
Trying to make Modbus code into a lib
Modbus
Enumerations | |
enum | eMBMode { MB_RTU, MB_ASCII, MB_TCP } |
Modbus serial transmission modes (RTU/ASCII). More... | |
enum | eMBRegisterMode { MB_REG_READ, MB_REG_WRITE } |
If register should be written or read. More... | |
enum | eMBErrorCode { MB_ENOERR, MB_ENOREG, MB_EINVAL, MB_EPORTERR, MB_ENORES, MB_EIO, MB_EILLSTATE, MB_ETIMEDOUT } |
Errorcodes used by all function in the protocol stack. More... | |
enum | eMBParity { MB_PAR_NONE, MB_PAR_ODD, MB_PAR_EVEN } |
Parity used for characters in serial mode. More... | |
Functions | |
eMBErrorCode | eMBInit (eMBMode eMode, UCHAR ucSlaveAddress, UCHAR ucPort, ULONG ulBaudRate, eMBParity eParity) |
Initialize the Modbus protocol stack. | |
eMBErrorCode | eMBTCPInit (USHORT usTCPPort) |
Initialize the Modbus protocol stack for Modbus TCP. | |
eMBErrorCode | eMBClose (void) |
Release resources used by the protocol stack. | |
eMBErrorCode | eMBEnable (void) |
Enable the Modbus protocol stack. | |
eMBErrorCode | eMBDisable (void) |
Disable the Modbus protocol stack. | |
eMBErrorCode | eMBPoll (void) |
The main pooling loop of the Modbus protocol stack. | |
eMBErrorCode | eMBSetSlaveID (UCHAR ucSlaveID, BOOL xIsRunning, UCHAR const *pucAdditional, USHORT usAdditionalLen) |
Configure the slave id of the device. | |
eMBErrorCode | eMBRegisterCB (UCHAR ucFunctionCode, pxMBFunctionHandler pxHandler) |
Registers a callback handler for a given function code. |
Detailed Description
#include "mb.h"
This module defines the interface for the application. It contains the basic functions and types required to use the Modbus protocol stack. A typical application will want to call eMBInit() first. If the device is ready to answer network requests it must then call eMBEnable() to activate the protocol stack. In the main loop the function eMBPoll() must be called periodically. The time interval between pooling depends on the configured Modbus timeout. If an RTOS is available a separate task should be created and the task should always call the function eMBPoll().
// Initialize protocol stack in RTU mode for a slave with address 10 = 0x0A eMBInit( MB_RTU , 0x0A, 38400, MB_PAR_EVEN ); // Enable the Modbus Protocol Stack. eMBEnable( ); for( ;; ) { // Call the main polling loop of the Modbus protocol stack. eMBPoll( ); ... }
Enumeration Type Documentation
enum eMBErrorCode |
Errorcodes used by all function in the protocol stack.
enum eMBMode |
Modbus serial transmission modes (RTU/ASCII).
Modbus serial supports two transmission modes. Either ASCII or RTU. RTU is faster but has more hardware requirements and requires a network with a low jitter. ASCII is slower and more reliable on slower links (E.g. modems)
enum eMBParity |
Parity used for characters in serial mode.
The parity which should be applied to the characters sent over the serial link. Please note that this values are actually passed to the porting layer and therefore not all parity modes might be available.
enum eMBRegisterMode |
If register should be written or read.
This value is passed to the callback functions which support either reading or writing register values. Writing means that the application registers should be updated and reading means that the modbus protocol stack needs to know the current register values.
- See also:
- eMBRegHoldingCB( ), eMBRegCoilsCB( ), eMBRegDiscreteCB( ) and eMBRegInputCB( ).
Function Documentation
eMBErrorCode eMBClose | ( | void | ) |
Release resources used by the protocol stack.
This function disables the Modbus protocol stack and release all hardware resources. It must only be called when the protocol stack is disabled.
- Note:
- Note all ports implement this function. A port which wants to get an callback must define the macro MB_PORT_HAS_CLOSE to 1.
- Returns:
- If the resources where released it return eMBErrorCode::MB_ENOERR. If the protocol stack is not in the disabled state it returns eMBErrorCode::MB_EILLSTATE.
eMBErrorCode eMBDisable | ( | void | ) |
eMBErrorCode eMBEnable | ( | void | ) |
Enable the Modbus protocol stack.
This function enables processing of Modbus frames. Enabling the protocol stack is only possible if it is in the disabled state.
- Returns:
- If the protocol stack is now in the state enabled it returns eMBErrorCode::MB_ENOERR. If it was not in the disabled state it return eMBErrorCode::MB_EILLSTATE.
eMBErrorCode eMBInit | ( | eMBMode | eMode, |
UCHAR | ucSlaveAddress, | ||
UCHAR | ucPort, | ||
ULONG | ulBaudRate, | ||
eMBParity | eParity | ||
) |
Initialize the Modbus protocol stack.
This functions initializes the ASCII or RTU module and calls the init functions of the porting layer to prepare the hardware. Please note that the receiver is still disabled and no Modbus frames are processed until eMBEnable( ) has been called.
- Parameters:
-
eMode If ASCII or RTU mode should be used. ucSlaveAddress The slave address. Only frames sent to this address or to the broadcast address are processed. ucPort The port to use. E.g. 1 for COM1 on windows. This value is platform dependent and some ports simply choose to ignore it. ulBaudRate The baudrate. E.g. 19200. Supported baudrates depend on the porting layer. eParity Parity used for serial transmission.
- Returns:
- If no error occurs the function returns eMBErrorCode::MB_ENOERR. The protocol is then in the disabled state and ready for activation by calling eMBEnable( ). Otherwise one of the following error codes is returned:
- eMBErrorCode::MB_EINVAL If the slave address was not valid. Valid slave addresses are in the range 1 - 247.
- eMBErrorCode::MB_EPORTERR IF the porting layer returned an error.
eMBErrorCode eMBPoll | ( | void | ) |
The main pooling loop of the Modbus protocol stack.
This function must be called periodically. The timer interval required is given by the application dependent Modbus slave timeout. Internally the function calls xMBPortEventGet() and waits for an event from the receiver or transmitter state machines.
- Returns:
- If the protocol stack is not in the enabled state the function returns eMBErrorCode::MB_EILLSTATE. Otherwise it returns eMBErrorCode::MB_ENOERR.
eMBErrorCode eMBRegisterCB | ( | UCHAR | ucFunctionCode, |
pxMBFunctionHandler | pxHandler | ||
) |
Registers a callback handler for a given function code.
This function registers a new callback handler for a given function code. The callback handler supplied is responsible for interpreting the Modbus PDU and the creation of an appropriate response. In case of an error it should return one of the possible Modbus exceptions which results in a Modbus exception frame sent by the protocol stack.
- Parameters:
-
ucFunctionCode The Modbus function code for which this handler should be registers. Valid function codes are in the range 1 to 127. pxHandler The function handler which should be called in case such a frame is received. If NULL
a previously registered function handler for this function code is removed.
- Returns:
- eMBErrorCode::MB_ENOERR if the handler has been installed. If no more resources are available it returns eMBErrorCode::MB_ENORES. In this case the values in mbconfig.h should be adjusted. If the argument was not valid it returns eMBErrorCode::MB_EINVAL.
eMBErrorCode eMBSetSlaveID | ( | UCHAR | ucSlaveID, |
BOOL | xIsRunning, | ||
UCHAR const * | pucAdditional, | ||
USHORT | usAdditionalLen | ||
) |
Configure the slave id of the device.
This function should be called when the Modbus function Report Slave ID is enabled ( By defining MB_FUNC_OTHER_REP_SLAVEID_ENABLED in mbconfig.h ).
- Parameters:
-
ucSlaveID Values is returned in the Slave ID byte of the Report Slave ID response. xIsRunning If TRUE the Run Indicator Status byte is set to 0xFF. otherwise the Run Indicator Status is 0x00. pucAdditional Values which should be returned in the Additional bytes of the Report Slave ID response. usAdditionalLen Length of the buffer pucAdditonal
.
- Returns:
- If the static buffer defined by MB_FUNC_OTHER_REP_SLAVEID_BUF in mbconfig.h is to small it returns eMBErrorCode::MB_ENORES. Otherwise it returns eMBErrorCode::MB_ENOERR.
Definition at line 53 of file mbfuncother.cpp.
eMBErrorCode eMBTCPInit | ( | USHORT | usTCPPort ) |
Initialize the Modbus protocol stack for Modbus TCP.
This function initializes the Modbus TCP Module. Please note that frame processing is still disabled until eMBEnable( ) is called.
- Parameters:
-
usTCPPort The TCP port to listen on.
- Returns:
- If the protocol stack has been initialized correctly the function returns eMBErrorCode::MB_ENOERR. Otherwise one of the following error codes is returned:
- eMBErrorCode::MB_EINVAL If the slave address was not valid. Valid slave addresses are in the range 1 - 247.
- eMBErrorCode::MB_EPORTERR IF the porting layer returned an error.
Generated on Tue Jul 12 2022 19:17:06 by
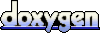