Ghz2000's 14Segment LED Display Library
Dependents: Nucleo_Seg14Display
lib14Seg.hpp
00001 /* Copyright (c) 2016 Ghz2000, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 00020 00021 00022 #ifndef _lib14Seg_ 00023 #define _lib14Seg_ 00024 00025 #include "mbed.h" 00026 00027 00028 //correct 00029 #define sign_A 0x4791 00030 #define sign_B 0x13B4 00031 #define sign_C 0x4221 00032 #define sign_D 0x13A4 00033 #define sign_E 0x4631 00034 #define sign_F 0x4611 00035 #define sign_G 0x42B1 00036 #define sign_H 0x4591 00037 #define sign_I 0x1224 00038 #define sign_J 0x01A1 00039 #define sign_K 0x4C09 00040 #define sign_L 0x4021 00041 #define sign_M 0x6981 00042 #define sign_N 0x6189 00043 #define sign_O 0x43A1 00044 #define sign_P 0x4711 00045 #define sign_Q 0x43A9 00046 #define sign_R 0x4719 00047 #define sign_S 0x46B0 00048 #define sign_T 0x1204 00049 #define sign_U 0x41A1 00050 #define sign_V 0x4803 00051 #define sign_W 0x418B 00052 #define sign_X 0x280A 00053 #define sign_Y 0x2804 00054 #define sign_Z 0x0A22 00055 00056 //incorrect 00057 #define sign_Exclamation 0x01C0 00058 #define sign_Wquot 0x1100 00059 #define sign_Sharp 0x1594 00060 #define sign_Doll 0x56B4 00061 #define sign_Percent 0x4882 00062 #define sign_And 0x3629 00063 #define sign_Quot 0x0800 00064 #define sign_RoundBracketS 0x0818 00065 #define sign_RoundBracketE 0x2402 00066 #define sign_Asta 0x3C1E 00067 #define sign_Plus 0x1414 00068 #define sign_Comma 0x0002 00069 #define sign_Minus 0x0410 00070 #define sign_Slash 0x0802 00071 #define sign_Colon 0x1004 00072 #define sign_SemiColon 0x1002 00073 #define sign_AngleS 0x0808 00074 #define sign_Equal 0x0430 00075 #define sign_AngleE 0x2002 00076 #define sign_Question 0x4314 00077 #define sign_At 0x5331 00078 #define sign_BoxS 0x2222 00079 #define sign_En 0x2C14 00080 #define sign_BoxE 0xA28 00081 #define sign_Hat 0x0900 00082 #define sign_Underbar 0x0020 00083 #define sign_Grave 0x2000 00084 #define sign_CurlyBracketS 0x2622 00085 #define sign_CurlyBracketE 0x0A38 00086 #define sign_Tate 0x4001 00087 #define sign_Child 0x0200 00088 00089 00090 #define sign_a 0x07B1 00091 #define sign_b 0x44B1 00092 #define sign_c 0x0431 00093 #define sign_d 0x05B1 00094 #define sign_e 0x0423 00095 #define sign_f 0x4601 00096 #define sign_g 0x23B0 00097 #define sign_h 0x4405 00098 #define sign_i 0x0004 00099 #define sign_j 0x01A0 00100 #define sign_k 0x180C 00101 #define sign_l 0x1004 00102 #define sign_m 0x0495 00103 #define sign_n 0x0405 00104 #define sign_o 0x04B1 00105 #define sign_p 0x4E01 00106 #define sign_q 0x2390 00107 #define sign_r 0x0401 00108 #define sign_s 0x0038 00109 #define sign_t 0x4421 00110 #define sign_u 0x00A1 00111 #define sign_v 0x0003 00112 #define sign_w 0x008B 00113 #define sign_x 0x280A 00114 #define sign_y 0x11B0 00115 #define sign_z 0x0422 00116 00117 #define sign_0 0x43A1 00118 #define sign_1 0x0980 00119 #define sign_2 0x0731 00120 #define sign_3 0x07B0 00121 #define sign_4 0x4590 00122 #define sign_5 0x46B0 00123 #define sign_6 0x46B1 00124 #define sign_7 0x4380 00125 #define sign_8 0x47B1 00126 #define sign_9 0x4790 00127 00128 #define sign_dot 0x0040 00129 00130 #define sign_ 0x0000 00131 00132 //#define sign_ 0x 00133 // 0x8000 表示用終端文字 00134 00135 00136 #define __LEN 30 00137 00138 /** Ghz2000 LED 14Segment Display 00139 * 00140 * http://ghz2000.dip.jp/wordpress/?p=665 00141 * 00142 * LED 14セグ表示器用ライブラリです。 00143 * 00144 * Used for showing Alphanumelic LED 14 Segment Display. 00145 * This library comment was written in Japanese. 00146 * The reason why for Japanese beginner. 00147 * 00148 * @attention 00149 * このバージョンでは8桁のみ動作します。カスケードできません。 00150 * また輝度も使用できません。 00151 * 00152 * Example: 00153 * @code 00154 * #include "mbed.h" 00155 * #include "lib14Seg.hpp" 00156 * 00157 * int main(){ 00158 * LED14Seg.init(SPI_MOSI, SPI_MISO, SPI_SCK, RCK, 0x04, 8); 00159 * 00160 * LED14Seg.setChar("ABCDEFGHIJKLMNOPQRSTUVWXYZ"); 00161 * LED14Seg.showStop(); 00162 * LED14Seg.setScrollSpeed(300); 00163 * while(1){}; 00164 * } 00165 * @endcode 00166 */ 00167 class C14Segment 00168 { 00169 private: 00170 uint16_t m_view[8]; 00171 uint16_t m_str[__LEN]; 00172 static const uint16_t ascii[]; 00173 PinName m_RCK; 00174 PinName m_MOSI; 00175 PinName m_MISO; 00176 PinName m_SCK; 00177 int m_kido; 00178 int m_ViewLength; 00179 int m_speed; 00180 00181 SPI *mp_SPIdevice; 00182 DigitalOut *mp_DigitalOutRCK; 00183 00184 void setSPI(PinName MOSI, PinName MISO, PinName SCK, PinName RCK); 00185 void setViewLength(int ViewLength); 00186 00187 public: 00188 C14Segment(); 00189 00190 /** イニシャライザ 最初に実行してください。 00191 * 00192 * @note 00193 * 輝度は現在使えません。 Lengthは現在8のみです。 00194 */ 00195 void init( PinName SPI_MOSI, //!< MOSI 00196 PinName SPI_MISO, //!< MISO 接続不要 00197 PinName SPI_SCK, //!< SCK 00198 PinName RCK, //!< Latch 00199 int kido, //!< 現在使えません 00200 int ViewLength //!< 8桁のみ 00201 ); 00202 void setChar(char *); //!< 文字列をセットします。 30文字まで 00203 void showStop(); //!< 格納した文字列を表示する 00204 void scroll(); //!< 格納した文字列をスクロール表示する 00205 void setScrollSpeed(int scrollSpeed); //!< スクロールスピードを設定する 00206 00207 void update(); 00208 00209 void setBlight(int kido); //4桁モデルのみで有効 00210 void putnum(); //未実装 00211 void cls(); //未実装 00212 void showCycleX(); //未実装 00213 void showCycleO(); //未実装 00214 00215 }; 00216 00217 extern C14Segment LED14Seg; 00218 00219 #endif
Generated on Wed Jul 13 2022 12:05:20 by
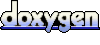