
Código para programar un PID por medio de un teclado matricial y un LCD.
Dependencies: FPointer TextLCD keypad mbed
main.cpp
00001 #include "mbed.h" 00002 #include "keypad.h" 00003 #include "TextLCD.h" 00004 #include "stdlib.h" 00005 00006 TextLCD lcd(PTB10, PTB11, PTE2, PTE3, PTE4, PTE5); // rs, e, d4-d7 00007 int C1=0x0F; // Cursor 00008 int C4=0x0C; // quito cursor bajo 00009 int kpnum=0,kinum=0,spnum=0,kdnum=0,pos=1,tecla,flag1=1,num=0; 00010 int flagt=0; 00011 Timer t; 00012 char cadena[3]={' ',' ',' '}; 00013 int llena=0; //verificar que la entrada es de 3 numeros 00014 // Define your own keypad values 00015 char Keytable[] = { '1', '2', '3', 'A', 00016 '4', '5', '6', 'B', 00017 '7', '8', '9', 'C', 00018 '*', '0', '#', 'D' 00019 }; 00020 00021 uint32_t cbAfterInput(uint32_t index) { 00022 tecla=index; 00023 flag1=0; 00024 return 0; 00025 } 00026 00027 int main() { 00028 // f0 f1 f2 f3 c0 c1 c2 c3 00029 Keypad keypad(PTA1, PTA2, PTD4, PTA12,PTC7, PTC0, PTC3, PTC4); 00030 ini1: 00031 lcd.cls(); // Borrar Pantalla 00032 lcd.locate(0,0); 00033 lcd.printf("**PID-TECLADO**"); 00034 wait(1); 00035 lcd.cls(); 00036 00037 lcd.locate(0,0); 00038 lcd.printf("Kp=%d",kpnum); 00039 lcd.locate(8,0); 00040 lcd.printf("Ki=%d",kinum); 00041 lcd.locate(0,1); 00042 lcd.printf("Kd=%d",kdnum); 00043 lcd.locate(8,1); 00044 lcd.printf("Sp=%d",spnum); 00045 00046 //lcd.locate(3,0); 00047 //lcd.writeCommand(C1);//escribimos un comando segun el manual del modulo LCD 00048 00049 ini2: 00050 keypad.CallAfterInput(&cbAfterInput); 00051 keypad.Start(); 00052 if(flag1==0){ 00053 if(Keytable[tecla]=='B'){ 00054 cadena[0]=' ';cadena[1]=' ';cadena[2]=' '; 00055 llena=0; 00056 num=0; 00057 if(pos==4){ 00058 pos=1;} 00059 else { 00060 pos++;} 00061 } 00062 else if(Keytable[tecla]=='A'){ 00063 llena--; 00064 cadena[llena]=' '; 00065 num=strtod(cadena,NULL); 00066 } 00067 else if((tecla==0)||(tecla==1)||(tecla==2)||(tecla==4)||(tecla==5)||(tecla==6)||(tecla==8)||(tecla==9)||(tecla==10)||(tecla==13)){ 00068 if(llena<3){ 00069 cadena[llena]=Keytable[tecla]; 00070 num=strtod(cadena,NULL); 00071 llena++;} 00072 } 00073 else if(Keytable[tecla]=='D'){ 00074 lcd.writeCommand(C4);//escribimos un comando segun el manual del modulo LCD para quitar cursor bajo 00075 lcd.cls(); //borra la pantalla 00076 lcd.printf(" GUARDADOS!"); 00077 wait(1); 00078 lcd.cls(); 00079 lcd.locate(0,0); 00080 lcd.printf("Kp=%d",kpnum); 00081 lcd.locate(8,0); 00082 lcd.printf("Ki=%d",kinum); 00083 lcd.locate(0,1); 00084 lcd.printf("Kd=%d",kdnum); 00085 lcd.locate(8,1); 00086 lcd.printf("Sp=%d",spnum); 00087 wait(2); 00088 cicloPID: 00089 if(Keytable[tecla]=='C'){ 00090 kpnum=0;kinum=0;kdnum=0;spnum=0;llena=0;pos=1;flag1=1; 00091 cadena[0]=' ';cadena[1]=' ';cadena[2]=' '; 00092 goto ini1;} 00093 00094 if(flagt==0){//se muestran las variables 00095 t.start(); 00096 flagt=1;} 00097 if(t>=0.3){ 00098 lcd.locate(3,0);lcd.printf(" "); 00099 lcd.locate(3,0);lcd.printf("%d",kpnum); 00100 lcd.locate(11,0);lcd.printf(" "); 00101 lcd.locate(11,0);lcd.printf("%d",kinum); 00102 lcd.locate(3,1);lcd.printf(" "); 00103 lcd.locate(3,1);lcd.printf("%d",kdnum); 00104 lcd.locate(11,1);lcd.printf(" "); 00105 lcd.locate(11,1);lcd.printf("%d",spnum); 00106 flagt=0; 00107 t.reset(); 00108 } 00109 goto cicloPID; 00110 } 00111 if(pos==1){ 00112 kpnum=num; 00113 lcd.locate(3,0);lcd.writeCommand(C1); 00114 lcd.locate(3,0);lcd.printf(" "); 00115 lcd.locate(3,0);lcd.printf("%d",kpnum); 00116 } 00117 else if(pos==2){ 00118 kinum=num; 00119 lcd.locate(11,0);lcd.printf(" "); 00120 lcd.locate(11,0);lcd.printf("%d",kinum); 00121 } 00122 else if(pos==3){ 00123 kdnum=num; 00124 lcd.locate(3,1);lcd.printf(" "); 00125 lcd.locate(3,1);lcd.printf("%d",kdnum); 00126 } 00127 else if(pos==4){ 00128 spnum=num; 00129 lcd.locate(11,1);lcd.printf(" "); 00130 lcd.locate(11,1);lcd.printf("%d",spnum); 00131 } 00132 } 00133 flag1=1; 00134 goto ini2; 00135 }
Generated on Mon Jul 18 2022 01:31:32 by
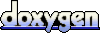