Small bitmap-library to use with monocrome displays.
Embed:
(wiki syntax)
Show/hide line numbers
TinyBitmap.cpp
00001 #define M_PI 3.14159265358979323846 //Pi 00002 00003 #include "mbed.h" 00004 #include <math.h> 00005 #include "TinyBitmap.h" 00006 00007 TinyBitmap::TinyBitmap(int width, int height, char *framebuffer, int commandbytes, int bitmaplayout) //Constructor 00008 { 00009 _IMAGEWIDTH = width; 00010 _IMAGEHEIGHT = height; 00011 _COMMANDBYTES = commandbytes; 00012 _BITMAPLAYOUT = bitmaplayout; 00013 _FRAMEBUFFER = framebuffer; 00014 00015 Bit0 = 0x01; 00016 Bit1 = 0x02; 00017 Bit2 = 0x04; 00018 Bit3 = 0x08; 00019 Bit4 = 0x10; 00020 Bit5 = 0x20; 00021 Bit6 = 0x40; 00022 Bit7 = 0x80; 00023 } 00024 00025 void TinyBitmap::DrawPixel(int x, int y) 00026 { 00027 bool InsideBorders = true; 00028 if (x < 0 | y < 0 | x > _IMAGEWIDTH - 1 | y > _IMAGEHEIGHT - 1) InsideBorders = false; 00029 00030 if(_BITMAPLAYOUT == 0x00 && InsideBorders) { 00031 int pixelnumber = (y * _IMAGEWIDTH) + x; //get the 1D pixel-position 00032 int bytecount = pixelnumber / 8; //the byte-number 00033 int offset = pixelnumber % 8; //the bit inside the byte 00034 char modifier = 0; 00035 00036 if (offset == 0) modifier = Bit7; 00037 if (offset == 1) modifier = Bit6; 00038 if (offset == 2) modifier = Bit5; 00039 if (offset == 3) modifier = Bit4; 00040 if (offset == 4) modifier = Bit3; 00041 if (offset == 5) modifier = Bit2; 00042 if (offset == 6) modifier = Bit1; 00043 if (offset == 7) modifier = Bit0; 00044 00045 _FRAMEBUFFER[bytecount + _COMMANDBYTES] = (char)(_FRAMEBUFFER[bytecount + _COMMANDBYTES] | modifier); 00046 } 00047 00048 if (_BITMAPLAYOUT == 0x02 && InsideBorders) 00049 { 00050 int MemPage = y / 8; 00051 int bytecount = x + (_IMAGEWIDTH * MemPage); //the byte-number 00052 int offset = y % 8; //the bit inside the byte 00053 //int pixelnumber = (bytecount * 8) + offset; //get the 1D pixel-position 00054 char modifier = 0; 00055 00056 if (offset == 7) modifier = Bit7; 00057 if (offset == 6) modifier = Bit6; 00058 if (offset == 5) modifier = Bit5; 00059 if (offset == 4) modifier = Bit4; 00060 if (offset == 3) modifier = Bit3; 00061 if (offset == 2) modifier = Bit2; 00062 if (offset == 1) modifier = Bit1; 00063 if (offset == 0) modifier = Bit0; 00064 00065 _FRAMEBUFFER[bytecount + _COMMANDBYTES] = (char)(_FRAMEBUFFER[bytecount + _COMMANDBYTES] | modifier); 00066 } 00067 00068 if (_BITMAPLAYOUT == 0x04 && InsideBorders) 00069 { 00070 int pixelnumber = (y * _IMAGEWIDTH) + x; //get the 1D pixel-position 00071 int bytecount = pixelnumber / 8; //the byte-number 00072 int offset = pixelnumber % 8; //the bit inside the byte 00073 char modifier = 0; 00074 00075 if (offset == 7) modifier = Bit7; 00076 if (offset == 6) modifier = Bit6; 00077 if (offset == 5) modifier = Bit5; 00078 if (offset == 4) modifier = Bit4; 00079 if (offset == 3) modifier = Bit3; 00080 if (offset == 2) modifier = Bit2; 00081 if (offset == 1) modifier = Bit1; 00082 if (offset == 0) modifier = Bit0; 00083 00084 _FRAMEBUFFER[bytecount + _COMMANDBYTES] = (char)(_FRAMEBUFFER[bytecount + _COMMANDBYTES] | modifier); 00085 } 00086 00087 } 00088 00089 void TinyBitmap::DrawLine(int x1, int y1, int x2, int y2) 00090 { 00091 float xdiff_abs = abs(x2 - x1); 00092 float ydiff_abs = abs(y2 - y1); 00093 00094 float steps; 00095 if (xdiff_abs >= ydiff_abs) steps = xdiff_abs; 00096 else steps = ydiff_abs; 00097 00098 for (float i = 0; i <= (int)steps; i++) 00099 { 00100 float corrector = 0; 00101 if (i == 0 && steps == 0) { 00102 corrector = 1; 00103 } 00104 //X-Values: 00105 float pX = x1 + ((x2 - x1) / (steps + corrector) * i); 00106 //Y-Values: 00107 float pY = y1 + ((y2 - y1) / (steps + corrector) * i); 00108 DrawPixel((int)pX, (int)pY); 00109 } 00110 } 00111 00112 void TinyBitmap::DrawCircle(int x, int y, int radius) 00113 { 00114 if(radius < 1) radius = 1; 00115 00116 for (float rad = 0; rad < M_PI * 2 ; rad += (float)(5 / (2 * M_PI * radius))) 00117 { 00118 DrawPixel(x + (int)(radius * cos(rad)), y + (int)(radius * sin(rad))); 00119 } 00120 } 00121 00122 void TinyBitmap::DrawRectangle(int x1, int y1, int x2, int y2) 00123 { 00124 DrawLine(x1, y1, x1, y2); 00125 DrawLine(x1, y1, x2, y1); 00126 DrawLine(x2, y1, x2, y2); 00127 DrawLine(x2, y2, x1, y2); 00128 } 00129 00130 void TinyBitmap::DrawLineDirection(int x, int y, int degree, int lenght) 00131 { 00132 float rad = degree * (float)M_PI / 180; 00133 for (int radius = 0; radius <= lenght; radius++) 00134 { 00135 DrawPixel(x + (int)(radius * cos(rad)), y + (int)(radius * sin(rad))); 00136 } 00137 } 00138 00139 00140 //Textrendering 00141 void TinyBitmap::DrawText(int x, int y, char *CharArray, bool UseLargeFont) //removed: string text 00142 { 00143 00144 const char Letter_A[] = { 0x41, 0x05, 0x0E, 0x11, 0x11, 0x11, 0x1F, 0x11, 0x11, 0x00 }; 00145 const char Letter_B[] = { 0x42, 0x05, 0x0F, 0x11, 0x11, 0x0F, 0x11, 0x11, 0x0F, 0x00 }; 00146 const char Letter_C[] = { 0x43, 0x05, 0x0E, 0x11, 0x01, 0x01, 0x01, 0x11, 0x0E, 0x00 }; 00147 const char Letter_D[] = { 0x44, 0x05, 0x07, 0x09, 0x11, 0x11, 0x11, 0x09, 0x07, 0x00 }; 00148 const char Letter_E[] = { 0x45, 0x05, 0x1F, 0x01, 0x01, 0x0F, 0x01, 0x01, 0x1F, 0x00 }; 00149 const char Letter_F[] = { 0x46, 0x05, 0x1F, 0x01, 0x01, 0x0F, 0x01, 0x01, 0x01, 0x00 }; 00150 const char Letter_G[] = { 0x47, 0x05, 0x0E, 0x11, 0x01, 0x1D, 0x11, 0x11, 0x1E, 0x00 }; 00151 const char Letter_H[] = { 0x48, 0x05, 0x11, 0x11, 0x11, 0x1F, 0x11, 0x11, 0x11, 0x00 }; 00152 const char Letter_I[] = { 0x49, 0x05, 0x0E, 0x04, 0x04, 0x04, 0x04, 0x04, 0x0E, 0x00 }; 00153 const char Letter_J[] = { 0x4A, 0x05, 0x1C, 0x08, 0x08, 0x08, 0x08, 0x09, 0x06, 0x00 }; 00154 const char Letter_K[] = { 0x4B, 0x05, 0x11, 0x09, 0x05, 0x03, 0x05, 0x09, 0x11, 0x00 }; 00155 const char Letter_L[] = { 0x4C, 0x05, 0x01, 0x01, 0x01, 0x01, 0x01, 0x01, 0x1F, 0x00 }; 00156 const char Letter_M[] = { 0x4D, 0x05, 0x11, 0x1B, 0x15, 0x15, 0x11, 0x11, 0x11, 0x00 }; 00157 const char Letter_N[] = { 0x4E, 0x05, 0x11, 0x11, 0x13, 0x15, 0x19, 0x11, 0x11, 0x00 }; 00158 const char Letter_O[] = { 0x4F, 0x05, 0x0E, 0x11, 0x11, 0x11, 0x11, 0x11, 0x0E, 0x00 }; 00159 const char Letter_P[] = { 0x50, 0x05, 0x0F, 0x11, 0x11, 0x0F, 0x01, 0x01, 0x01, 0x00 }; 00160 const char Letter_Q[] = { 0x51, 0x05, 0x0E, 0x11, 0x11, 0x11, 0x15, 0x09, 0x16, 0x00 }; 00161 const char Letter_R[] = { 0x52, 0x05, 0x0F, 0x11, 0x11, 0x0F, 0x05, 0x09, 0x11, 0x00 }; 00162 const char Letter_S[] = { 0x53, 0x05, 0x1E, 0x01, 0x01, 0x0E, 0x10, 0x10, 0x0F, 0x00 }; 00163 const char Letter_T[] = { 0x54, 0x05, 0x1F, 0x04, 0x04, 0x04, 0x04, 0x04, 0x04, 0x00 }; 00164 const char Letter_U[] = { 0x55, 0x05, 0x11, 0x11, 0x11, 0x11, 0x11, 0x11, 0x0E, 0x00 }; 00165 const char Letter_V[] = { 0x56, 0x05, 0x11, 0x11, 0x11, 0x11, 0x11, 0x0A, 0x04, 0x00 }; 00166 const char Letter_W[] = { 0x57, 0x05, 0x11, 0x11, 0x11, 0x15, 0x15, 0x15, 0x0A, 0x00 }; 00167 const char Letter_X[] = { 0x58, 0x05, 0x11, 0x11, 0x0A, 0x04, 0x0A, 0x11, 0x11, 0x00 }; 00168 const char Letter_Y[] = { 0x59, 0x05, 0x11, 0x11, 0x0A, 0x04, 0x04, 0x04, 0x04, 0x00 }; 00169 const char Letter_Z[] = { 0x5A, 0x05, 0x1F, 0x10, 0x08, 0x04, 0x02, 0x01, 0x1F, 0x00 }; 00170 00171 const char Letter_a[] = { 0x61, 0x05, 0x00, 0x00, 0x0E, 0x10, 0x1E, 0x11, 0x1E, 0x00 }; 00172 const char Letter_b[] = { 0x62, 0x05, 0x01, 0x01, 0x01, 0x0F, 0x11, 0x11, 0x0F, 0x00 }; 00173 const char Letter_c[] = { 0x63, 0x05, 0x00, 0x00, 0x1E, 0x01, 0x01, 0x01, 0x1E, 0x00 }; 00174 const char Letter_d[] = { 0x64, 0x05, 0x10, 0x10, 0x10, 0x1E, 0x11, 0x11, 0x1E, 0x00 }; 00175 const char Letter_e[] = { 0x65, 0x05, 0x00, 0x00, 0x0E, 0x11, 0x1F, 0x01, 0x1E, 0x00 }; 00176 const char Letter_f[] = { 0x66, 0x05, 0x08, 0x14, 0x04, 0x0E, 0x04, 0x04, 0x04, 0x00 }; 00177 const char Letter_g[] = { 0x67, 0x05, 0x00, 0x00, 0x1E, 0x11, 0x1E, 0x10, 0x0F, 0x00 }; 00178 const char Letter_h[] = { 0x68, 0x05, 0x01, 0x01, 0x01, 0x0F, 0x11, 0x11, 0x11, 0x00 }; 00179 const char Letter_i[] = { 0x69, 0x05, 0x00, 0x04, 0x00, 0x06, 0x04, 0x04, 0x0E, 0x00 }; 00180 const char Letter_j[] = { 0x6A, 0x05, 0x08, 0x00, 0x08, 0x08, 0x08, 0x09, 0x06, 0x00 }; 00181 const char Letter_k[] = { 0x6B, 0x05, 0x02, 0x02, 0x12, 0x0A, 0x06, 0x0A, 0x12, 0x00 }; 00182 const char Letter_l[] = { 0x6C, 0x05, 0x06, 0x04, 0x04, 0x04, 0x04, 0x04, 0x0E, 0x00 }; 00183 const char Letter_m[] = { 0x6D, 0x05, 0x00, 0x00, 0x1B, 0x15, 0x15, 0x11, 0x11, 0x00 }; 00184 const char Letter_n[] = { 0x6E, 0x05, 0x00, 0x00, 0x0D, 0x13, 0x11, 0x11, 0x11, 0x00 }; 00185 const char Letter_o[] = { 0x6F, 0x05, 0x00, 0x00, 0x0E, 0x11, 0x11, 0x11, 0x0E, 0x00 }; 00186 const char Letter_p[] = { 0x70, 0x05, 0x00, 0x00, 0x0F, 0x11, 0x0F, 0x01, 0x01, 0x00 }; 00187 const char Letter_q[] = { 0x71, 0x05, 0x00, 0x00, 0x1E, 0x11, 0x1E, 0x10, 0x10, 0x00 }; 00188 const char Letter_r[] = { 0x72, 0x05, 0x00, 0x00, 0x0D, 0x13, 0x01, 0x01, 0x01, 0x00 }; 00189 const char Letter_s[] = { 0x73, 0x05, 0x00, 0x00, 0x1E, 0x01, 0x0E, 0x10, 0x0F, 0x00 }; 00190 const char Letter_t[] = { 0x74, 0x05, 0x04, 0x04, 0x1F, 0x04, 0x04, 0x14, 0x08, 0x00 }; 00191 const char Letter_u[] = { 0x75, 0x05, 0x00, 0x00, 0x11, 0x11, 0x11, 0x11, 0x0E, 0x00 }; 00192 const char Letter_v[] = { 0x76, 0x05, 0x00, 0x00, 0x11, 0x11, 0x11, 0x0A, 0x04, 0x00 }; 00193 const char Letter_w[] = { 0x77, 0x05, 0x00, 0x00, 0x11, 0x11, 0x15, 0x15, 0x0A, 0x00 }; 00194 const char Letter_x[] = { 0x78, 0x05, 0x00, 0x00, 0x11, 0x0A, 0x04, 0x0A, 0x11, 0x00 }; 00195 const char Letter_y[] = { 0x79, 0x05, 0x00, 0x00, 0x11, 0x0A, 0x04, 0x04, 0x02, 0x00 }; 00196 const char Letter_z[] = { 0x7A, 0x05, 0x00, 0x00, 0x1F, 0x08, 0x04, 0x02, 0x1F, 0x00 }; 00197 00198 const char Letter_0[] = { 0x30, 0x05, 0x0E, 0x11, 0x19, 0x15, 0x13, 0x11, 0x0E, 0x00 }; 00199 const char Letter_1[] = { 0x31, 0x05, 0x04, 0x06, 0x04, 0x04, 0x04, 0x04, 0x0E, 0x00 }; 00200 const char Letter_2[] = { 0x32, 0x05, 0x0E, 0x11, 0x10, 0x08, 0x04, 0x02, 0x1F, 0x00 }; 00201 const char Letter_3[] = { 0x33, 0x05, 0x1F, 0x08, 0x04, 0x08, 0x10, 0x11, 0x0E, 0x00 }; 00202 const char Letter_4[] = { 0x34, 0x05, 0x08, 0x0C, 0x0A, 0x09, 0x1F, 0x08, 0x08, 0x00 }; 00203 const char Letter_5[] = { 0x35, 0x05, 0x1F, 0x01, 0x0F, 0x10, 0x10, 0x11, 0x0E, 0x00 }; 00204 const char Letter_6[] = { 0x36, 0x05, 0x0C, 0x02, 0x01, 0x0F, 0x11, 0x11, 0x0E, 0x00 }; 00205 const char Letter_7[] = { 0x37, 0x05, 0x1F, 0x10, 0x08, 0x04, 0x02, 0x02, 0x02, 0x00 }; 00206 const char Letter_8[] = { 0x38, 0x05, 0x0E, 0x11, 0x11, 0x0E, 0x11, 0x11, 0x0E, 0x00 }; 00207 const char Letter_9[] = { 0x39, 0x05, 0x0E, 0x11, 0x11, 0x1E, 0x10, 0x08, 0x06, 0x00 }; 00208 00209 const char Letter_CH21[] = { 0x21, 0x05, 0x04, 0x04, 0x04, 0x04, 0x00, 0x00, 0x04, 0x00 }; 00210 const char Letter_CH22[] = { 0x22, 0x05, 0x0A, 0x0A, 0x0A, 0x00, 0x00, 0x00, 0x00, 0x00 }; 00211 const char Letter_CH23[] = { 0x23, 0x05, 0x0A, 0x0A, 0x1F, 0x0A, 0x1F, 0x0A, 0x0A, 0x00 }; 00212 const char Letter_CH24[] = { 0x24, 0x05, 0x04, 0x1E, 0x05, 0x0E, 0x14, 0x0F, 0x04, 0x00 }; 00213 const char Letter_CH25[] = { 0x25, 0x05, 0x03, 0x13, 0x08, 0x04, 0x02, 0x19, 0x18, 0x00 }; 00214 const char Letter_CH26[] = { 0x26, 0x05, 0x06, 0x09, 0x05, 0x02, 0x15, 0x09, 0x16, 0x00 }; 00215 const char Letter_CH27[] = { 0x27, 0x05, 0x06, 0x04, 0x02, 0x00, 0x00, 0x00, 0x00, 0x00 }; 00216 const char Letter_CH28[] = { 0x28, 0x05, 0x08, 0x04, 0x02, 0x02, 0x02, 0x04, 0x08, 0x00 }; 00217 const char Letter_CH29[] = { 0x29, 0x05, 0x02, 0x04, 0x08, 0x08, 0x08, 0x04, 0x02, 0x00 }; 00218 const char Letter_CH2A[] = { 0x2A, 0x05, 0x00, 0x04, 0x15, 0x0E, 0x15, 0x04, 0x00, 0x00 }; 00219 const char Letter_CH2B[] = { 0x2B, 0x05, 0x00, 0x04, 0x04, 0x1F, 0x04, 0x04, 0x00, 0x00 }; 00220 const char Letter_CH2C[] = { 0x2C, 0x05, 0x00, 0x00, 0x00, 0x00, 0x06, 0x04, 0x02, 0x00 }; 00221 const char Letter_CH2D[] = { 0x2D, 0x05, 0x00, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x00, 0x00 }; 00222 const char Letter_CH2E[] = { 0x2E, 0x05, 0x00, 0x00, 0x00, 0x00, 0x00, 0x06, 0x06, 0x00 }; 00223 const char Letter_CH2F[] = { 0x2F, 0x05, 0x00, 0x10, 0x08, 0x04, 0x02, 0x01, 0x00, 0x00 }; 00224 00225 const char Letter_CH3A[] = { 0x3A, 0x05, 0x00, 0x06, 0x06, 0x00, 0x06, 0x06, 0x00, 0x00 }; 00226 const char Letter_CH3B[] = { 0x3B, 0x05, 0x00, 0x06, 0x06, 0x00, 0x06, 0x04, 0x02, 0x00 }; 00227 const char Letter_CH3C[] = { 0x3C, 0x05, 0x08, 0x04, 0x02, 0x01, 0x02, 0x04, 0x08, 0x00 }; 00228 const char Letter_CH3D[] = { 0x3D, 0x05, 0x00, 0x00, 0x1F, 0x00, 0x1F, 0x00, 0x00, 0x00 }; 00229 const char Letter_CH3E[] = { 0x3E, 0x05, 0x02, 0x04, 0x08, 0x10, 0x08, 0x04, 0x02, 0x00 }; 00230 const char Letter_CH3F[] = { 0x3F, 0x05, 0x0E, 0x11, 0x10, 0x08, 0x04, 0x00, 0x04, 0x00 }; 00231 const char Letter_CH40[] = { 0x40, 0x05, 0x0E, 0x11, 0x10, 0x16, 0x15, 0x15, 0x0E, 0x00 }; 00232 00233 const char unArray[] = { 0x00, 0x05, 0x00, 0x00, 0x0A, 0x04, 0x0A, 0x00, 0x00, 0x00 }; 00234 00235 const char Letter_Space[] = { 0x20, 0x05, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 }; 00236 00237 00238 //char CharArray[text.size()]; 00239 //strncpy(CharArray, text.c_str(), sizeof(CharArray)); 00240 //CharArray[sizeof(CharArray) - 1] = 0; 00241 00242 int xOffset = 0; 00243 00244 for (int i = 0; i < 255; i++) { 00245 char ArrayToUse[10] = { 0 }; 00246 char cha = CharArray[i]; 00247 int LetterHeigth = 8; 00248 int LetterWidth; 00249 00250 switch (cha) { //Select char-pattern 00251 case 'A': 00252 for(int i = 0; i < 10; i++) { 00253 ArrayToUse[i] = Letter_A[i]; 00254 } 00255 break; 00256 case 'B': 00257 for(int i = 0; i < 10; i++) { 00258 ArrayToUse[i] = Letter_B[i]; 00259 } 00260 break; 00261 case 'C': 00262 for(int i = 0; i < 10; i++) { 00263 ArrayToUse[i] = Letter_C[i]; 00264 } 00265 break; 00266 case 'D': 00267 for(int i = 0; i < 10; i++) { 00268 ArrayToUse[i] = Letter_D[i]; 00269 } 00270 break; 00271 case 'E': 00272 for(int i = 0; i < 10; i++) { 00273 ArrayToUse[i] = Letter_E[i]; 00274 } 00275 break; 00276 case 'F': 00277 for(int i = 0; i < 10; i++) { 00278 ArrayToUse[i] = Letter_F[i]; 00279 } 00280 break; 00281 case 'G': 00282 for(int i = 0; i < 10; i++) { 00283 ArrayToUse[i] = Letter_G[i]; 00284 } 00285 break; 00286 case 'H': 00287 for(int i = 0; i < 10; i++) { 00288 ArrayToUse[i] = Letter_H[i]; 00289 } 00290 break; 00291 case 'I': 00292 for(int i = 0; i < 10; i++) { 00293 ArrayToUse[i] = Letter_I[i]; 00294 } 00295 break; 00296 case 'J': 00297 for(int i = 0; i < 10; i++) { 00298 ArrayToUse[i] = Letter_J[i]; 00299 } 00300 break; 00301 case 'K': 00302 for(int i = 0; i < 10; i++) { 00303 ArrayToUse[i] = Letter_K[i]; 00304 } 00305 break; 00306 case 'L': 00307 for(int i = 0; i < 10; i++) { 00308 ArrayToUse[i] = Letter_L[i]; 00309 } 00310 break; 00311 case 'M': 00312 for(int i = 0; i < 10; i++) { 00313 ArrayToUse[i] = Letter_M[i]; 00314 } 00315 break; 00316 case 'N': 00317 for(int i = 0; i < 10; i++) { 00318 ArrayToUse[i] = Letter_N[i]; 00319 } 00320 break; 00321 case 'O': 00322 for(int i = 0; i < 10; i++) { 00323 ArrayToUse[i] = Letter_O[i]; 00324 } 00325 break; 00326 case 'P': 00327 for(int i = 0; i < 10; i++) { 00328 ArrayToUse[i] = Letter_P[i]; 00329 } 00330 break; 00331 case 'Q': 00332 for(int i = 0; i < 10; i++) { 00333 ArrayToUse[i] = Letter_Q[i]; 00334 } 00335 break; 00336 case 'R': 00337 for(int i = 0; i < 10; i++) { 00338 ArrayToUse[i] = Letter_R[i]; 00339 } 00340 break; 00341 case 'S': 00342 for(int i = 0; i < 10; i++) { 00343 ArrayToUse[i] = Letter_S[i]; 00344 } 00345 break; 00346 case 'T': 00347 for(int i = 0; i < 10; i++) { 00348 ArrayToUse[i] = Letter_T[i]; 00349 } 00350 break; 00351 case 'U': 00352 for(int i = 0; i < 10; i++) { 00353 ArrayToUse[i] = Letter_U[i]; 00354 } 00355 break; 00356 case 'V': 00357 for(int i = 0; i < 10; i++) { 00358 ArrayToUse[i] = Letter_V[i]; 00359 } 00360 break; 00361 case 'W': 00362 for(int i = 0; i < 10; i++) { 00363 ArrayToUse[i] = Letter_W[i]; 00364 } 00365 break; 00366 case 'X': 00367 for(int i = 0; i < 10; i++) { 00368 ArrayToUse[i] = Letter_X[i]; 00369 } 00370 break; 00371 case 'Y': 00372 for(int i = 0; i < 10; i++) { 00373 ArrayToUse[i] = Letter_Y[i]; 00374 } 00375 break; 00376 case 'Z': 00377 for(int i = 0; i < 10; i++) { 00378 ArrayToUse[i] = Letter_Z[i]; 00379 } 00380 break; 00381 00382 case 'a': 00383 for(int i = 0; i < 10; i++) { 00384 ArrayToUse[i] = Letter_a[i]; 00385 } 00386 break; 00387 case 'b': 00388 for(int i = 0; i < 10; i++) { 00389 ArrayToUse[i] = Letter_b[i]; 00390 } 00391 break; 00392 case 'c': 00393 for(int i = 0; i < 10; i++) { 00394 ArrayToUse[i] = Letter_c[i]; 00395 } 00396 break; 00397 case 'd': 00398 for(int i = 0; i < 10; i++) { 00399 ArrayToUse[i] = Letter_d[i]; 00400 } 00401 break; 00402 case 'e': 00403 for(int i = 0; i < 10; i++) { 00404 ArrayToUse[i] = Letter_e[i]; 00405 } 00406 break; 00407 case 'f': 00408 for(int i = 0; i < 10; i++) { 00409 ArrayToUse[i] = Letter_f[i]; 00410 } 00411 break; 00412 case 'g': 00413 for(int i = 0; i < 10; i++) { 00414 ArrayToUse[i] = Letter_g[i]; 00415 } 00416 break; 00417 case 'h': 00418 for(int i = 0; i < 10; i++) { 00419 ArrayToUse[i] = Letter_h[i]; 00420 } 00421 break; 00422 case 'i': 00423 for(int i = 0; i < 10; i++) { 00424 ArrayToUse[i] = Letter_i[i]; 00425 } 00426 break; 00427 case 'j': 00428 for(int i = 0; i < 10; i++) { 00429 ArrayToUse[i] = Letter_j[i]; 00430 } 00431 break; 00432 case 'k': 00433 for(int i = 0; i < 10; i++) { 00434 ArrayToUse[i] = Letter_k[i]; 00435 } 00436 break; 00437 case 'l': 00438 for(int i = 0; i < 10; i++) { 00439 ArrayToUse[i] = Letter_l[i]; 00440 } 00441 break; 00442 case 'm': 00443 for(int i = 0; i < 10; i++) { 00444 ArrayToUse[i] = Letter_m[i]; 00445 } 00446 break; 00447 case 'n': 00448 for(int i = 0; i < 10; i++) { 00449 ArrayToUse[i] = Letter_n[i]; 00450 } 00451 break; 00452 case 'o': 00453 for(int i = 0; i < 10; i++) { 00454 ArrayToUse[i] = Letter_o[i]; 00455 } 00456 break; 00457 case 'p': 00458 for(int i = 0; i < 10; i++) { 00459 ArrayToUse[i] = Letter_p[i]; 00460 } 00461 break; 00462 case 'q': 00463 for(int i = 0; i < 10; i++) { 00464 ArrayToUse[i] = Letter_q[i]; 00465 } 00466 break; 00467 case 'r': 00468 for(int i = 0; i < 10; i++) { 00469 ArrayToUse[i] = Letter_r[i]; 00470 } 00471 break; 00472 case 's': 00473 for(int i = 0; i < 10; i++) { 00474 ArrayToUse[i] = Letter_s[i]; 00475 } 00476 break; 00477 case 't': 00478 for(int i = 0; i < 10; i++) { 00479 ArrayToUse[i] = Letter_t[i]; 00480 } 00481 break; 00482 case 'u': 00483 for(int i = 0; i < 10; i++) { 00484 ArrayToUse[i] = Letter_u[i]; 00485 } 00486 break; 00487 case 'v': 00488 for(int i = 0; i < 10; i++) { 00489 ArrayToUse[i] = Letter_v[i]; 00490 } 00491 break; 00492 case 'w': 00493 for(int i = 0; i < 10; i++) { 00494 ArrayToUse[i] = Letter_w[i]; 00495 } 00496 break; 00497 case 'x': 00498 for(int i = 0; i < 10; i++) { 00499 ArrayToUse[i] = Letter_x[i]; 00500 } 00501 break; 00502 case 'y': 00503 for(int i = 0; i < 10; i++) { 00504 ArrayToUse[i] = Letter_y[i]; 00505 } 00506 break; 00507 case 'z': 00508 for(int i = 0; i < 10; i++) { 00509 ArrayToUse[i] = Letter_z[i]; 00510 } 00511 break; 00512 00513 case '0': 00514 for(int i = 0; i < 10; i++) { 00515 ArrayToUse[i] = Letter_0[i]; 00516 } 00517 break; 00518 case '1': 00519 for(int i = 0; i < 10; i++) { 00520 ArrayToUse[i] = Letter_1[i]; 00521 } 00522 break; 00523 case '2': 00524 for(int i = 0; i < 10; i++) { 00525 ArrayToUse[i] = Letter_2[i]; 00526 } 00527 break; 00528 case '3': 00529 for(int i = 0; i < 10; i++) { 00530 ArrayToUse[i] = Letter_3[i]; 00531 } 00532 break; 00533 case '4': 00534 for(int i = 0; i < 10; i++) { 00535 ArrayToUse[i] = Letter_4[i]; 00536 } 00537 break; 00538 case '5': 00539 for(int i = 0; i < 10; i++) { 00540 ArrayToUse[i] = Letter_5[i]; 00541 } 00542 break; 00543 case '6': 00544 for(int i = 0; i < 10; i++) { 00545 ArrayToUse[i] = Letter_6[i]; 00546 } 00547 break; 00548 case '7': 00549 for(int i = 0; i < 10; i++) { 00550 ArrayToUse[i] = Letter_7[i]; 00551 } 00552 break; 00553 case '8': 00554 for(int i = 0; i < 10; i++) { 00555 ArrayToUse[i] = Letter_8[i]; 00556 } 00557 break; 00558 case '9': 00559 for(int i = 0; i < 10; i++) { 00560 ArrayToUse[i] = Letter_9[i]; 00561 } 00562 break; 00563 00564 case '!': 00565 for(int i = 0; i < 10; i++) { 00566 ArrayToUse[i] = Letter_CH21[i]; 00567 } 00568 break; 00569 case '"': 00570 for(int i = 0; i < 10; i++) { 00571 ArrayToUse[i] = Letter_CH22[i]; 00572 } 00573 break; 00574 case '#': 00575 for(int i = 0; i < 10; i++) { 00576 ArrayToUse[i] = Letter_CH23[i]; 00577 } 00578 break; 00579 case '$': 00580 for(int i = 0; i < 10; i++) { 00581 ArrayToUse[i] = Letter_CH24[i]; 00582 } 00583 break; 00584 case '%': 00585 for(int i = 0; i < 10; i++) { 00586 ArrayToUse[i] = Letter_CH25[i]; 00587 } 00588 break; 00589 case '&': 00590 for(int i = 0; i < 10; i++) { 00591 ArrayToUse[i] = Letter_CH26[i]; 00592 } 00593 break; 00594 case '\'': 00595 for(int i = 0; i < 10; i++) { 00596 ArrayToUse[i] = Letter_CH27[i]; 00597 } 00598 break; 00599 case '(': 00600 for(int i = 0; i < 10; i++) { 00601 ArrayToUse[i] = Letter_CH28[i]; 00602 } 00603 break; 00604 case ')': 00605 for(int i = 0; i < 10; i++) { 00606 ArrayToUse[i] = Letter_CH29[i]; 00607 } 00608 break; 00609 case '*': 00610 for(int i = 0; i < 10; i++) { 00611 ArrayToUse[i] = Letter_CH2A[i]; 00612 } 00613 break; 00614 case '+': 00615 for(int i = 0; i < 10; i++) { 00616 ArrayToUse[i] = Letter_CH2B[i]; 00617 } 00618 break; 00619 case ',': 00620 for(int i = 0; i < 10; i++) { 00621 ArrayToUse[i] = Letter_CH2C[i]; 00622 } 00623 break; 00624 case '-': 00625 for(int i = 0; i < 10; i++) { 00626 ArrayToUse[i] = Letter_CH2D[i]; 00627 } 00628 break; 00629 case '.': 00630 for(int i = 0; i < 10; i++) { 00631 ArrayToUse[i] = Letter_CH2E[i]; 00632 } 00633 break; 00634 case '/': 00635 for(int i = 0; i < 10; i++) { 00636 ArrayToUse[i] = Letter_CH2F[i]; 00637 } 00638 break; 00639 00640 case ':': 00641 for(int i = 0; i < 10; i++) { 00642 ArrayToUse[i] = Letter_CH3A[i]; 00643 } 00644 break; 00645 case ';': 00646 for(int i = 0; i < 10; i++) { 00647 ArrayToUse[i] = Letter_CH3B[i]; 00648 } 00649 break; 00650 case '<': 00651 for(int i = 0; i < 10; i++) { 00652 ArrayToUse[i] = Letter_CH3C[i]; 00653 } 00654 break; 00655 case '=': 00656 for(int i = 0; i < 10; i++) { 00657 ArrayToUse[i] = Letter_CH3D[i]; 00658 } 00659 break; 00660 case '>': 00661 for(int i = 0; i < 10; i++) { 00662 ArrayToUse[i] = Letter_CH3E[i]; 00663 } 00664 break; 00665 case '?': 00666 for(int i = 0; i < 10; i++) { 00667 ArrayToUse[i] = Letter_CH3F[i]; 00668 } 00669 break; 00670 case '@': 00671 for(int i = 0; i < 10; i++) { 00672 ArrayToUse[i] = Letter_CH40[i]; 00673 } 00674 break; 00675 00676 case ' ': 00677 for(int i = 0; i < 10; i++) { 00678 ArrayToUse[i] = Letter_Space[i]; 00679 } 00680 break; 00681 00682 case '\0': //if the end of string char is found, end function 00683 return; 00684 00685 00686 default: 00687 for(int i = 0; i < 10; i++) { 00688 ArrayToUse[i] = unArray[i]; 00689 } 00690 break; 00691 } 00692 // ASCII Width 1stR 2ndR 3rdR 4thR 5thR 6thR 7thR 8thR 00693 // Letter_A = { 0x41, 0x05, 0x0E, 0x11, 0x11, 0x11, 0x1F, 0x11, 0x11, 0x00 } 00694 LetterWidth = ArrayToUse[1]; 00695 00696 if (UseLargeFont) { 00697 for (int LetterRow = 0; LetterRow < sizeof ArrayToUse - 2; LetterRow++) { 00698 if (((ArrayToUse[LetterRow + 2] & Bit0) == Bit0) && LetterWidth > 0) { 00699 DrawPixel(x + xOffset + 0, y + (LetterRow * 2) + 1); 00700 DrawPixel(x + xOffset + 1, y + (LetterRow * 2) + 1); 00701 DrawPixel(x + xOffset + 0, y + (LetterRow * 2) + 0); 00702 DrawPixel(x + xOffset + 1, y + (LetterRow * 2) + 0); 00703 } 00704 if (((ArrayToUse[LetterRow + 2] & Bit1) == Bit1) && LetterWidth > 1) { 00705 DrawPixel(x + xOffset + 2, y + (LetterRow * 2) + 1); 00706 DrawPixel(x + xOffset + 3, y + (LetterRow * 2) + 1); 00707 DrawPixel(x + xOffset + 2, y + (LetterRow * 2) + 0); 00708 DrawPixel(x + xOffset + 3, y + (LetterRow * 2) + 0); 00709 } 00710 if (((ArrayToUse[LetterRow + 2] & Bit2) == Bit2) && LetterWidth > 2) { 00711 DrawPixel(x + xOffset + 4, y + (LetterRow * 2) + 1); 00712 DrawPixel(x + xOffset + 5, y + (LetterRow * 2) + 1); 00713 DrawPixel(x + xOffset + 4, y + (LetterRow * 2) + 0); 00714 DrawPixel(x + xOffset + 5, y + (LetterRow * 2) + 0); 00715 } 00716 if (((ArrayToUse[LetterRow + 2] & Bit3) == Bit3) && LetterWidth > 3) { 00717 DrawPixel(x + xOffset + 6, y + (LetterRow * 2) + 1); 00718 DrawPixel(x + xOffset + 7, y + (LetterRow * 2) + 1); 00719 DrawPixel(x + xOffset + 6, y + (LetterRow * 2) + 0); 00720 DrawPixel(x + xOffset + 7, y + (LetterRow * 2) + 0); 00721 } 00722 if (((ArrayToUse[LetterRow + 2] & Bit4) == Bit4) && LetterWidth > 4) { 00723 DrawPixel(x + xOffset + 8, y + (LetterRow * 2) + 1); 00724 DrawPixel(x + xOffset + 9, y + (LetterRow * 2) + 1); 00725 DrawPixel(x + xOffset + 8, y + (LetterRow * 2) + 0); 00726 DrawPixel(x + xOffset + 9, y + (LetterRow * 2) + 0); 00727 } 00728 if (((ArrayToUse[LetterRow + 2] & Bit5) == Bit5) && LetterWidth > 5) { 00729 DrawPixel(x + xOffset + 10, y + (LetterRow * 2) + 1); 00730 DrawPixel(x + xOffset + 11, y + (LetterRow * 2) + 1); 00731 DrawPixel(x + xOffset + 10, y + (LetterRow * 2) + 0); 00732 DrawPixel(x + xOffset + 11, y + (LetterRow * 2) + 0); 00733 } 00734 if (((ArrayToUse[LetterRow + 2] & Bit6) == Bit6) && LetterWidth > 6) { 00735 DrawPixel(x + xOffset + 12, y + (LetterRow * 2) + 1); 00736 DrawPixel(x + xOffset + 13, y + (LetterRow * 2) + 1); 00737 DrawPixel(x + xOffset + 12, y + (LetterRow * 2) + 0); 00738 DrawPixel(x + xOffset + 13, y + (LetterRow * 2) + 0); 00739 } 00740 if (((ArrayToUse[LetterRow + 2] & Bit7) == Bit7) && LetterWidth > 7) { 00741 DrawPixel(x + xOffset + 14, y + (LetterRow * 2) + 1); 00742 DrawPixel(x + xOffset + 15, y + (LetterRow * 2) + 1); 00743 DrawPixel(x + xOffset + 14, y + (LetterRow * 2) + 0); 00744 DrawPixel(x + xOffset + 15, y + (LetterRow * 2) + 0); 00745 } 00746 } 00747 00748 xOffset += (LetterWidth * 2) + 1; 00749 } 00750 00751 else { 00752 for (int LetterRow = 2; LetterRow < sizeof ArrayToUse; LetterRow++) { 00753 if (((ArrayToUse[LetterRow] & Bit0) == Bit0) && LetterWidth > 0) 00754 DrawPixel(x + xOffset + 0, y + LetterRow - 2); 00755 if (((ArrayToUse[LetterRow] & Bit1) == Bit1) && LetterWidth > 1) 00756 DrawPixel(x + xOffset + 1, y + LetterRow - 2); 00757 if (((ArrayToUse[LetterRow] & Bit2) == Bit2) && LetterWidth > 2) 00758 DrawPixel(x + xOffset + 2, y + LetterRow - 2); 00759 if (((ArrayToUse[LetterRow] & Bit3) == Bit3) && LetterWidth > 3) 00760 DrawPixel(x + xOffset + 3, y + LetterRow - 2); 00761 if (((ArrayToUse[LetterRow] & Bit4) == Bit4) && LetterWidth > 4) 00762 DrawPixel(x + xOffset + 4, y + LetterRow - 2); 00763 if (((ArrayToUse[LetterRow] & Bit5) == Bit5) && LetterWidth > 5) 00764 DrawPixel(x + xOffset + 5, y + LetterRow - 2); 00765 if (((ArrayToUse[LetterRow] & Bit6) == Bit6) && LetterWidth > 6) 00766 DrawPixel(x + xOffset + 6, y + LetterRow - 2); 00767 if (((ArrayToUse[LetterRow] & Bit7) == Bit7) && LetterWidth > 7) 00768 DrawPixel(x + xOffset + 7, y + LetterRow - 2); 00769 } 00770 00771 xOffset += LetterWidth + 1; 00772 } 00773 } 00774 00775 } 00776 00777 void TinyBitmap::ClearScreen() 00778 { 00779 int size = (_IMAGEWIDTH * _IMAGEHEIGHT / 8) + _COMMANDBYTES; 00780 00781 for (int i = _COMMANDBYTES; i < size; i++) { 00782 _FRAMEBUFFER[i] = 0x00; 00783 } 00784 } 00785
Generated on Fri Jul 15 2022 16:38:52 by
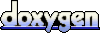