Source code for Maxim Sensor Hub Communications. Mostly C library for MAX32664 sensor hub communications.
Embed:
(wiki syntax)
Show/hide line numbers
SHComm.h
00001 /******************************************************************************* 00002 * Copyright (C) 2018 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 00034 #ifndef SOURCE_SHCOMM_H_ 00035 #define SOURCE_SHCOMM_H_ 00036 00037 /* 00038 #ifdef __cplusplus 00039 extern "C" { 00040 #endif 00041 */ 00042 00043 // Sensor/Algo indicies 00044 #define SH_SENSORIDX_MAX8614X 0x00 00045 #define SH_SENSORIDX_MAX30205 0x01 00046 #define SH_SENSORIDX_MAX30001 0x02 00047 #define SH_SENSORIDX_MAX30101 0x03 00048 #define SH_SENSORIDX_ACCEL 0x04 00049 #define SH_NUM_CURRENT_SENSORS 5 00050 00051 #define SH_ALGOIDX_AGC 0x00 00052 #define SH_ALGOIDX_AEC 0x01 00053 #define SH_ALGOIDX_WHRM 0x02 00054 #define SH_ALGOIDX_ECG 0x03 00055 #define SH_ALGOIDX_BPT 0x04 00056 #define SH_ALGOIDX_WSPO2 0x05 00057 #define SH_NUM_CURRENT_ALGOS 6 00058 00059 #define PADDING_BYTE (0xEE) 00060 #define DATA_BYTE (0xED) 00061 00062 00063 #define SS_I2C_8BIT_SLAVE_ADDR 0xAA 00064 #define SS_DEFAULT_CMD_SLEEP_MS 2 00065 #define SS_DUMP_REG_SLEEP_MS 100 00066 #define SS_ENABLE_SENSOR_SLEEP_MS 20 00067 00068 #define SH_INPUT_DATA_DIRECT_SENSOR 0x00 00069 #define SH_INPUT_DATA_FROM_HOST 0x01 00070 00071 #define SS_FAM_R_STATUS 0x00 00072 #define SS_CMDIDX_STATUS 0x00 00073 #define SS_SHIFT_STATUS_ERR 0 00074 #define SS_MASK_STATUS_ERR (0x07 << SS_SHIFT_STATUS_ERR) 00075 #define SS_SHIFT_STATUS_DATA_RDY 3 00076 #define SS_MASK_STATUS_DATA_RDY (1 << SS_SHIFT_STATUS_DATA_RDY) 00077 #define SS_SHIFT_STATUS_FIFO_OUT_OVR 4 00078 #define SS_MASK_STATUS_FIFO_OUT_OVR (1 << SS_SHIFT_STATUS_FIFO_OUT_OVR) 00079 #define SS_SHIFT_STATUS_FIFO_IN_OVR 5 00080 #define SS_MASK_STATUS_FIFO_IN_OVR (1 << SS_SHIFT_STATUS_FIFO_IN_OVR) 00081 00082 #define SS_SHIFT_STATUS_LOG_OVR 6 00083 #define SS_MASK_STATUS_LOG_OVR (1 << SS_SHIFT_STATUS_LOG_OVR) 00084 00085 #define SS_SHIFT_STATUS_LOG_RDY 7 00086 #define SS_MASK_STATUS_LOG_RDY (1 << SS_SHIFT_STATUS_LOG_RDY) 00087 00088 00089 00090 #define SS_FAM_W_MODE 0x01 00091 #define SS_FAM_R_MODE 0x02 00092 #define SS_CMDIDX_MODE 0x00 00093 #define SS_SHIFT_MODE_SHDN 0 00094 #define SS_MASK_MODE_SHDN (1 << SS_SHIFT_MODE_SHDN) 00095 #define SS_SHIFT_MODE_RESET 1 00096 #define SS_MASK_MODE_RESET (1 << SS_SHIFT_MODE_RESET) 00097 #define SS_SHIFT_MODE_FIFORESET 2 00098 #define SS_MASK_MODE_FIFORESET (1 << SS_SHIFT_MODE_FIFORESET) 00099 #define SS_SHIFT_MODE_BOOTLDR 3 00100 #define SS_MASK_MODE_BOOTLDR (1 << SS_SHIFT_MODE_BOOTLDR) 00101 00102 /*MYG*/ 00103 #define SH_MODE_REQUEST_RET_BYTES (2) 00104 #define SH_MODE_REQUEST_DELAY (2) 00105 #define SH_STATUS_REQUEST_RET_BYTES (2) 00106 #define SH_STATUS_REQUEST_DELAY (2) 00107 00108 00109 00110 #define SS_I2C_READ 0x03 00111 00112 #define SS_FAM_W_COMMCHAN 0x10 00113 #define SS_FAM_R_COMMCHAN 0x11 00114 #define SS_CMDIDX_OUTPUTMODE 0x00 00115 #define SS_SHIFT_OUTPUTMODE_DATATYPE 0 00116 #define SS_MASK_OUTPUTMODE_DATATYPE (0x03 << SS_SHIFT_OUTPUTMODE_DATATYPE) 00117 #define SS_DATATYPE_PAUSE 0 00118 #define SS_DATATYPE_RAW 1 00119 #define SS_DATATYPE_ALGO 2 00120 #define SS_DATATYPE_BOTH 3 00121 #define SS_SHIFT_OUTPUTMODE_SC_EN 2 00122 #define SS_MASK_OUTPUTMODE_SC_EN (1 << SS_SHIFT_OUTPUTMODE_SC_EN) 00123 #define SS_CMDIDX_FIFOAFULL 0x01 00124 00125 #define SS_FAM_R_OUTPUTFIFO 0x12 00126 #define SS_CMDIDX_OUT_NUMSAMPLES 0x00 00127 #define SS_CMDIDX_READFIFO 0x01 00128 00129 #define SS_FAM_R_INPUTFIFO 0x13 00130 #define SS_CMDIDX_SAMPLE_SIZE 0x00 00131 #define SS_CMDIDX_INPUT_FIFO_SIZE 0x01 00132 #define SS_CMDIDX_SENSOR_FIFO_SIZE 0x02 00133 #define SS_CMDIDX_NUM_SAMPLES_SENSOR_FIFO 0x03 00134 #define SS_CMDIDX_NUM_SAMPLES_INPUT_FIFO 0x04 00135 00136 #define SS_FAM_W_INPUTFIFO 0x14 00137 #define SS_CMDIDN_WRITEFIFO 0x00 00138 #define SS_CMDIDX_WRITE_FIFO 0x00 00139 00140 #define SS_FAM_W_WRITEREG 0x40 00141 #define SS_FAM_R_READREG 0x41 00142 #define SS_FAM_R_REGATTRIBS 0x42 00143 #define SS_FAM_R_DUMPREG 0x43 00144 00145 #define SS_FAM_W_SENSORMODE 0x44 00146 #define SS_FAM_R_SENSORMODE 0x45 00147 00148 //TODO: Fill in known configuration parameters 00149 #define SS_FAM_W_ALGOCONFIG 0x50 00150 #define SS_FAM_R_ALGOCONFIG 0x51 00151 #define SS_CFGIDX_AGC_TARGET 0x00 00152 #define SS_CFGIDX_AGC_CORR_COEFF 0x01 00153 #define SS_CFGIDX_AGC_SENSITIVITY 0x02 00154 #define SS_CFGIDX_AGC_SMP_AVG 0x03 00155 00156 #define SS_CFGIDX_WHRM_SR 0x00 00157 #define SS_CFGIDX_WHRM_MAX_HEIGHT 0x01 00158 #define SS_CFGIDX_WHRM_MAX_WEIGHT 0x02 00159 #define SS_CFGIDX_WHRM_MAX_AGE 0x03 00160 #define SS_CFGIDX_WHRM_MIN_HEIGHT 0x04 00161 #define SS_CFGIDX_WHRM_MIN_WEIGHT 0x05 00162 #define SS_CFGIDX_WHRM_MIN_AGE 0x06 00163 #define SS_CFGIDX_WHRM_DEF_HEIGHT 0x07 00164 #define SS_CFGIDX_WHRM_DEF_WEIGHT 0x08 00165 #define SS_CFGIDX_WHRM_DEF_AGE 0x09 00166 #define SS_CFGIDX_WHRM_INIT_HR 0x0A 00167 00168 #define SS_CFGIDX_BP_USE_MED 0x00 00169 #define SS_CFGIDX_BP_SYS_BP_CAL 0x01 00170 #define SS_CFGIDX_BP_DIA_BP_CAL 0x02 00171 #define SS_CFGIDX_BP_CAL_DATA 0x03 00172 #define SS_CFGIDX_BP_EST_DATE 0x04 00173 #define SS_CFGIDX_BP_EST_NONREST 0x05 00174 00175 #define SS_FAM_W_ALGOMODE 0x52 00176 #define SS_FAM_R_ALGOMODE 0x53 00177 00178 #define SS_FAM_W_EXTERNSENSORMODE 0x60 00179 #define SS_FAM_R_EXTERNSENSORMODE 0x61 00180 00181 #define SS_FAM_R_SELFTEST 0x70 00182 00183 #define SS_FAM_W_BOOTLOADER 0x80 00184 #define SS_CMDIDX_SETIV 0x00 00185 #define SS_CMDIDX_SETAUTH 0x01 00186 #define SS_CMDIDX_SETNUMPAGES 0x02 00187 #define SS_CMDIDX_ERASE 0x03 00188 #define SS_CMDIDX_SENDPAGE 0x04 00189 #define SS_CMDIDX_ERASE_PAGE 0x05 00190 #define SS_FAM_R_BOOTLOADER 0x81 00191 #define SS_CMDIDX_BOOTFWVERSION 0x00 00192 #define SS_CMDIDX_PAGESIZE 0x01 00193 00194 #define SS_FAM_W_BOOTLOADER_CFG 0x82 00195 #define SS_FAM_R_BOOTLOADER_CFG 0x83 00196 #define SS_CMDIDX_BL_SAVE 0x00 00197 #define SS_CMDIDX_BL_ENTRY 0x01 00198 #define SS_BL_CFG_ENTER_BL_MODE 0x00 00199 #define SS_BL_CFG_EBL_PIN 0x01 00200 #define SS_BL_CFG_EBL_POL 0x02 00201 #define SS_CMDIDX_BL_EXIT 0x02 00202 #define SS_BL_CFG_EXIT_BL_MODE 0x00 00203 #define SS_BL_CFG_TIMEOUT 0x01 00204 00205 /* Enable logging/debugging */ 00206 #define SS_FAM_R_LOG 0x90 00207 #define SS_CMDIDX_R_LOG_DATA 0x00 00208 #define SS_CMDIDX_R_LOG_LEN 0x01 00209 00210 #define SS_CMDIDX_R_LOG_LEVEL 0x02 00211 #define SS_LOG_DISABLE 0x00 00212 #define SS_LOG_CRITICAL 0x01 00213 #define SS_LOG_ERROR 0x02 00214 #define SS_LOG_INFO 0x04 00215 #define SS_LOG_DEBUG 0x08 00216 00217 #define SS_FAM_W_LOG_CFG 0x91 00218 #define SS_CMDIDX_LOG_GET_LEVEL 0x00 00219 #define SS_CMDIDX_LOG_SET_LEVEL 0x01 00220 00221 #define SS_FAM_R_IDENTITY 0xFF 00222 #define SS_CMDIDX_PLATTYPE 0x00 00223 #define SS_CMDIDX_PARTID 0x01 00224 #define SS_CMDIDX_REVID 0x02 00225 #define SS_CMDIDX_FWVERSION 0x03 00226 #define SS_CMDIDX_AVAILSENSORS 0x04 00227 #define SS_CMDIDX_DRIVERVER 0x05 00228 #define SS_CMDIDX_AVAILALGOS 0x06 00229 #define SS_CMDIDX_ALGOVER 0x07 00230 00231 00232 /* Newly added ones; checko for collosion or repeats with the ones above */ 00233 #define SS_RESET_TIME 10 00234 #define SS_STARTUP_TO_BTLDR_TIME 20 00235 #define SS_STARTUP_TO_MAIN_APP_TIME 1000 00236 00237 #define SS_MAX_SUPPORTED_SENSOR_NUM 0xFE 00238 #define SS_MAX_SUPPORTED_ALGO_NUM 0xFE 00239 00240 #define SS_APPPLICATION_MODE 0x00 00241 #define SS_BOOTLOADER_MODE 0x08 00242 00243 typedef enum { 00244 SS_SUCCESS =0x00, 00245 SS_ERR_COMMAND =0x01, 00246 SS_ERR_UNAVAILABLE =0x02, 00247 SS_ERR_DATA_FORMAT =0x03, 00248 SS_ERR_INPUT_VALUE =0x04, 00249 SS_ERR_BTLDR_GENERAL =0x80, 00250 SS_ERR_BTLDR_CHECKSUM =0x81, 00251 SS_ERR_TRY_AGAIN =0xFE, 00252 SS_ERR_UNKNOWN =0xFF, 00253 00254 } SS_STATUS; 00255 00256 00257 00258 /* ***************************************************************************************** * 00259 * * 00260 * SENSOR HUB COMMUNICATION INTERFACE ( Defined in MAX32664 User Guide ) API FUNCTIONS * 00261 * * 00262 * * 00263 * ***************************************************************************************** */ 00264 00265 00266 /** 00267 * @brief Func to write to sensor hub via sending generic command byte sequences 00268 * 00269 * @param[in] tx_buf - command byte sequence 00270 * @param[in] tx_len - command byte sequence length in bytes 00271 * @param[in] sleep_ms - time to wait for sensor hub to report statuss 00272 * 00273 * @return 1 byte status: 0x00 (SS_SUCCESS) on success 00274 */ 00275 int sh_write_cmd( uint8_t *tx_buf, 00276 int tx_len, 00277 int sleep_ms ); 00278 00279 00280 /** 00281 * @brief Func to write to sensor hub via sending generic command byte sequences and data bytes 00282 * 00283 * @param[in] cmd_bytes - command byte sequence 00284 * @param[in] cmd_bytes_len - command byte sequence length in bytes 00285 * @param[in] data - data byte array to be sent following cmd bytes 00286 * @param[in] data_len - data array size in bytes 00287 * @param[in] cmd_delay_ms - time to wait for sensor hub to report status 00288 * 00289 * @return 1 byte status: 0x00 (SS_SUCCESS) on success 00290 */ 00291 int sh_write_cmd_with_data(uint8_t *cmd_bytes, 00292 int cmd_bytes_len, 00293 uint8_t *data, 00294 int data_len, 00295 int cmd_delay_ms); 00296 00297 00298 /** 00299 * @brief Func to read from sensor hub via sending generic command byte sequences 00300 * 00301 * @param[in] cmd_bytes - command byte sequence 00302 * @param[in] cmd_bytes_len - command byte sequence length in bytes 00303 * @param[in] data - data byte array to be sent following cmd bytes 00304 * @param[in] data_len - data array size in bytes 00305 * @param[out] rxbuf - byte buffer to store incoming data (including status byte) 00306 * @param[in] rxbuf_sz - incoming data buffer size in bytes ( to prevent overflow) 00307 * @param[in] cmd_delay_ms - time to wait for sensor hub to report status 00308 * 00309 * @return 1 byte status: 0x00 (SS_SUCCESS) on success 00310 */ 00311 int sh_read_cmd( uint8_t *cmd_bytes, 00312 int cmd_bytes_len, 00313 uint8_t *data, 00314 int data_len, 00315 uint8_t *rxbuf, 00316 int rxbuf_sz, 00317 int sleep_ms ); 00318 00319 00320 /** 00321 * @brief func to read sensor hub status 00322 * @param[out] hubStatus - pointer to output byte sesnor hub status will be written 00323 * @details ensor hub status byte: [2:0] -> 0 : no Err , 1: comm failure with sensor 00324 * [3] -> 0 : FIFO below threshold; 1: FIFO filled to threshold or above. 00325 * [4] -> 0 : No FIFO overflow; 1: Sensor Hub Output FIFO overflowed, data lost. 00326 * [5] -> 0 : No FIFO overflow; 1: Sensor Hub Input FIFO overflowed, data lost. 00327 * [6] -> 0 : Sensor Hub ready; 1: Sensor Hub is busy processing. 00328 * [6] -> reserved. 00329 * 00330 * @return 1 byte status: 0x00 (SS_SUCCESS) on success 00331 */ 00332 int sh_get_sensorhub_status(uint8_t *hubStatus); 00333 00334 00335 /** 00336 * @brief func to read sensor operating mode 00337 * 00338 * @param[in] hubMode - pointer to output byte mode will be written 00339 * @details 0x00: application operating mode 00340 * 0x08: bootloader operating mode 00341 * 00342 * @return 1 byte status: 0x00 (SS_SUCCESS) on success 00343 */ 00344 int sh_get_sensorhub_operating_mode(uint8_t *hubMode); 00345 00346 00347 /** 00348 * @brief func to set sensor hub operating mode 00349 * 00350 * @param[out] hubMode - pointer to output byte mode will be written 00351 * @details 0x00: application operating mode 00352 * 0x02: soft reset 00353 * 0x08: bootloader operating mode 00354 * 00355 * @return 1 byte status: 0x00 (SS_SUCCESS) on success 00356 */ 00357 int sh_set_sensorhub_operating_mode(uint8_t hubMode); 00358 00359 00360 /** 00361 * @brief func to set sensorhub data output mode 00362 * 00363 * @param[in] data_type : 1 byte output format 00364 * @details outpur format 0x00 : no data 00365 * 0x01 : sensor data SS_DATATYPE_RAW 00366 * 0x02 : algo data SS_DATATYPE_ALGO 00367 * 0x03 : algo+sensor SS_DATATYPE_BOTH 00368 * 00369 * @return 1 byte status: 0x00 (SS_SUCCESS) on success 00370 */ 00371 int sh_set_data_type(int data_type, bool sc_en); 00372 00373 00374 /** 00375 * @brief func to get sensorhub data output mode 00376 * 00377 * @param[out] data_type - pointer to byte, output format will be written to. 00378 * 00379 * @param[out] sc_en - pointer to boolean, sample count enable/disable status format will be written to. 00380 * If true, SmartSensor is prepending data with 1 byte sample count. 00381 * 00382 * @details output format 0x00 : only algorithm data 00383 * 0x01 : only raw sensor data 00384 * 0x02 : algo + raw sensor data 00385 * 0x03 : no data 00386 * 00387 * @return 1 byte status: 0x00 (SS_SUCCESS) on success 00388 */ 00389 int sh_get_data_type(int *data_type, bool *sc_en); 00390 00391 00392 /** 00393 * @brief func to set the number of samples for the SmartSensor to collect 00394 * before issuing an mfio event reporting interrupt 00395 * 00396 * @param[in] thresh - Number of samples (1-255) to collect before interrupt 00397 * 00398 * @return 1 byte status (SS_STATUS) : 0x00 (SS_SUCCESS) on success 00399 */ 00400 int sh_set_fifo_thresh( int threshold ); 00401 00402 00403 /** 00404 * @brief func to get the number of samples the SmartSensor will collect 00405 * before issuing an mfio event reporting interrupt 00406 * 00407 * @param[out] thresh - Number of samples (1-255) collected before interrupt 00408 * 00409 * @return 1 byte status (SS_STATUS) : 0x00 (SS_SUCCESS) on success 00410 */ 00411 int sh_get_fifo_thresh(int *thresh); 00412 00413 00414 /** 00415 * @brief func to check that the SmartSensor is connected 00416 * 00417 * @return 1 byte connection status 0x00: on connection 00418 */ 00419 int sh_ss_comm_check(void); 00420 00421 00422 /** 00423 * @brief func to get the number of available samples in SmartSensor output FIFO 00424 * 00425 * @param[out] numSamples - number of data struct samples (1-255) 00426 * 00427 * @return 1 byte status: 0x00 (SS_SUCCESS) on success 00428 */ 00429 int sh_num_avail_samples(int *numSamples); 00430 00431 00432 /** 00433 * @brief func to pull samples from SmartSensor output FIFO 00434 * 00435 * @param[in] numSamples - number of data struct samples to be pulled 00436 * @param[in] sampleSize - size of cumulative data sample struct (based on enabled sesnors+algorithms) in bytes 00437 * @param[out] databuf - buffer samples be written 00438 * @param[in] databufSize - size of provided buffer size samples to be written 00439 * 00440 * @return 1 byte status: 0x00 (SS_SUCCESS) on success 00441 */ 00442 int sh_read_fifo_data( int numSamples, int sampleSize, uint8_t* databuf, int databufSz); 00443 00444 00445 /** 00446 * @brief func to set register of a device onboard SmartSensor 00447 * 00448 * @param[in] idx - Index of device to read 00449 * @param[in] addr - Register address 00450 * @param[in] val - Register value 00451 * @param[in] regSz - Size of sensor device register in bytes 00452 * 00453 * @return 1 byte status (SS_STATUS) : 0x00 (SS_SUCCESS) on success 00454 */ 00455 int sh_set_reg(int idx, uint8_t addr, uint32_t val, int regSz); 00456 00457 00458 /** 00459 * @brief func to read register from a device onboard SmartSensor 00460 * 00461 * @param[in] idx - Index of device to read 00462 * @param[in] addr - Register address 00463 * @param[out] val - Register value 00464 * 00465 * @return 1 byte status (SS_STATUS) : 0x00 (SS_SUCCESS) on success 00466 */ 00467 int sh_get_reg(int idx, uint8_t addr, uint32_t *val); 00468 00469 00470 // depricated: int sh_sensor_enable( int idx , int sensorSampleSz); 00471 /** 00472 * @brief func to enable a sensor device onboard SmartSensor 00473 * 00474 * @param[in] idx - index of sensor device( i.e max8614x) to enable 00475 * @param[in] sensorSampleSz - sample size of sensor device( i.e max8614x) to enable 00476 * @param[in] ext_mode - enable extermal data input to Sensot Hub, ie accelerometer data for WHRM+WSPo2 00477 * 00478 * @return 1 byte status (SS_STATUS) : 0x00 (SS_SUCCESS) on success 00479 */ 00480 int sh_sensor_enable( int idx , int sensorSampleSz , uint8_t ext_mode ); 00481 00482 00483 /** 00484 * @brief func to disable a device on the SmartSensor 00485 * 00486 * @param[in] idx - Index of device 00487 * 00488 * @return 1 byte status (SS_STATUS) : 0x00 (SS_SUCCESS) on success 00489 */ 00490 int sh_sensor_disable( int idx ); 00491 00492 00493 /** 00494 * @brief func to get the total number of samples the input FIFO can hold 00495 * 00496 * @param[in] fifo_size - intger input FIFO capacity will be written to. 00497 * 00498 * @return 1 byte status (SS_STATUS) : 0x00 (SS_SUCCESS) on success 00499 */ 00500 int sh_get_input_fifo_size(int *fifo_size); 00501 00502 00503 /** 00504 * @brief func to send ass external sensor data (accelerometer) to sensor hub's input FIFO 00505 * 00506 * @param[in] tx_buf - host sample data to be send to sensor hub input FIFO 00507 * @param[in] tx_buf_sz - number of bytes of tx_buf 00508 * @param[out] nb_written - number of samples succesfully written to sensor hub's input FIFO 00509 * 00510 * @return 1 byte status (SS_STATUS) : 0x00 (SS_SUCCESS) on success 00511 */ 00512 int sh_feed_to_input_fifo(uint8_t *tx_buf, int tx_buf_sz, int *nb_written); 00513 00514 00515 /** 00516 * @brief func to get the total number of bytes in the sensor hub's input FIFO 00517 * 00518 * @param[in] fifo_size - total number of sample bytes available in input FIFO 00519 * 00520 * @return 1 byte status (SS_STATUS) : 0x00 (SS_SUCCESS) on success 00521 */ 00522 int sh_get_num_bytes_in_input_fifo(int *fifo_size); 00523 00524 00525 /** 00526 * @brief func to enable an algorithm on SmartSensor 00527 * 00528 * @param[in] idx - index of algorithm to enable 00529 * @param[in] sensorSampleSz - sample size of algorithm to enable 00530 * 00531 * @details idx - 0x00 : AGC 00532 * 0x01 : AEC 00533 * 0x02 : WHRM/Maximfast 00534 * 0x03 : ECG 00535 * 0x04 : BPT 00536 * 0x05 : SPo2 00537 * 0x06 : HRM/Maximfast finger 00538 * 00539 * @return 1 byte status (SS_STATUS) : 0x00 (SS_SUCCESS) on success 00540 */ 00541 int sh_enable_algo(int idx , int algoSampleSz); 00542 00543 00544 /** 00545 * @brief func to disable an algorithm on the SmartSensor 00546 * 00547 * @param[in] idx - index of algorithm to disable 00548 * 00549 * @return 1 byte status (SS_STATUS) : 0x00 (SS_SUCCESS) on success 00550 */ 00551 int sh_disable_algo(int idx); 00552 00553 00554 /** 00555 * @brief func to set the value of an algorithm configuration parameter 00556 * 00557 * @param[in] algo_idx - index of algorithm 00558 * @param[in] cfg_idx - index of configuration parameter 00559 * @param[in] cfg Array - byte array of configuration 00560 * @param[in] cfg_sz - size of cfg array 00561 * 00562 * @return 1 byte status (SS_STATUS) : 0x00 (SS_SUCCESS) on success 00563 */ 00564 int sh_set_algo_cfg(int algo_idx, int cfg_idx, uint8_t *cfg, int cfg_sz); 00565 00566 00567 /** 00568 * @brief func to get the value of an algorithm configuration parameter 00569 * 00570 * @param[in] algo_idx - index of algorithm 00571 * @param[in] cfg_idx - index of configuration parameter 00572 * @param[out] cfg - array of configuration bytes to be filled in 00573 * @param[in] cfg_sz - number of configuration parameter bytes to be read 00574 * 00575 * @return 1 byte status (SS_STATUS) : 0x00 (SS_SUCCESS) on success 00576 */ 00577 int sh_get_algo_cfg(int algo_idx, int cfg_idx, uint8_t *cfg, int cfg_sz); 00578 00579 /** 00580 * @brief func to pull sensor, algo data sample bytes from sensor hub. outpur buffer, Content of the buffer depends on 00581 * enabled sensors, algorithms and their sample sizes. 00582 * 00583 * @param[out] databuf - byte buffer to hold pulled samples 00584 * @param[in] databufLen - size of provided databuf in bytes 00585 * @param[out] nSamplesRea - number of pulled samples in databuf 00586 * 00587 * @return N/A 00588 */ 00589 void sh_ss_execute_once( uint8_t *databuf , int databufLen , int *nSamplesRead); 00590 00591 00592 00593 00594 00595 00596 00597 /* *************************************************************************************** * 00598 * DEMO SPECIFIC DECLERATIONS, NOT RELATED TO SENSOR HUB INTERFACE API. * 00599 * * 00600 * * * 00601 ******************************************************************************************/ 00602 00603 void sh_init_hwcomm_interface(); 00604 bool sh_has_mfio_event(void); 00605 void sh_enable_irq_mfioevent(void); 00606 void sh_disable_irq_mfioevent(void); 00607 void sh_clear_mfio_event_flag(void); 00608 int sh_hard_reset(int wakeupMode); 00609 00610 extern uint8_t sh_write_buf[]; 00611 00612 /* 00613 #ifdef __cplusplus 00614 } 00615 #endif 00616 */ 00617 00618 00619 00620 #endif /* _SENSOR_HUB_H */ 00621 00622 00623 00624 00625
Generated on Wed Jul 13 2022 21:47:33 by
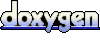