Object for MX28 with rs485
Embed:
(wiki syntax)
Show/hide line numbers
Mx28.h
00001 /* 00002 How Dynamixel work can be found 00003 -------------------------------- 00004 Robotis e-Manual 00005 http://support.robotis.com 00006 00007 Overview of Communication 00008 http://support.robotis.com/en/product/dynamixel/dxl_communication.htm 00009 00010 Kind of Instruction 00011 http://support.robotis.com/en/product/dynamixel/communication/dxl_instruction.htm 00012 00013 Instruction Packet & Status Packet (Return Packet) 00014 http://support.robotis.com/en/product/dynamixel/communication/dxl_packet.htm 00015 00016 Control Table 00017 http://support.robotis.com/en/product/dynamixel/mx_series/mx-28.htm 00018 00019 */ 00020 00021 #ifndef Mx28_h 00022 #define Mx28_h 00023 00024 //------------------------------------------------------------------------------------------------------------------------------- 00025 // define - Dynamixel Hex code table 00026 //------------------------------------------------------------------------------------------------------------------------------- 00027 // EEPROM AREA 00028 #define EEPROM_MODEL_NUMBER_L 0x00 00029 #define EEPROM_MODEL_NUMBER_H 0x01 00030 #define EEPROM_VERSION 0x02 00031 #define EEPROM_ID 0x03 00032 #define EEPROM_BAUD_RATE 0x04 00033 #define EEPROM_RETURN_DELAY_TIME 0x05 00034 #define EEPROM_CW_ANGLE_LIMIT_L 0x06 00035 #define EEPROM_CW_ANGLE_LIMIT_H 0x07 00036 #define EEPROM_CCW_ANGLE_LIMIT_L 0x08 00037 #define EEPROM_CCW_ANGLE_LIMIT_H 0x09 00038 #define EEPROM_LIMIT_TEMPERATURE 0x0B 00039 #define EEPROM_LOW_LIMIT_VOLTAGE 0x0C 00040 #define EEPROM_HIGN_LIMIT_VOLTAGE 0x0D 00041 #define EEPROM_MAX_TORQUE_L 0x0E 00042 #define EEPROM_MAX_TORQUE_H 0x0F 00043 #define EEPROM_RETURN_LEVEL 0x10 00044 #define EEPROM_ALARM_LED 0x11 00045 #define EEPROM_ALARM_SHUTDOWN 0x12 00046 // RAM AREA 00047 #define RAM_TORQUE_ENABLE 0x18 00048 #define RAM_LED 0x19 00049 #define RAM_PROPORTIONAL_GAIN 0x1A 00050 #define RAM_INTERGRAL_GAIN 0x1B 00051 #define RAM_DERIVATIVE_GAIN 0x1C 00052 #define RAM_GOAL_POSITION_L 0x1E 00053 #define RAM_GOAL_POSITION_H 0x1F 00054 #define RAM_GOAL_SPEED_L 0x20 00055 #define RAM_GOAL_SPEED_H 0x21 00056 #define RAM_TORQUE_LIMIT_L 0x22 00057 #define RAM_TORQUE_LIMIT_H 0x23 00058 #define RAM_PRESENT_POSITION_L 0x24 00059 #define RAM_PRESENT_POSITION_H 0x25 00060 #define RAM_PRESENT_SPEED_L 0x26 00061 #define RAM_PRESENT_SPEED_H 0x27 00062 #define RAM_PRESENT_LOAD_L 0x28 00063 #define RAM_PRESENT_LOAD_H 0x29 00064 #define RAM_PRESENT_VOLTAGE 0x2A 00065 #define RAM_PRESENT_TEMPERATURE 0x2B 00066 #define RAM_REGISTER 0x2C 00067 #define RAM_MOVING 0x2E 00068 #define RAM_LOCK 0x2F 00069 #define RAM_PUNCH_L 0x30 00070 #define RAM_PUNCH_H 0x31 00071 00072 00073 //------------------------------------------------------------------------------------------------------------------------------- 00074 // Instruction commands Set 00075 //------------------------------------------------------------------------------------------------------------------------------- 00076 #define COMMAND_PING 0x01 00077 #define COMMAND_READ_DATA 0x02 00078 #define COMMAND_WRITE_DATA 0x03 00079 #define COMMAND_REG_WRITE_DATA 0x04 00080 #define COMMAND_ACTION 0x05 00081 #define COMMAND_RESET 0x06 00082 #define COMMAND_SYNC_WRITE 0x83 00083 00084 00085 //------------------------------------------------------------------------------------------------------------------------------- 00086 //Instruction packet lengths 00087 #define READ_ONE_BYTE_LENGTH 0x01 00088 #define READ_TWO_BYTE_LENGTH 0x02 00089 #define RESET_LENGTH 0x02 00090 #define PING_LENGTH 0x02 00091 #define ACTION_LENGTH 0x02 00092 #define SET_ID_LENGTH 0x04 00093 #define SET_BD_LENGTH 0x04 00094 #define SET_RETURN_LEVEL_LENGTH 0x04 00095 #define READ_TEMP_LENGTH 0x04 00096 #define READ_POS_LENGTH 0x04 00097 #define READ_LOAD_LENGTH 0x04 00098 #define READ_SPEED_LENGTH 0x04 00099 #define READ_VOLT_LENGTH 0x04 00100 #define READ_REGISTER_LENGTH 0x04 00101 #define READ_MOVING_LENGTH 0x04 00102 #define READ_LOCK_LENGTH 0x04 00103 #define LED_LENGTH 0x04 00104 #define SET_HOLDING_TORQUE_LENGTH 0x04 00105 #define SET_MAX_TORQUE_LENGTH 0x05 00106 #define SET_ALARM_LENGTH 0x04 00107 #define READ_LOAD_LENGTH 0x04 00108 #define SET_RETURN_LENGTH 0x04 00109 #define WHEEL_LENGTH 0x05 00110 #define SERVO_GOAL_LENGTH 0x07 00111 #define SET_MODE_LENGTH 0x07 00112 #define SET_PUNCH_LENGTH 0x04 00113 #define SET_PID_LENGTH 0x06 00114 #define SET_TEMP_LENGTH 0x04 00115 #define SET_VOLT_LENGTH 0x05 00116 #define SYNC_LOAD_LENGTH 0x0D 00117 #define SYNC_DATA_LENGTH 0x02 00118 00119 00120 //------------------------------------------------------------------------------------------------------------------------------- 00121 // Specials 00122 //------------------------------------------------------------------------------------------------------------------------------- 00123 00124 #define OFF 0x00 00125 #define ON 0x01 00126 00127 #define SERVO 0x01 00128 #define WHEEL 0x00 00129 00130 #define LEFT 0x00 00131 #define RIGHT 0x01 00132 00133 #define NONE 0x00 00134 #define READ 0x01 00135 #define ALL 0x02 00136 00137 #define BROADCAST_ID 0xFE 00138 00139 #define HEADER 0xFF 00140 00141 #define STATUS_PACKET_TIMEOUT 50 // in millis() 00142 #define STATUS_FRAME_BUFFER 5 00143 00144 00145 00146 class DynamixelClass { 00147 private: 00148 DigitalOut *servoSerialDir; 00149 Serial *servoSerial; 00150 void transmitInstructionPacket(void); 00151 unsigned int readStatusPacket(void); 00152 bool overflow; 00153 void debugframe(void); 00154 void debugStatusframe(void); 00155 DigitalOut *led2; 00156 public: 00157 00158 DynamixelClass(int baud,PinName D_Pin,PinName tx, PinName rx); //Constructor 00159 ~DynamixelClass(void); //destruktor 00160 00161 void NewBaudRate(int baud); 00162 unsigned int reset(unsigned char); 00163 unsigned int ping(unsigned char); 00164 00165 unsigned int setStatusPaketReturnDelay(unsigned char,unsigned char); 00166 unsigned int setID(unsigned char, unsigned char); 00167 unsigned int setBaudRate(unsigned char, long); 00168 unsigned int setMaxTorque(unsigned char, int); 00169 unsigned int setHoldingTorque(unsigned char, bool); 00170 unsigned int setAlarmShutdown(unsigned char,unsigned char); 00171 unsigned int setStatusPaket(unsigned char,unsigned char); 00172 unsigned int setMode(unsigned char, bool, unsigned int, unsigned int); 00173 unsigned int setPunch(unsigned char,unsigned int); 00174 unsigned int setPID(unsigned char,unsigned char,unsigned char,unsigned char); 00175 unsigned int setTemp(unsigned char,unsigned char); 00176 unsigned int setVoltage(unsigned char,unsigned char,unsigned char); 00177 00178 unsigned int servo(unsigned char, unsigned int, unsigned int); 00179 unsigned int servoPreload(unsigned char, unsigned int, unsigned int); 00180 unsigned int wheel(unsigned char, bool, unsigned int); 00181 void wheelSync(unsigned char,bool,unsigned int,unsigned char, bool,unsigned int,unsigned char, bool,unsigned int); 00182 unsigned int wheelPreload(unsigned char, bool, unsigned int); 00183 00184 unsigned int action(unsigned char); 00185 00186 unsigned int readTemperature(unsigned char); 00187 unsigned int readVoltage(unsigned char); 00188 unsigned int readPosition(unsigned char); 00189 unsigned int readLoad(unsigned char); 00190 unsigned int readSpeed(unsigned char); 00191 00192 unsigned int checkRegister(unsigned char); 00193 unsigned int checkMovement(unsigned char); 00194 unsigned int checkLock(unsigned char); 00195 00196 unsigned int ledState(unsigned char, bool); 00197 }; 00198 00199 #endif
Generated on Sat Jul 16 2022 20:47:13 by
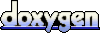