
Makes 100 samples on maximum sample rate and transmits it over UART
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #define SAMPLE_RATE 750000 00002 #define LENGTH_RESULT 100 00003 00004 #include "mbed.h" 00005 #include "adc.h" 00006 00007 //Initialise ADC to maximum SAMPLE_RATE and cclk divide set to 1 00008 ADC adc(SAMPLE_RATE, 1); 00009 00010 Serial uart(USBTX, USBRX); // tx, rx 00011 volatile int result[LENGTH_RESULT]; 00012 00013 int main() { 00014 // Init UART 00015 // uart.baud(256000); 00016 // uart.printf("Requested max sample rate is %u, actual max sample rate is %u.\n", SAMPLE_RATE, adc.actual_sample_rate()); 00017 00018 //Set up ADC on pin 20 00019 adc.setup(p20,1); 00020 //Measure pin 20 00021 adc.select(p20); 00022 00023 // Vars 00024 00025 int count; 00026 // Program 00027 00028 // AD conversion 00029 for(count = 0; count < LENGTH_RESULT; count++){ 00030 //Start ADC conversion 00031 adc.start(); 00032 //Wait for it to complete 00033 while(!adc.done(p20)); 00034 result[count] = adc.read(p20); 00035 } 00036 // Send over UART 00037 for(count = 0; count < LENGTH_RESULT; count++){ 00038 uart.printf("%04u.\n", result[count]); 00039 } 00040 } 00041 00042 00043
Generated on Fri Jul 15 2022 03:08:06 by
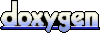