asdf
Dependencies: L3GD20 LSM303DLHC mbed
Flooding.h
00001 #ifndef FLOODING_H 00002 #define FLOODING_H 00003 00004 00005 #include "Mapping.h" 00006 00007 enum MOVE 00008 { 00009 M_NORTH, 00010 M_SOUTH, 00011 M_EAST, 00012 M_WEST, 00013 M_INVALID 00014 }; 00015 00016 unsigned char destX = 8; 00017 unsigned char destY = 8; 00018 unsigned char pathX = 8; 00019 unsigned char pathY = 8; 00020 00021 00022 unsigned char flood[16][16] = 00023 { 00024 {0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00}, 00025 {0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00}, 00026 {0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00}, 00027 {0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00}, 00028 {0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00}, 00029 {0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00}, 00030 {0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00}, 00031 {0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00}, 00032 {0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00}, 00033 {0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00}, 00034 {0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00}, 00035 {0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00}, 00036 {0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00}, 00037 {0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00}, 00038 {0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00}, 00039 {0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00} 00040 }; 00041 00042 void clearFlood() 00043 { 00044 for(unsigned char i = 0; i < (unsigned char)16; i++) 00045 for(unsigned char j = 0; j < (unsigned char)16; j++) 00046 flood[i][j] = 0x00; 00047 } 00048 00049 bool flood_getTop(int i, int j) 00050 { 00051 if(validDimen(i, j) && flood[i][j] == 0) 00052 return !((MAP[i + 1][j] & TOP) == TOP || ((MAP[i][j] & BOTTOM) == BOTTOM)); 00053 00054 return false; 00055 } 00056 00057 bool flood_getRight(int i, int j) 00058 { 00059 if(validDimen(i, j) && flood[i][j] == 0) 00060 return !((MAP[i][j - 1] & RIGHT) == RIGHT || ((MAP[i][j] & LEFT) == LEFT)); 00061 00062 return false; 00063 } 00064 00065 bool flood_getLeft(int i, int j) 00066 { 00067 if(validDimen(i, j) && flood[i][j] == 0) 00068 return !((MAP[i][j + 1] & LEFT) == LEFT || ((MAP[i][j] & RIGHT) == RIGHT)); 00069 00070 return false; 00071 } 00072 00073 bool flood_getBottom(int i, int j) 00074 { 00075 if(validDimen(i, j) && flood[i][j] == 0) 00076 return !((MAP[i - 1][j] & BOTTOM) == BOTTOM || ((MAP[i][j] & TOP) == TOP)); 00077 00078 return false; 00079 } 00080 00081 int flood_getCell(int i, int j) 00082 { 00083 if(validDimen(i, j)) 00084 return flood[i][j]; 00085 00086 return 0; 00087 } 00088 00089 void flood_floodStep(int step) 00090 { 00091 for(int i = 4; i >= 0 ; i--) 00092 { 00093 for(int j = 0; j < 5; j++) 00094 { 00095 if(flood[i][j] == step) 00096 { 00097 if(flood_getTop(i - 1, j)) 00098 { 00099 flood[i - 1][j] = (char) (step + 1); 00100 } 00101 00102 if(flood_getBottom(i + 1, j)) 00103 { 00104 flood[i + 1][j] = (char) (step + 1); 00105 } 00106 00107 if(flood_getLeft(i, j - 1)) 00108 { 00109 flood[i][j - 1] = (char) (step + 1); 00110 } 00111 if(flood_getRight(i, j + 1)) 00112 { 00113 flood[i][j + 1] = (char) (step + 1); 00114 } 00115 } 00116 } 00117 } 00118 } 00119 00120 MOVE flood_bestMove(int x, int y) 00121 { 00122 int target = flood_getCell(x, y) - 1; 00123 00124 if(flood_getCell(x + 1, y) == target && ((MAP[x + 1][y] & TOP) != TOP)) 00125 { 00126 pathX++; 00127 return M_NORTH; 00128 } 00129 if(flood_getCell(x, y - 1) == target && ((MAP[x][y - 1] & RIGHT) != RIGHT)) 00130 { 00131 pathY--; 00132 return M_EAST; 00133 } 00134 00135 if(flood_getCell(x, y + 1) == target && ((MAP[x][y + 1] & LEFT) != LEFT)) 00136 { 00137 pathY++; 00138 return M_WEST; 00139 } 00140 if(flood_getCell(x - 1, y) == target && ((MAP[x - 1][y] & BOTTOM) != BOTTOM)) 00141 { 00142 pathX--; 00143 return M_SOUTH; 00144 } 00145 00146 return M_INVALID; 00147 } 00148 00149 MOVE flood_findPath(int xt, int yt) 00150 { 00151 flood[xt][yt] = 1; 00152 00153 int step = 1; 00154 while(flood[destX][destY] == 0) 00155 { 00156 flood_floodStep(step++); 00157 } 00158 00159 MOVE last = M_INVALID; 00160 00161 while (flood_getCell(pathX, pathY) != 1) 00162 { 00163 last = flood_bestMove(pathX, pathY); 00164 } 00165 00166 return last; 00167 } 00168 00169 #endif
Generated on Tue Jul 12 2022 19:34:20 by
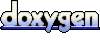