
X-CUBE-SPN1-20150128 example source code for one motor compiled under mbed. Tested OK on Nucleo F401. l6474.cpp is modified from original with defines in l6474_target_config.h to select the original behaviour (motor de-energised when halted), or new mode to continue powering with a (reduced) current in the coils (braking/position hold capability). On F401 avoid using mbed's InterruptIn on pins 10-15 (any port). Beware of other conflicts! L0 & F0 are included but untested.
motor.h
00001 /** 00002 ****************************************************************************** 00003 * @file motor.h 00004 * @author IPC Rennes 00005 * @version V1.3.0 00006 * @date November 12, 2014 00007 * @brief This file contains all the functions prototypes for motor drivers. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2014 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Define to prevent recursive inclusion -------------------------------------*/ 00039 #ifndef __MOTOR_H 00040 #define __MOTOR_H 00041 00042 #ifdef __cplusplus 00043 extern "C" { 00044 #endif 00045 00046 /* Includes ------------------------------------------------------------------*/ 00047 #include <stdint.h> 00048 00049 /** @addtogroup BSP 00050 * @{ 00051 */ 00052 00053 /** @addtogroup Components 00054 * @{ 00055 */ 00056 00057 /** @defgroup Motor 00058 * @{ 00059 */ 00060 00061 /** @defgroup Motor_Exported_Constants 00062 * @{ 00063 */ 00064 00065 /// boolean for false condition 00066 #ifndef FALSE 00067 #define FALSE (0) 00068 #endif 00069 /// boolean for true condition 00070 #ifndef TRUE 00071 #define TRUE (1) 00072 #endif 00073 00074 /** 00075 * @} 00076 */ 00077 00078 /** @defgroup Motor_Exported_Types 00079 * @{ 00080 */ 00081 00082 /** @defgroup Motor_Boolean_Type 00083 * @{ 00084 */ 00085 ///bool Type 00086 //mbed typedef uint8_t bool; 00087 /** 00088 * @} 00089 */ 00090 00091 /** @defgroup Device_Direction_Options 00092 * @{ 00093 */ 00094 /// Direction options 00095 typedef enum { 00096 BACKWARD = 0, 00097 FORWARD = 1 00098 } motorDir_t; 00099 /** 00100 * @} 00101 */ 00102 00103 /** @defgroup Device_Action_Options 00104 * @{ 00105 */ 00106 /// Action options 00107 typedef enum { 00108 ACTION_RESET = ((uint8_t)0x00), 00109 ACTION_COPY = ((uint8_t)0x08) 00110 } motorAction_t; 00111 /** 00112 * @} 00113 */ 00114 00115 /** @defgroup Device_States 00116 * @{ 00117 */ 00118 /// Device states 00119 typedef enum { 00120 ACCELERATING = 0, 00121 DECELERATING = 1, 00122 STEADY = 2, 00123 INACTIVE= 3 00124 } motorState_t; 00125 /** 00126 * @} 00127 */ 00128 00129 /** @defgroup Device_Step_mode 00130 * @{ 00131 */ 00132 /// Stepping options 00133 typedef enum { 00134 STEP_MODE_FULL = ((uint8_t)0x00), 00135 STEP_MODE_HALF = ((uint8_t)0x01), 00136 STEP_MODE_1_4 = ((uint8_t)0x02), 00137 STEP_MODE_1_8 = ((uint8_t)0x03), 00138 STEP_MODE_1_16 = ((uint8_t)0x04), 00139 STEP_MODE_1_32 = ((uint8_t)0x05), 00140 STEP_MODE_1_64 = ((uint8_t)0x06), 00141 STEP_MODE_1_128 = ((uint8_t)0x07) 00142 } motorStepMode_t; 00143 /** 00144 * @} 00145 */ 00146 00147 00148 /** @defgroup Motor_Driver_Structure 00149 * @{ 00150 */ 00151 /// Motor driver structure definition 00152 typedef struct 00153 { 00154 /// Function pointer to Init 00155 void (*Init)(uint8_t); 00156 /// Function pointer to ReadID 00157 uint16_t (*ReadID)(void); 00158 /// Function pointer to AttachErrorHandler 00159 void(*AttachErrorHandler)(void (*callback)(uint16_t)); 00160 /// Function pointer to AttachFlagInterrupt 00161 void (*AttachFlagInterrupt)(void (*callback)(void)); 00162 /// Function pointer to AttachBusyInterrupt 00163 void (*AttachBusyInterrupt)(void (*callback)(void)); 00164 /// Function pointer to FlagInterruptHandler 00165 void (*FlagInterruptHandler)(void); 00166 /// Function pointer to GetAcceleration 00167 uint16_t (*GetAcceleration)(uint8_t); 00168 /// Function pointer to GetCurrentSpeed 00169 uint16_t (*GetCurrentSpeed)(uint8_t); 00170 /// Function pointer to GetDeceleration 00171 uint16_t (*GetDeceleration)(uint8_t); 00172 /// Function pointer to GetDeviceState 00173 motorState_t(*GetDeviceState)(uint8_t); 00174 /// Function pointer to GetFwVersion 00175 uint8_t (*GetFwVersion)(void); 00176 /// Function pointer to GetMark 00177 int32_t (*GetMark)(uint8_t); 00178 /// Function pointer to GetMaxSpeed 00179 uint16_t (*GetMaxSpeed)(uint8_t); 00180 /// Function pointer to GetMinSpeed 00181 uint16_t (*GetMinSpeed)(uint8_t); 00182 /// Function pointer to GetPosition 00183 int32_t (*GetPosition)(uint8_t); 00184 /// Function pointer to GoHome 00185 void (*GoHome)(uint8_t); 00186 /// Function pointer to GoMark 00187 void (*GoMark)(uint8_t); 00188 /// Function pointer to GoTo 00189 void (*GoTo)(uint8_t, int32_t); 00190 /// Function pointer to HardStop 00191 void (*HardStop)(uint8_t); 00192 /// Function pointer to Move 00193 void (*Move)(uint8_t, motorDir_t, uint32_t ); 00194 /// Function pointer to ResetAllDevices 00195 void (*ResetAllDevices)(void); 00196 /// Function pointer to Run 00197 void (*Run)(uint8_t, motorDir_t); 00198 /// Function pointer to SetAcceleration 00199 bool(*SetAcceleration)(uint8_t ,uint16_t ); 00200 /// Function pointer to SetDeceleration 00201 bool(*SetDeceleration)(uint8_t , uint16_t ); 00202 /// Function pointer to SetHome 00203 void (*SetHome)(uint8_t); 00204 /// Function pointer to SetMark 00205 void (*SetMark)(uint8_t); 00206 /// Function pointer to SetMaxSpeed 00207 bool (*SetMaxSpeed)(uint8_t, uint16_t ); 00208 /// Function pointer to SetMinSpeed 00209 bool (*SetMinSpeed)(uint8_t, uint16_t ); 00210 /// Function pointer to SoftStop 00211 bool (*SoftStop)(uint8_t); 00212 /// Function pointer to StepClockHandler 00213 void (*StepClockHandler)(uint8_t deviceId); 00214 /// Function pointer to WaitWhileActive 00215 void (*WaitWhileActive)(uint8_t); 00216 /// Function pointer to CmdDisable 00217 void (*CmdDisable)(uint8_t); 00218 /// Function pointer to CmdEnable 00219 void (*CmdEnable)(uint8_t); 00220 /// Function pointer to CmdGetParam 00221 uint32_t (*CmdGetParam)(uint8_t, uint32_t); 00222 /// Function pointer to CmdGetStatus 00223 uint16_t (*CmdGetStatus)(uint8_t); 00224 /// Function pointer to CmdNop 00225 void (*CmdNop)(uint8_t); 00226 /// Function pointer to CmdSetParam 00227 void (*CmdSetParam)(uint8_t, uint32_t, uint32_t); 00228 /// Function pointer to ReadStatusRegister 00229 uint16_t (*ReadStatusRegister)(uint8_t); 00230 /// Function pointer to ReleaseReset 00231 void (*ReleaseReset)(void); 00232 /// Function pointer to Reset 00233 void (*Reset)(void); 00234 /// Function pointer to SelectStepMode 00235 void (*SelectStepMode)(uint8_t deviceId, motorStepMode_t); 00236 /// Function pointer to SetDirection 00237 void (*SetDirection)(uint8_t, motorDir_t); 00238 /// Function pointer to CmdGoToDir 00239 void (*CmdGoToDir)(uint8_t, motorDir_t, int32_t); 00240 /// Function pointer to CheckBusyHw 00241 uint8_t (*CheckBusyHw)(void); 00242 /// Function pointer to CheckStatusHw 00243 uint8_t (*CheckStatusHw)(void); 00244 /// Function pointer to CmdGoUntil 00245 void (*CmdGoUntil)(uint8_t, motorAction_t, motorDir_t, uint32_t); 00246 /// Function pointer to CmdHardHiZ 00247 void (*CmdHardHiZ)(uint8_t); 00248 /// Function pointer to CmdReleaseSw 00249 void (*CmdReleaseSw)(uint8_t, motorAction_t, motorDir_t); 00250 /// Function pointer to CmdResetDevice 00251 void (*CmdResetDevice)(uint8_t); 00252 /// Function pointer to CmdResetPos 00253 void (*CmdResetPos)(uint8_t); 00254 /// Function pointer to CmdRun 00255 void (*CmdRun)(uint8_t, motorDir_t, uint32_t); 00256 /// Function pointer to CmdSoftHiZ 00257 void (*CmdSoftHiZ)(uint8_t); 00258 /// Function pointer to CmdStepClock 00259 void (*CmdStepClock)(uint8_t, motorDir_t); 00260 /// Function pointer to FetchAndClearAllStatus 00261 void (*FetchAndClearAllStatus)(void); 00262 /// Function pointer to GetFetchedStatus 00263 uint16_t (*GetFetchedStatus)(uint8_t); 00264 /// Function pointer to GetNbDevices 00265 uint8_t (*GetNbDevices)(void); 00266 /// Function pointer to IsDeviceBusy 00267 bool (*IsDeviceBusy)(uint8_t); 00268 /// Function pointer to SendQueuedCommands 00269 void (*SendQueuedCommands)(void); 00270 /// Function pointer to QueueCommands 00271 void (*QueueCommands)(uint8_t, uint8_t, uint32_t); 00272 /// Function pointer to WaitForAllDevicesNotBusy 00273 void (*WaitForAllDevicesNotBusy)(void); 00274 /// Function pointer to ErrorHandler 00275 void (*ErrorHandler)(uint16_t); 00276 /// Function pointer to BusyInterruptHandler 00277 void (*BusyInterruptHandler)(void); 00278 void (*CmdSoftStop)(uint8_t); 00279 }motorDrv_t; 00280 00281 /** 00282 00283 * @} 00284 */ 00285 00286 /** 00287 * @} 00288 */ 00289 00290 /** 00291 * @} 00292 */ 00293 00294 /** 00295 * @} 00296 */ 00297 00298 /** 00299 * @} 00300 */ 00301 00302 #ifdef __cplusplus 00303 } 00304 #endif 00305 00306 #endif /* __MOTOR_H */ 00307 00308 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 22:53:31 by
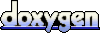