a library that provides a connection to a SHT21 temperature and humidity sensor Author: Graeme Coapes - Newcastle University, graeme.coapes@ncl.ac.uk Date: 29/11/12
Dependents: test_ncleee WeatherStation Temp_hum PROJ ... more
SHT21 Class Reference
SHT21 Connection class, utilizing a I2C interface. More...
#include <SHT21_ncleee.h>
Public Member Functions | |
SHT21 (I2C *i2c) | |
Constructor - create a connection to a SHT21 temperature and humidity sensor through an I2C interface. | |
float | readTemp () |
Read the temperature value from the sensor . | |
float | readHumidity () |
Read the humidity value from the sensor . | |
int | reset () |
Perform a soft-reset of the sensor unit. | |
int | setPrecision (char precision) |
Set the precision of the measuring. |
Detailed Description
SHT21 Connection class, utilizing a I2C interface.
Example:
#include "mbed.h" #include "SHT21_ncleee.h" DigitalOut myled(LED1); Serial pc(USBTX, USBRX); I2C i2c(p28,p27); SHT21 sht(&i2c); int main() { pc.printf("Hello World...\n\tTesting temperature Sensor\n"); int temperature = sht.readTemp(); pc.printf("Temperature is: %d \n", temperature); pc.printf("Experiment complete...\n"); }
Definition at line 112 of file SHT21_ncleee.h.
Constructor & Destructor Documentation
SHT21 | ( | I2C * | i2c ) |
Constructor - create a connection to a SHT21 temperature and humidity sensor through an I2C interface.
- Parameters:
-
*i2c a pointer to the i2c interface that is used for communication
Definition at line 31 of file SHT21_ncleee.cpp.
Member Function Documentation
float readHumidity | ( | ) |
Read the humidity value from the sensor
.
Involves triggering the measuring unit then waiting for 100ms for the measuring to complete before reading the humidity
- Parameters:
-
returns the percentage humidity
Definition at line 127 of file SHT21_ncleee.cpp.
float readTemp | ( | ) |
Read the temperature value from the sensor
.
Involves triggering the measuring unit then waiting for 100ms for the measuring to complete before reading the temperature
- Parameters:
-
returns a value representing the temperature in degrees centigrade
Definition at line 69 of file SHT21_ncleee.cpp.
int reset | ( | ) |
Perform a soft-reset of the sensor unit.
Definition at line 159 of file SHT21_ncleee.cpp.
int setPrecision | ( | char | precision ) |
Set the precision of the measuring.
//Data precision settings
//RH 12 T 14 - default
define SHT_PREC_1214 0x00
//RH 8 T 10
define SHT_PREC_0812 0x01
//RH 10 T 13
define SHT_PREC_1013 0x80
//RH 11 T 11
define SHT_PREC_1111 0x81
- Parameters:
-
precision - the precision, refer to above or datasheet.
Definition at line 165 of file SHT21_ncleee.cpp.
Generated on Tue Jul 12 2022 21:35:21 by
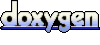