Class library for a TCS3200 Color Sensor Module.
Dependents: LCD PROJETO PROJETO_MECATRONICO Bottle_Tracker_Insper ... more
TCS3200.h
00001 /* TCS3200 Library v1.0 00002 * Copyright (c) 2016 Grant Phillips 00003 * grant.phillips@nmmu.ac.za 00004 * 00005 * 00006 * Permission is hereby granted, free of charge, to any person obtaining a copy 00007 * of this software and associated documentation files (the "Software"), to deal 00008 * in the Software without restriction, including without limitation the rights 00009 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00010 * copies of the Software, and to permit persons to whom the Software is 00011 * furnished to do so, subject to the following conditions: 00012 * 00013 * The above copyright notice and this permission notice shall be included in 00014 * all copies or substantial portions of the Software. 00015 * 00016 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00017 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00018 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00019 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00020 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00021 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00022 * THE SOFTWARE. 00023 */ 00024 00025 #ifndef TCS3200_H 00026 #define TCS3200_H 00027 00028 #include "mbed.h" 00029 00030 /** Class library for a TCS3200 Color Sensor Module. 00031 * 00032 * Example: 00033 * @code 00034 * #include "mbed.h" 00035 * #include "TCS3200.h" 00036 * 00037 * TCS3200 color(PD_8, PD_9, PD_10, PD_11, PA_1); //Create a TCS3200 object 00038 * // S0 S1 S2 S3 OUT 00039 * 00040 * int main() { 00041 * long red, green, blue, clear; 00042 * 00043 * //Set the scaling factor to 100% 00044 * color.SetMode(TCS3200::SCALE_100); 00045 * 00046 * while(1){ 00047 * //Read the HIGH pulse width in nS for each color. 00048 * //The lower the value, the more of that color is detected 00049 * red = color.ReadRed(); 00050 * green = color.ReadGreen(); 00051 * blue = color.ReadBlue(); 00052 * clear = color.ReadClear(); 00053 * 00054 * printf("RED: %10d GREEN: %10d BLUE: %10d CLEAR: %10d ", red, green, blue, clear); 00055 * 00056 * wait(0.1); 00057 * } 00058 * } 00059 * @endcode 00060 */ 00061 00062 class TCS3200 { 00063 public: 00064 enum TCS3200Mode { 00065 POWERDOWN = 0, 00066 SCALE_2 = 1, 00067 SCALE_20 = 2, 00068 SCALE_100 = 3, 00069 }; 00070 00071 /** Create a TCS3200 object connected to the specified pins. 00072 * @param S0 Frequency scaling output pin S0 00073 * @param S1 Frequency scaling output pin S1 00074 * @param S2 Photo diode selection output pin S2 00075 * @param S3 Photo diode selection output pin S3 00076 * @param OUT Frequency input pin 00077 */ 00078 TCS3200(PinName S0, PinName S1, PinName S2, PinName S3, PinName OUT); 00079 00080 /** Reads the output signal's HIGH pulse for RED. 00081 * @param 00082 * None 00083 * @return 00084 * Duration as nanoseconds (ns). 00085 */ 00086 long ReadRed(); 00087 00088 /** Reads the output signal's HIGH pulse for GREEN. 00089 * @param 00090 * None 00091 * @return 00092 * Duration as nanoseconds (ns). 00093 */ 00094 long ReadGreen(); 00095 00096 /** Reads the output signal's HIGH pulse for BLUE. 00097 * @param 00098 * None 00099 * @return 00100 * Duration as nanoseconds (ns). 00101 */ 00102 long ReadBlue(); 00103 00104 /** Reads the output signal's HIGH pulse for CLEAR. 00105 * @param 00106 * None 00107 * @return 00108 * Duration as nanoseconds (ns). 00109 */ 00110 long ReadClear(); 00111 00112 /** Sets the mode of operation. 00113 * @param 00114 * mode POWERDOWN, SCALE_2 (2% scaling), SCALE_20 (20% scaling), SCALE_100 (100% scaling). 00115 * @return 00116 * None 00117 */ 00118 void SetMode(TCS3200Mode mode); 00119 00120 private: 00121 DigitalOut mS0; 00122 DigitalOut mS1; 00123 DigitalOut mS2; 00124 DigitalOut mS3; 00125 InterruptIn signal; 00126 Timer timer; 00127 long pulsewidth; 00128 void HighTrigger(); 00129 void LowTrigger(); 00130 }; 00131 00132 #endif
Generated on Sun Jul 17 2022 19:57:11 by
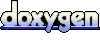