
X-CUBE-SPN1-20150128 example source code for one motor compiled under mbed. Tested OK on Nucleo F401. l6474.cpp is modified from original with defines in l6474_target_config.h to select the original behaviour (motor de-energised when halted), or new mode to continue powering with a (reduced) current in the coils (braking/position hold capability). On F401 avoid using mbed's InterruptIn on pins 10-15 (any port). Beware of other conflicts! L0 & F0 are included but untested.
stdint.h
00001 /* Copyright (C) ARM Ltd., 1999,2014 */ 00002 /* All rights reserved */ 00003 00004 /* 00005 * RCS $Revision: 185525 $ 00006 * Checkin $Date: 2014-05-29 12:44:48 +0100 (Thu, 29 May 2014) $ 00007 * Revising $Author: agrant $ 00008 */ 00009 00010 #ifndef __stdint_h 00011 #define __stdint_h 00012 #define __ARMCLIB_VERSION 5060001 00013 00014 #ifdef __INT64_TYPE__ 00015 /* armclang predefines '__INT64_TYPE__' and '__INT64_C_SUFFIX__' */ 00016 #define __INT64 __INT64_TYPE__ 00017 #else 00018 /* armcc has builtin '__int64' which can be used in --strict mode */ 00019 #define __INT64 __int64 00020 #define __INT64_C_SUFFIX__ ll 00021 #endif 00022 #define __PASTE2(x, y) x ## y 00023 #define __PASTE(x, y) __PASTE2(x, y) 00024 #define __INT64_C(x) __ESCAPE__(__PASTE(x, __INT64_C_SUFFIX__)) 00025 #define __UINT64_C(x) __ESCAPE__(__PASTE(x ## u, __INT64_C_SUFFIX__)) 00026 #if defined(__clang__) || (defined(__ARMCC_VERSION) && !defined(__STRICT_ANSI__)) 00027 /* armclang and non-strict armcc allow 'long long' in system headers */ 00028 #define __LONGLONG long long 00029 #else 00030 /* strict armcc has '__int64' */ 00031 #define __LONGLONG __int64 00032 #endif 00033 00034 #ifndef __STDINT_DECLS 00035 #define __STDINT_DECLS 00036 00037 #undef __CLIBNS 00038 00039 #ifdef __cplusplus 00040 namespace std { 00041 #define __CLIBNS std:: 00042 extern "C" { 00043 #else 00044 #define __CLIBNS 00045 #endif /* __cplusplus */ 00046 00047 00048 /* 00049 * 'signed' is redundant below, except for 'signed char' and if 00050 * the typedef is used to declare a bitfield. 00051 */ 00052 00053 /* 7.18.1.1 */ 00054 00055 /* exact-width signed integer types */ 00056 typedef signed char int8_t; 00057 typedef signed short int int16_t; 00058 typedef signed int int32_t; 00059 typedef signed __INT64 int64_t; 00060 00061 /* exact-width unsigned integer types */ 00062 typedef unsigned char uint8_t; 00063 typedef unsigned short int uint16_t; 00064 typedef unsigned int uint32_t; 00065 typedef unsigned __INT64 uint64_t; 00066 00067 /* 7.18.1.2 */ 00068 00069 /* smallest type of at least n bits */ 00070 /* minimum-width signed integer types */ 00071 typedef signed char int_least8_t; 00072 typedef signed short int int_least16_t; 00073 typedef signed int int_least32_t; 00074 typedef signed __INT64 int_least64_t; 00075 00076 /* minimum-width unsigned integer types */ 00077 typedef unsigned char uint_least8_t; 00078 typedef unsigned short int uint_least16_t; 00079 typedef unsigned int uint_least32_t; 00080 typedef unsigned __INT64 uint_least64_t; 00081 00082 /* 7.18.1.3 */ 00083 00084 /* fastest minimum-width signed integer types */ 00085 typedef signed int int_fast8_t; 00086 typedef signed int int_fast16_t; 00087 typedef signed int int_fast32_t; 00088 typedef signed __INT64 int_fast64_t; 00089 00090 /* fastest minimum-width unsigned integer types */ 00091 typedef unsigned int uint_fast8_t; 00092 typedef unsigned int uint_fast16_t; 00093 typedef unsigned int uint_fast32_t; 00094 typedef unsigned __INT64 uint_fast64_t; 00095 00096 /* 7.18.1.4 integer types capable of holding object pointers */ 00097 #if __sizeof_ptr == 8 00098 typedef signed __INT64 intptr_t; 00099 typedef unsigned __INT64 uintptr_t; 00100 #else 00101 typedef signed int intptr_t; 00102 typedef unsigned int uintptr_t; 00103 #endif 00104 00105 /* 7.18.1.5 greatest-width integer types */ 00106 typedef signed __LONGLONG intmax_t; 00107 typedef unsigned __LONGLONG uintmax_t; 00108 00109 00110 #if !defined(__cplusplus) || defined(__STDC_LIMIT_MACROS) 00111 00112 /* 7.18.2.1 */ 00113 00114 /* minimum values of exact-width signed integer types */ 00115 #define INT8_MIN -128 00116 #define INT16_MIN -32768 00117 #define INT32_MIN (~0x7fffffff) /* -2147483648 is unsigned */ 00118 #define INT64_MIN __INT64_C(~0x7fffffffffffffff) /* -9223372036854775808 is unsigned */ 00119 00120 /* maximum values of exact-width signed integer types */ 00121 #define INT8_MAX 127 00122 #define INT16_MAX 32767 00123 #define INT32_MAX 2147483647 00124 #define INT64_MAX __INT64_C(9223372036854775807) 00125 00126 /* maximum values of exact-width unsigned integer types */ 00127 #define UINT8_MAX 255 00128 #define UINT16_MAX 65535 00129 #define UINT32_MAX 4294967295u 00130 #define UINT64_MAX __UINT64_C(18446744073709551615) 00131 00132 /* 7.18.2.2 */ 00133 00134 /* minimum values of minimum-width signed integer types */ 00135 #define INT_LEAST8_MIN -128 00136 #define INT_LEAST16_MIN -32768 00137 #define INT_LEAST32_MIN (~0x7fffffff) 00138 #define INT_LEAST64_MIN __INT64_C(~0x7fffffffffffffff) 00139 00140 /* maximum values of minimum-width signed integer types */ 00141 #define INT_LEAST8_MAX 127 00142 #define INT_LEAST16_MAX 32767 00143 #define INT_LEAST32_MAX 2147483647 00144 #define INT_LEAST64_MAX __INT64_C(9223372036854775807) 00145 00146 /* maximum values of minimum-width unsigned integer types */ 00147 #define UINT_LEAST8_MAX 255 00148 #define UINT_LEAST16_MAX 65535 00149 #define UINT_LEAST32_MAX 4294967295u 00150 #define UINT_LEAST64_MAX __UINT64_C(18446744073709551615) 00151 00152 /* 7.18.2.3 */ 00153 00154 /* minimum values of fastest minimum-width signed integer types */ 00155 #define INT_FAST8_MIN (~0x7fffffff) 00156 #define INT_FAST16_MIN (~0x7fffffff) 00157 #define INT_FAST32_MIN (~0x7fffffff) 00158 #define INT_FAST64_MIN __INT64_C(~0x7fffffffffffffff) 00159 00160 /* maximum values of fastest minimum-width signed integer types */ 00161 #define INT_FAST8_MAX 2147483647 00162 #define INT_FAST16_MAX 2147483647 00163 #define INT_FAST32_MAX 2147483647 00164 #define INT_FAST64_MAX __INT64_C(9223372036854775807) 00165 00166 /* maximum values of fastest minimum-width unsigned integer types */ 00167 #define UINT_FAST8_MAX 4294967295u 00168 #define UINT_FAST16_MAX 4294967295u 00169 #define UINT_FAST32_MAX 4294967295u 00170 #define UINT_FAST64_MAX __UINT64_C(18446744073709551615) 00171 00172 /* 7.18.2.4 */ 00173 00174 /* minimum value of pointer-holding signed integer type */ 00175 #if __sizeof_ptr == 8 00176 #define INTPTR_MIN INT64_MIN 00177 #else 00178 #define INTPTR_MIN INT32_MIN 00179 #endif 00180 00181 /* maximum value of pointer-holding signed integer type */ 00182 #if __sizeof_ptr == 8 00183 #define INTPTR_MAX INT64_MAX 00184 #else 00185 #define INTPTR_MAX INT32_MAX 00186 #endif 00187 00188 /* maximum value of pointer-holding unsigned integer type */ 00189 #if __sizeof_ptr == 8 00190 #define UINTPTR_MAX INT64_MAX 00191 #else 00192 #define UINTPTR_MAX INT32_MAX 00193 #endif 00194 00195 /* 7.18.2.5 */ 00196 00197 /* minimum value of greatest-width signed integer type */ 00198 #define INTMAX_MIN __ESCAPE__(~0x7fffffffffffffffll) 00199 00200 /* maximum value of greatest-width signed integer type */ 00201 #define INTMAX_MAX __ESCAPE__(9223372036854775807ll) 00202 00203 /* maximum value of greatest-width unsigned integer type */ 00204 #define UINTMAX_MAX __ESCAPE__(18446744073709551615ull) 00205 00206 /* 7.18.3 */ 00207 00208 /* limits of ptrdiff_t */ 00209 #if __sizeof_ptr == 8 00210 #define PTRDIFF_MIN INT64_MIN 00211 #define PTRDIFF_MAX INT64_MAX 00212 #else 00213 #define PTRDIFF_MIN INT32_MIN 00214 #define PTRDIFF_MAX INT32_MAX 00215 #endif 00216 00217 /* limits of sig_atomic_t */ 00218 #define SIG_ATOMIC_MIN (~0x7fffffff) 00219 #define SIG_ATOMIC_MAX 2147483647 00220 00221 /* limit of size_t */ 00222 #if __sizeof_ptr == 8 00223 #define SIZE_MAX UINT64_MAX 00224 #else 00225 #define SIZE_MAX UINT32_MAX 00226 #endif 00227 00228 /* limits of wchar_t */ 00229 /* NB we have to undef and redef because they're defined in both 00230 * stdint.h and wchar.h */ 00231 #undef WCHAR_MIN 00232 #undef WCHAR_MAX 00233 00234 #if defined(__WCHAR32) || (defined(__ARM_SIZEOF_WCHAR_T) && __ARM_SIZEOF_WCHAR_T == 4) 00235 #define WCHAR_MIN 0 00236 #define WCHAR_MAX 0xffffffffU 00237 #else 00238 #define WCHAR_MIN 0 00239 #define WCHAR_MAX 65535 00240 #endif 00241 00242 /* limits of wint_t */ 00243 #define WINT_MIN (~0x7fffffff) 00244 #define WINT_MAX 2147483647 00245 00246 #endif /* __STDC_LIMIT_MACROS */ 00247 00248 #if !defined(__cplusplus) || defined(__STDC_CONSTANT_MACROS) 00249 00250 /* 7.18.4.1 macros for minimum-width integer constants */ 00251 #define INT8_C(x) (x) 00252 #define INT16_C(x) (x) 00253 #define INT32_C(x) (x) 00254 #define INT64_C(x) __INT64_C(x) 00255 00256 #define UINT8_C(x) (x ## u) 00257 #define UINT16_C(x) (x ## u) 00258 #define UINT32_C(x) (x ## u) 00259 #define UINT64_C(x) __UINT64_C(x) 00260 00261 /* 7.18.4.2 macros for greatest-width integer constants */ 00262 #define INTMAX_C(x) __ESCAPE__(x ## ll) 00263 #define UINTMAX_C(x) __ESCAPE__(x ## ull) 00264 00265 #endif /* __STDC_CONSTANT_MACROS */ 00266 00267 #ifdef __cplusplus 00268 } /* extern "C" */ 00269 } /* namespace std */ 00270 #endif /* __cplusplus */ 00271 #endif /* __STDINT_DECLS */ 00272 00273 #ifdef __cplusplus 00274 #ifndef __STDINT_NO_EXPORTS 00275 using ::std::int8_t; 00276 using ::std::int16_t; 00277 using ::std::int32_t; 00278 using ::std::int64_t; 00279 using ::std::uint8_t; 00280 using ::std::uint16_t; 00281 using ::std::uint32_t; 00282 using ::std::uint64_t; 00283 using ::std::int_least8_t; 00284 using ::std::int_least16_t; 00285 using ::std::int_least32_t; 00286 using ::std::int_least64_t; 00287 using ::std::uint_least8_t; 00288 using ::std::uint_least16_t; 00289 using ::std::uint_least32_t; 00290 using ::std::uint_least64_t; 00291 using ::std::int_fast8_t; 00292 using ::std::int_fast16_t; 00293 using ::std::int_fast32_t; 00294 using ::std::int_fast64_t; 00295 using ::std::uint_fast8_t; 00296 using ::std::uint_fast16_t; 00297 using ::std::uint_fast32_t; 00298 using ::std::uint_fast64_t; 00299 using ::std::intptr_t; 00300 using ::std::uintptr_t; 00301 using ::std::intmax_t; 00302 using ::std::uintmax_t; 00303 #endif 00304 #endif /* __cplusplus */ 00305 00306 #undef __INT64 00307 #undef __LONGLONG 00308 00309 #endif /* __stdint_h */ 00310 00311 /* end of stdint.h */
Generated on Tue Jul 12 2022 22:53:31 by
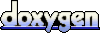