Libery for HX711 sensor. This is for measureing the weigth of the keg
Embed:
(wiki syntax)
Show/hide line numbers
Hx711.h
00001 #ifndef HX711_h 00002 #define HX711_h 00003 //Translatet module from arduino to mbed https://codebender.cc/library/HX711#HX711.h 00004 00005 #include "mbed.h" 00006 00007 class HX711 00008 { 00009 private: 00010 DigitalOut _pin_slk; // Power Down and Serial Clock Input Pin 00011 DigitalIn _pin_dout; // Serial Data Output Pin 00012 char GAIN; // amplification factor 00013 long OFFSET; // used for tare weight 00014 float SCALE; // used to return weight in grams, kg, ounces, whatever 00015 public: 00016 // define clock and data pin, channel, and gain factor 00017 // channel selection is made by passing the appropriate gain: 128 or 64 for channel A, 32 for channel B 00018 // gain: 128 or 64 for channel A; channel B works with 32 gain factor only 00019 HX711(PinName pin_dout, PinName pin_slk); 00020 00021 virtual ~HX711(); 00022 00023 // check if HX711 is ready 00024 // from the datasheet: When output data is not ready for retrieval, digital output pin DOUT is high. Serial clock 00025 // input PD_SCK should be low. When DOUT goes to low, it indicates data is ready for retrieval. 00026 00027 00028 // set the gain factor; takes effect only after a call to read() 00029 // channel A can be set for a 128 or 64 gain; channel B has a fixed 32 gain 00030 // depending on the parameter, the channel is also set to either A or B 00031 void set_gain(char gain = 128); 00032 00033 // waits for the chip to be ready and returns a reading 00034 long read(); 00035 00036 // returns an average reading; times = how many times to read 00037 long read_average(char times = 10); 00038 00039 // returns (read_average() - OFFSET), that is the current value without the tare weight; times = how many readings to do 00040 double get_value(char times = 1); 00041 00042 // returns get_value() divided by SCALE, that is the raw value divided by a value obtained via calibration 00043 // times = how many readings to do 00044 float get_units(char times = 1); 00045 00046 // set the OFFSET value for tare weight; times = how many times to read the tare value 00047 void tare(char times = 10); 00048 00049 // set the SCALE value; this value is used to convert the raw data to "human readable" data (measure units) 00050 void set_scale(float scale = 1.f); 00051 00052 // set OFFSET, the value that's subtracted from the actual reading (tare weight) 00053 void set_offset(long offset = 0); 00054 00055 // puts the chip into power down mode 00056 void power_down(); 00057 00058 // wakes up the chip after power down mode 00059 void power_up(); 00060 }; 00061 00062 #endif /* HX711_h */
Generated on Wed Jul 20 2022 19:09:29 by
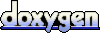