
A guitar Tuner project made by: Guarino Yuri Pota Giuseppe Sito Leonardo It is made up of a circuit of signal amplification(using LM386 OP-AMP), and a circuit of many LEDs that display if the guitar is tuned or not.
main.cpp
00001 #include "mbed.h" 00002 #include "mbed.h" 00003 #include "arm_math.h" 00004 #include "arm_const_structs.h" 00005 #define SAMP_FREQ 2000 00006 00007 InterruptIn button(PC_13); 00008 DigitalOut El(D2); 00009 DigitalOut A(D3); 00010 DigitalOut D(D4); 00011 DigitalOut G(D5); 00012 DigitalOut B(D6); 00013 DigitalOut Eh(D7); 00014 DigitalOut Led_Ok(D9); 00015 DigitalOut Led_Down(D10); 00016 DigitalOut Led_Up(D8); 00017 Serial pc (SERIAL_TX,SERIAL_RX); 00018 AnalogIn analog_value(A0); 00019 const int FFT_LEN = 1024; 00020 const int bins = 1000; 00021 00022 float Ing[FFT_LEN]; 00023 00024 const static arm_cfft_instance_f32 *S; 00025 00026 float samples[FFT_LEN*2]; 00027 float magnitudes[FFT_LEN]; 00028 float freq_window[bins]; 00029 00030 int c =0; 00031 void selectNote() { 00032 c++; 00033 if(c==1) 00034 { 00035 El=1; 00036 A=0; 00037 D=0; 00038 G=0; 00039 B=0; 00040 Eh=0; 00041 } 00042 else if(c==2) 00043 { 00044 El=0; 00045 A=1; 00046 D=0; 00047 G=0; 00048 B=0; 00049 Eh=0; 00050 00051 } 00052 else if(c==3) 00053 { 00054 El=0; 00055 A=0; 00056 D=1; 00057 G=0; 00058 B=0; 00059 Eh=0; 00060 00061 } 00062 00063 else if(c==4) 00064 { 00065 El=0; 00066 A=0; 00067 D=0; 00068 G=1; 00069 B=0; 00070 Eh=0; 00071 00072 } 00073 00074 else if(c==5) 00075 { 00076 El=0; 00077 A=0; 00078 D=0; 00079 G=0; 00080 B=1; 00081 Eh=0; 00082 00083 } 00084 00085 else if(c==6) 00086 { 00087 El=0; 00088 A=0; 00089 D=0; 00090 G=0; 00091 B=0; 00092 Eh=1; 00093 00094 00095 } 00096 else if(c==7) 00097 c=0; 00098 00099 } 00100 int main() { 00101 El=0; 00102 A=0; 00103 D=0; 00104 G=0; 00105 B=0; 00106 Eh=0; 00107 00108 El=1;wait(0.1);El=0; 00109 A=1;wait(0.1);A=0; 00110 D=1;wait(0.1);D=0; 00111 G=1;wait(0.1);G=0; 00112 B=1;wait(0.1);B=0; 00113 Eh=1;wait(0.1);Eh=0; 00114 El=1;A=1;D=1;G=1;B=1;Eh=1;wait(0.2); 00115 El=0;A=0;D=0;G=0;B=0;Eh=0; 00116 Led_Down=1; 00117 Led_Up=0; 00118 Led_Ok=0; 00119 00120 00121 00122 int32_t i = 0; 00123 pc.printf("\r\n\r\nFFT test program!\r\n"); 00124 00125 00126 // Init arm_ccft_32 00127 switch (FFT_LEN) 00128 { 00129 case 16: 00130 S = & arm_cfft_sR_f32_len16; 00131 break; 00132 case 32: 00133 S = & arm_cfft_sR_f32_len32; 00134 break; 00135 case 64: 00136 S = & arm_cfft_sR_f32_len64; 00137 break; 00138 case 128: 00139 S = & arm_cfft_sR_f32_len128; 00140 break; 00141 case 256: 00142 S = & arm_cfft_sR_f32_len256; 00143 break; 00144 case 512: 00145 S = & arm_cfft_sR_f32_len512; 00146 break; 00147 case 1024: 00148 S = & arm_cfft_sR_f32_len1024; 00149 break; 00150 case 2048: 00151 S = & arm_cfft_sR_f32_len2048; 00152 break; 00153 case 4096: 00154 S = & arm_cfft_sR_f32_len4096; 00155 break; 00156 } 00157 while(1) { 00158 button.rise(&selectNote); 00159 for (int k = 0; k < 1024; k++) { 00160 Ing[k] = (short) (analog_value.read_u16() - 0x8000); 00161 wait_us(1e6/SAMP_FREQ); 00162 } 00163 //Riempiamo il vettore per la FFT 00164 for(i = 0; i< FFT_LEN*2; i+=2) 00165 { 00166 00167 samples[i] = Ing[i]; 00168 samples[i+1] = 0; 00169 } 00170 arm_cfft_f32(S, samples, 0, 1); 00171 // Calcola la FFT 00172 arm_cmplx_mag_f32(samples, magnitudes, FFT_LEN); 00173 00174 if(c==1){ 00175 int max=3; 00176 int j=2; 00177 do{ 00178 if (magnitudes[j]>magnitudes[max]&&(j*1000/1024<100)&&(j*1000/1024>30))//restringo la banda a [30Hz, 100Hz] 00179 max=j; 00180 j++; 00181 00182 }while(j<1024/2); 00183 00184 float maxx=(float)max*1000/1024; 00185 00186 if(maxx<82){ 00187 Led_Down=1; 00188 Led_Up=0; 00189 Led_Ok=0; 00190 } 00191 if(maxx>84){ 00192 Led_Down=0; 00193 Led_Up=1; 00194 Led_Ok=0; 00195 } 00196 else if(maxx>82.3 &&maxx<=84){ 00197 Led_Down=0; 00198 Led_Up=0; 00199 Led_Ok=1; 00200 } 00201 } 00202 //--------------A STRING------------------ 00203 if(c==2){ 00204 int max=3; 00205 int j=2; 00206 do{ 00207 00208 if (magnitudes[j]>magnitudes[max]&&(j*1000/1024<130)&&(j*1000/1024>90))//restringo la banda a [90Hz, 130Hz] 00209 max=j; 00210 j++; 00211 00212 }while(j<1024/2); 00213 00214 float maxx=(float)max*1000/1024; 00215 //pc.printf("Il massimo in ampiezza è alla frequenza %f Hertz \n",(float)max*1000/1024); 00216 if(maxx<110){ 00217 Led_Down=1; 00218 Led_Up=0; 00219 Led_Ok=0; 00220 } 00221 if(maxx>112){ 00222 Led_Down=0; 00223 Led_Up=1; 00224 Led_Ok=0; 00225 } 00226 else if(maxx>110 &&maxx<113){ 00227 Led_Down=0; 00228 Led_Up=0; 00229 Led_Ok=1; 00230 } 00231 } 00232 //--------------D STRING------------------ 00233 if(c==3){ 00234 int max=3; 00235 int j=2; 00236 do{ 00237 00238 if (magnitudes[j]>magnitudes[max]&&(j*1000/1024<160)&&(j*1000/1024>130))//restringo la banda a [130Hz, 160Hz] 00239 max=j; 00240 j++; 00241 00242 }while(j<1024/2); 00243 00244 float maxx=(float)max*1000/1024; 00245 //pc.printf("Il massimo in ampiezza è alla frequenza %f Hertz \n",(float)max*1000/1024); 00246 if(maxx<147){ 00247 Led_Down=1; 00248 Led_Up=0; 00249 Led_Ok=0; 00250 } 00251 if(maxx>149){ 00252 Led_Down=0; 00253 Led_Up=1; 00254 Led_Ok=0; 00255 } 00256 else if(maxx>147 &&maxx<149){ 00257 Led_Down=0; 00258 Led_Up=0; 00259 Led_Ok=1; 00260 } 00261 } 00262 //--------------G STRING------------------ 00263 if(c==4){ 00264 int max=3; 00265 int j=2; 00266 do{ 00267 00268 if (magnitudes[j]>magnitudes[max]&&(j*1000/1024<210)&&(j*1000/1024>170))//restringo la banda a [170Hz, 210Hz] 00269 max=j; 00270 j++; 00271 00272 }while(j<1024/2); 00273 00274 float maxx=(float)max*1000/1024; 00275 //pc.printf("Il massimo in ampiezza è alla frequenza %f Hertz \n",(float)max*1000/1024); 00276 if(maxx<196){ 00277 Led_Down=1; 00278 Led_Up=0; 00279 Led_Ok=0; 00280 } 00281 if(maxx>203){ 00282 Led_Down=0; 00283 Led_Up=1; 00284 Led_Ok=0; 00285 } 00286 else if(maxx>=196 &&maxx<=197.5){ 00287 Led_Down=0; 00288 Led_Up=0; 00289 Led_Ok=1; 00290 } 00291 } 00292 //--------------B STRING------------------ 00293 if(c==5){ 00294 int max=3; 00295 int j=2; 00296 do{ 00297 00298 if (magnitudes[j]>magnitudes[max]&&(j*1000/1024<260)&&(j*1000/1024>230))//restringo la banda a [230Hz, 260Hz] 00299 max=j; 00300 j++; 00301 00302 }while(j<1024/2); 00303 00304 float maxx=(float)max*1000/1024; 00305 //pc.printf("Il massimo in ampiezza è alla frequenza %f Hertz \n",(float)max*1000/1024); 00306 if(maxx<244){ 00307 Led_Down=1; 00308 Led_Up=0; 00309 Led_Ok=0; 00310 } 00311 if(maxx>250){ 00312 Led_Down=0; 00313 Led_Up=1; 00314 Led_Ok=0; 00315 } 00316 else if(maxx>246 &&maxx<250){ 00317 Led_Down=0; 00318 Led_Up=0; 00319 Led_Ok=1; 00320 } 00321 } 00322 //--------------Eh STRING------------------ 00323 if(c==6){ 00324 int max=3; 00325 int j=2; 00326 do{ 00327 00328 if (magnitudes[j]>magnitudes[max]&&(j*1000/1024<340)&&(j*1000/1024>310))//restringo la banda a [340Hz, 310Hz] 00329 max=j; 00330 j++; 00331 00332 }while(j<1024/2); 00333 00334 float maxx=(float)max*1000/1024; 00335 //pc.printf("Il massimo in ampiezza è alla frequenza %f Hertz \n",(float)max*1000/1024); 00336 if(maxx<327){ 00337 Led_Down=1; 00338 Led_Up=0; 00339 Led_Ok=0; 00340 } 00341 if(maxx>334){ 00342 Led_Down=0; 00343 Led_Up=1; 00344 Led_Ok=0; 00345 } 00346 else if(maxx>330.3 &&maxx<333.5){ 00347 Led_Down=0; 00348 Led_Up=0; 00349 Led_Ok=1; 00350 } 00351 } 00352 00353 00354 } 00355 } 00356
Generated on Fri Oct 28 2022 03:03:26 by
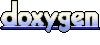