (GPIO_AF5_SPI1) 00156 #else /* #ifndef BSP_MOTOR_CONTROL_BOARD_USE_SPI2 */ 00157 /// SPI SCK AF 00158 #define SPIx_SCK_AF (GPIO_AF5_SPI2) 00159 #endif /* #ifndef BSP_MOTOR_CONTROL_BOARD_USE_SPI2 */ 00160 00161 /// PWM1 frequency rescaler (1 for HW PWM, 2 for SW PWM) 00162 #define BSP_MOTOR_CONTROL_BOARD_PWM1_FREQ_RESCALER (1) 00163 /// PWM2 frequency rescaler (1 for HW PWM, 2 for SW PWM) 00164 #define BSP_MOTOR_CONTROL_BOARD_PWM2_FREQ_RESCALER (1) 00165 /// PWM3 frequency rescaler (1 for HW PWM, 2 for SW PWM) 00166 #define BSP_MOTOR_CONTROL_BOARD_PWM3_FREQ_RESCALER (2) 00167 00168 /** 00169 * @} 00170 */ 00171 00172 /******************************************************************************/ 00173 /* Independent plateform definitions */ 00174 /******************************************************************************/ 00175 00176 /** @defgroup Constants_For_All_Nucleo_Platforms 00177 * @{ 00178 */ 00179 00180 /// GPIO Pin used for the L6474 flag pin 00181 #define BSP_MOTOR_CONTROL_BOARD_FLAG_PIN (GPIO_PIN_10) 00182 /// GPIO port used for the L6474 flag pin 00183 #define BSP_MOTOR_CONTROL_BOARD_FLAG_PORT (GPIOA) 00184 00185 /// GPIO Pin used for the L6474 step clock pin of device 0 00186 #define BSP_MOTOR_CONTROL_BOARD_PWM_1_PIN (GPIO_PIN_7) 00187 /// GPIO Port used for the L6474 step clock pin of device 0 00188 #define BSP_MOTOR_CONTROL_BOARD_PWM_1_PORT (GPIOC) 00189 00190 /// GPIO Pin used for the L6474 step clock pin of device 1 00191 #define BSP_MOTOR_CONTROL_BOARD_PWM_2_PIN (GPIO_PIN_3) 00192 /// GPIO port used for the L6474 step clock pin of device 1 00193 #define BSP_MOTOR_CONTROL_BOARD_PWM_2_PORT (GPIOB) 00194 00195 /// GPIO Pin used for the L6474 step clock pin of device 2 00196 #define BSP_MOTOR_CONTROL_BOARD_PWM_3_PIN (GPIO_PIN_10) 00197 /// GPIO port used for the L6474 step clock pin of device 2 00198 #define BSP_MOTOR_CONTROL_BOARD_PWM_3_PORT (GPIOB) 00199 00200 /// GPIO Pin used for the L6474 direction pin of device 0 00201 #define BSP_MOTOR_CONTROL_BOARD_DIR_1_PIN (GPIO_PIN_8) 00202 /// GPIO port used for the L6474 direction pin of device 0 00203 #define BSP_MOTOR_CONTROL_BOARD_DIR_1_PORT (GPIOA) 00204 00205 /// GPIO Pin used for the L6474 direction pin of device 1 00206 #define BSP_MOTOR_CONTROL_BOARD_DIR_2_PIN (GPIO_PIN_5) 00207 /// GPIO port used for the L6474 direction pin of device 1 00208 #define BSP_MOTOR_CONTROL_BOARD_DIR_2_PORT (GPIOB) 00209 00210 /// GPIO Pin used for the L6474 direction pin of device 2 00211 #define BSP_MOTOR_CONTROL_BOARD_DIR_3_PIN (GPIO_PIN_4) 00212 /// GPIO port used for the L6474 direction pin of device 2 00213 #define BSP_MOTOR_CONTROL_BOARD_DIR_3_PORT (GPIOB) 00214 00215 /// GPIO Pin used for the L6474 reset pin 00216 #define BSP_MOTOR_CONTROL_BOARD_RESET_PIN (GPIO_PIN_9) 00217 /// GPIO port used for the L6474 reset pin 00218 #define BSP_MOTOR_CONTROL_BOARD_RESET_PORT (GPIOA) 00219 00220 /// GPIO Pin used for the L6474 SPI chip select pin 00221 #define BSP_MOTOR_CONTROL_BOARD_CS_PIN (GPIO_PIN_6) 00222 /// GPIO port used for the L6474 SPI chip select pin 00223 #define BSP_MOTOR_CONTROL_BOARD_CS_PORT (GPIOB) 00224 00225 /* Definition for SPIx clock resources */ 00226 00227 #ifndef BSP_MOTOR_CONTROL_BOARD_USE_SPI2 00228 /* Default SPI is SPI1 */ 00229 00230 /// Used SPI 00231 #define SPIx (SPI1) 00232 00233 /// SPI clock enable 00234 #define SPIx_CLK_ENABLE() __SPI1_CLK_ENABLE() 00235 00236 /// SPI SCK enable 00237 #define SPIx_SCK_GPIO_CLK_ENABLE() __GPIOA_CLK_ENABLE() 00238 00239 /// SPI MISO enable 00240 #define SPIx_MISO_GPIO_CLK_ENABLE() __GPIOA_CLK_ENABLE() 00241 00242 /// SPI MOSI enable 00243 #define SPIx_MOSI_GPIO_CLK_ENABLE() __GPIOA_CLK_ENABLE() 00244 00245 /// SPI Force reset 00246 #define SPIx_FORCE_RESET() __SPI1_FORCE_RESET() 00247 00248 /// SPI Release reset 00249 #define SPIx_RELEASE_RESET() __SPI1_RELEASE_RESET() 00250 00251 /// SPI SCK pin 00252 #define SPIx_SCK_PIN (GPIO_PIN_5) 00253 00254 /// SPI SCK port 00255 #define SPIx_SCK_GPIO_PORT (GPIOA) 00256 00257 00258 /// SPI MISO pin 00259 #define SPIx_MISO_PIN (GPIO_PIN_6) 00260 00261 /// SPI MISO port 00262 #define SPIx_MISO_GPIO_PORT (GPIOA) 00263 00264 /// SPI MOSI pin 00265 #define SPIx_MOSI_PIN (GPIO_PIN_7) 00266 00267 /// SPI MOSI port 00268 #define SPIx_MOSI_GPIO_PORT (GPIOA) 00269 00270 #else /* USE SPI2 */ 00271 00272 /// Used SPI 00273 #define SPIx (SPI2) 00274 00275 /// SPI clock enable 00276 #define SPIx_CLK_ENABLE() __SPI2_CLK_ENABLE() 00277 00278 /// SPI SCK enable 00279 #define SPIx_SCK_GPIO_CLK_ENABLE() __GPIOB_CLK_ENABLE() 00280 00281 /// SPI MISO enable 00282 #define SPIx_MISO_GPIO_CLK_ENABLE() __GPIOB_CLK_ENABLE() 00283 00284 /// SPI MOSI enable 00285 #define SPIx_MOSI_GPIO_CLK_ENABLE() __GPIOB_CLK_ENABLE() 00286 00287 /// SPI Force reset 00288 #define SPIx_FORCE_RESET() __SPI2_FORCE_RESET() 00289 00290 /// SPI Release reset 00291 #define SPIx_RELEASE_RESET() __SPI2_RELEASE_RESET() 00292 00293 /// SPI SCK pin 00294 #define SPIx_SCK_PIN (GPIO_PIN_13) 00295 00296 /// SPI SCK port 00297 #define SPIx_SCK_GPIO_PORT (GPIOB) 00298 00299 /// SPI MISO pin 00300 #define SPIx_MISO_PIN (GPIO_PIN_14) 00301 00302 /// SPI MISO port 00303 #define SPIx_MISO_GPIO_PORT (GPIOB) 00304 00305 /// SPI MISO AF 00306 #define SPIx_MISO_AF (SPIx_SCK_AF) 00307 00308 /// SPI MOSI pin 00309 #define SPIx_MOSI_PIN (GPIO_PIN_15) 00310 00311 /// SPI MOSI port 00312 #define SPIx_MOSI_GPIO_PORT (GPIOB) 00313 00314 #endif 00315 00316 /// SPI MISO AF 00317 #define SPIx_MISO_AF (SPIx_SCK_AF) 00318 00319 /// SPI MOSI AF 00320 #define SPIx_MOSI_AF (SPIx_SCK_AF) 00321 00322 /** 00323 * @} 00324 */ 00325 00326 /** 00327 * @} 00328 */ 00329 00330 #ifdef __cplusplus 00331 } 00332 #endif 00333 00334 #endif /* __STM32F4XX_NUCLEO_IHM01A1_H */ 00335 00336 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 22:53:31 by
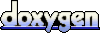