Full graphic 640x400 monochrome VGA driver library
Embed:
(wiki syntax)
Show/hide line numbers
vga640x400g_functions.h
00001 #include "vga_font.h" 00002 #define FRAMEBUFFERMAX 32000 00003 #define VGA_HEIGHT 400 00004 #define VGA_WIDTH 640 00005 #include "mbed.h" 00006 00007 extern unsigned char *framebuffer; 00008 00009 static const unsigned char rmask[]={0x7f,0xbf,0xdf,0xef,0xf7,0xfb,0xfd,0xfe}; 00010 static const unsigned char smask[]={0x80,0x40,0x20,0x10,0x08,0x04,0x02,0x01}; 00011 00012 // clear the screen 00013 void vga_cls() { 00014 memset(framebuffer,0,FRAMEBUFFERMAX); 00015 } 00016 00017 // scroll the screen one text line up 00018 void vga_scroll() { 00019 memcpy(framebuffer,framebuffer+(FONTHEIGHT*80),FRAMEBUFFERMAX-80*(FONTHEIGHT)); 00020 memset(framebuffer+FRAMEBUFFERMAX-80*(FONTHEIGHT),0,FONTHEIGHT*80); 00021 } 00022 00023 // set or reset a single pixel on screen 00024 void vga_plot(int x,int y,char color) { 00025 unsigned int ad; 00026 00027 ad=80*y+((x/8)^1); // calculate offset 00028 if (ad>=FRAMEBUFFERMAX) return; // crude clip 00029 framebuffer[ad]&=rmask[x%8]; // clear the bit 00030 if (color) framebuffer[ad]|=smask[x%8]; // if color != 0 set the bit 00031 } 00032 00033 // put a character from the vga font on screen 00034 void vga_putchar(int x,int y,int ch, char color) { 00035 int n,m; 00036 for (n=0; n<FONTHEIGHT; n++) { 00037 for (m=0; m<FONTWIDTH; m++) { 00038 if (vga_font8x16[ch*FONTHEIGHT+n] & (1<<(7-m))) vga_plot(x+m,y+n, color); 00039 else vga_plot(x+m,y+n,0); 00040 } 00041 } 00042 } 00043 00044 // put a string on screen 00045 void vga_putstring(int x,int y, char *s, char color) { 00046 while (*s) { 00047 vga_putchar(x,y,*(s++),color); 00048 x+=FONTWIDTH; 00049 } 00050 } 00051 00052 // Bresenham line routine 00053 void vga_line(int x0, int y0, int x1, int y1,char color) { 00054 00055 char steep=1; 00056 int li,dx,dy,le; 00057 int sx,sy; 00058 00059 // if same coordinates 00060 if (x0==x1 && y0==y1) { 00061 vga_plot(x0,y0,color); 00062 return; 00063 } 00064 00065 dx = abs(x1-x0); 00066 sx = ((x1 - x0) >0) ? 1 : -1; 00067 dy=abs(y1-y0); 00068 sy = ((y1 - y0) >0) ? 1 : -1; 00069 00070 if (dy > dx) { 00071 steep=0; 00072 // swap X0 and Y0 00073 x0=x0 ^ y0; 00074 y0=x0 ^ y0; 00075 x0=x0 ^ y0; 00076 00077 // swap DX and DY 00078 dx=dx ^ dy; 00079 dy=dx ^ dy; 00080 dx=dx ^ dy; 00081 00082 // swap SX and SY 00083 sx=sx ^ sy; 00084 sy=sx ^ sy; 00085 sx=sx ^ sy; 00086 } 00087 00088 le = (dy << 1) - dx; 00089 00090 for (li=0; li<=dx; li++) { 00091 if (steep) { 00092 vga_plot(x0,y0,color); 00093 } else { 00094 vga_plot(y0,x0,color); 00095 } 00096 while (le >= 0) { 00097 y0 += sy; 00098 le -= (dx << 1); 00099 } 00100 x0 += sx; 00101 le += (dy << 1); 00102 } 00103 } 00104 00105 // draw an outline box 00106 void vga_box(int x0, int y0, int x1, int y1,char color) { 00107 vga_line(x0,y0,x1,y0,color); 00108 vga_line(x1,y0,x1,y1,color); 00109 vga_line(x1,y1,x0,y1,color); 00110 vga_line(x0,y1,x0,y0,color); 00111 } 00112 00113 // draw a filled box 00114 void vga_filledbox(int x0, int y0, int x1, int y1,char color) { 00115 int n,dn; 00116 00117 if (y1>y0) dn=1; 00118 else dn=-1; 00119 00120 for (n=abs(y1-y0); n>=0; n--) { 00121 vga_line(x0,y0,x1,y0,color); 00122 y0+=dn; 00123 } 00124 } 00125 00126 // Bresenham circle routine 00127 void vga_circle(int x0,int y0, int radius, char color) { 00128 00129 int cf = 1 - radius; 00130 int dx = 1; 00131 int dy = -2 * radius; 00132 int cx = 0; 00133 int py = radius; 00134 00135 vga_plot(x0, y0 + radius,color); 00136 vga_plot(x0, y0 - radius,color); 00137 vga_plot(x0 + radius, y0,color); 00138 vga_plot(x0 - radius, y0,color); 00139 00140 while (cx < py) { 00141 if (cf >= 0) { 00142 py--; 00143 dy += 2; 00144 cf += dy; 00145 } 00146 cx++; 00147 dx += 2; 00148 cf += dx; 00149 vga_plot(x0 + cx, y0 + py,color); 00150 vga_plot(x0 - cx, y0 + py,color); 00151 vga_plot(x0 + cx, y0 - py,color); 00152 vga_plot(x0 - cx, y0 - py,color); 00153 vga_plot(x0 + py, y0 + cx,color); 00154 vga_plot(x0 - py, y0 + cx,color); 00155 vga_plot(x0 + py, y0 - cx,color); 00156 vga_plot(x0 - py, y0 - cx,color); 00157 } 00158 }
Generated on Tue Jul 19 2022 20:06:41 by
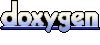