
X-CUBE-SPN1-20150128 example source code for one motor compiled under mbed. Tested OK on Nucleo F401. l6474.cpp is modified from original with defines in l6474_target_config.h to select the original behaviour (motor de-energised when halted), or new mode to continue powering with a (reduced) current in the coils (braking/position hold capability). On F401 avoid using mbed's InterruptIn on pins 10-15 (any port). Beware of other conflicts! L0 & F0 are included but untested.
stm32f4xx_it.cpp
00001 /** 00002 ****************************************************************************** 00003 * @file Multi/Examples/MotionControl/IHM01A1_ExampleFor1Motor/Src/stm32f4xx_it.c 00004 * @author IPC Rennes 00005 * @version V1.5.0 00006 * @date November 12, 2014 00007 * @brief Main Interrupt Service Routines. 00008 * This file provides template for all exceptions handler and 00009 * peripherals interrupt service routine. 00010 ****************************************************************************** 00011 * @attention 00012 * 00013 * <h2><center>© COPYRIGHT(c) 2014 STMicroelectronics</center></h2> 00014 * 00015 * Redistribution and use in source and binary forms, with or without modification, 00016 * are permitted provided that the following conditions are met: 00017 * 1. Redistributions of source code must retain the above copyright notice, 00018 * this list of conditions and the following disclaimer. 00019 * 2. Redistributions in binary form must reproduce the above copyright notice, 00020 * this list of conditions and the following disclaimer in the documentation 00021 * and/or other materials provided with the distribution. 00022 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00023 * may be used to endorse or promote products derived from this software 00024 * without specific prior written permission. 00025 * 00026 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00027 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00028 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00029 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00030 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00031 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00032 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00033 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00034 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00035 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00036 * 00037 ****************************************************************************** 00038 */ 00039 #ifdef TARGET_STM32F4 00040 /* Includes ------------------------------------------------------------------*/ 00041 #include "mbed.h" 00042 #include "stm32f4xx_it.h" 00043 #include "motorcontrol.h" 00044 00045 /** @addtogroup Interrupt_Handlers 00046 * @{ 00047 */ 00048 00049 /* Private typedef -----------------------------------------------------------*/ 00050 /* Private define ------------------------------------------------------------*/ 00051 /* Private macro -------------------------------------------------------------*/ 00052 /* Private variables ---------------------------------------------------------*/ 00053 extern TIM_HandleTypeDef hTimPwm1; 00054 extern TIM_HandleTypeDef hTimPwm2; 00055 extern TIM_HandleTypeDef hTimPwm3; 00056 00057 /* Private function prototypes -----------------------------------------------*/ 00058 /* Private functions ---------------------------------------------------------*/ 00059 00060 00061 /******************************************************************************/ 00062 /* Cortex-M4 Processor Exceptions Handlers */ 00063 /******************************************************************************/ 00064 00065 /** 00066 * @brief This function handles NMI exception. 00067 * @param None 00068 * @retval None 00069 */ 00070 void NMI_Handler(void) 00071 { 00072 } 00073 00074 /** 00075 * @brief This function handles Hard Fault exception. 00076 * @param None 00077 * @retval None 00078 */ 00079 void HardFault_Handler(void) 00080 { 00081 /* Go to infinite loop when Hard Fault exception occurs */ 00082 while (1) 00083 { 00084 } 00085 } 00086 00087 /** 00088 * @brief This function handles Memory Manage exception. 00089 * @param None 00090 * @retval None 00091 */ 00092 void MemManage_Handler(void) 00093 { 00094 /* Go to infinite loop when Memory Manage exception occurs */ 00095 while (1) 00096 { 00097 } 00098 } 00099 00100 /** 00101 * @brief This function handles Bus Fault exception. 00102 * @param None 00103 * @retval None 00104 */ 00105 void BusFault_Handler(void) 00106 { 00107 /* Go to infinite loop when Bus Fault exception occurs */ 00108 while (1) 00109 { 00110 } 00111 } 00112 00113 /** 00114 * @brief This function handles Usage Fault exception. 00115 * @param None 00116 * @retval None 00117 */ 00118 void UsageFault_Handler(void) 00119 { 00120 /* Go to infinite loop when Usage Fault exception occurs */ 00121 while (1) 00122 { 00123 } 00124 } 00125 00126 /** 00127 * @brief This function handles SVCall exception. 00128 * @param None 00129 * @retval None 00130 */ 00131 void SVC_Handler(void) 00132 { 00133 } 00134 00135 /** 00136 * @brief This function handles Debug Monitor exception. 00137 * @param None 00138 * @retval None 00139 */ 00140 void DebugMon_Handler(void) 00141 { 00142 } 00143 00144 /** 00145 * @brief This function handles PendSVC exception. 00146 * @param None 00147 * @retval None 00148 */ 00149 void PendSV_Handler(void) 00150 { 00151 } 00152 00153 /** 00154 * @brief This function handles SysTick Handler. 00155 * @param None 00156 * @retval None 00157 */ 00158 void SysTick_Handler(void) 00159 { 00160 HAL_IncTick(); 00161 } 00162 00163 /******************************************************************************/ 00164 /* STM32F4xx Peripherals Interrupt Handlers */ 00165 /* Add here the Interrupt Handler for the used peripheral(s) (PPP), for the */ 00166 /* available peripheral interrupt handler's name please refer to the startup */ 00167 /* file (startup_stm32f4xx.s). */ 00168 /******************************************************************************/ 00169 00170 /** 00171 * @brief This function handles interrupt for External lines 10 to 15 00172 * @param None 00173 * @retval None 00174 */ 00175 void EXTI15_10_IRQHandler(void) 00176 { 00177 HAL_GPIO_EXTI_IRQHandler(GPIO_PIN_10); 00178 } 00179 00180 /** 00181 * @brief This function handles TIM2 interrupt request. 00182 * @param None 00183 * @retval None 00184 */ 00185 void TIM2_IRQHandler(void) 00186 { 00187 HAL_TIM_IRQHandler(&hTimPwm2); 00188 } 00189 00190 /** 00191 * @brief This function handles TIM3 interrupt request. 00192 * @param None 00193 * @retval None 00194 */ 00195 void TIM3_IRQHandler(void) 00196 { 00197 HAL_TIM_IRQHandler(&hTimPwm1); 00198 } 00199 00200 /** 00201 * @brief This function handles TIM4 interrupt request. 00202 * @param None 00203 * @retval None 00204 */ 00205 void TIM4_IRQHandler(void) 00206 { 00207 HAL_TIM_IRQHandler(&hTimPwm3); 00208 } 00209 /** 00210 * @brief This function handles PPP interrupt request. 00211 * @param None 00212 * @retval None 00213 */ 00214 /*void PPP_IRQHandler(void) 00215 { 00216 }*/ 00217 00218 /** 00219 * @} 00220 */ 00221 #endif 00222 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 22:53:31 by
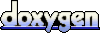