A simple fifo for data
Embed:
(wiki syntax)
Show/hide line numbers
fifo.cpp
00001 #include "fifo.h" 00002 00003 fifo::fifo() 00004 { 00005 this->head = 0; 00006 this->tail = 0; 00007 } 00008 uint32_t fifo::available() 00009 { 00010 return (FIFO_SIZE + this->head - this->tail) % FIFO_SIZE; 00011 } 00012 uint32_t fifo::free() 00013 { 00014 return (FIFO_SIZE - 1 - available()); 00015 } 00016 uint8_t fifo::put(FIFO_TYPE data) 00017 { 00018 uint32_t next; 00019 00020 // check if FIFO has room 00021 next = (this->head + 1) % FIFO_SIZE; 00022 if (next == this->tail) 00023 { 00024 // full 00025 return 1; 00026 } 00027 00028 this->buffer[this->head] = data; 00029 this->head = next; 00030 00031 return 0; 00032 } 00033 uint8_t fifo::get(FIFO_TYPE* data) 00034 { 00035 uint32_t next; 00036 00037 // check if FIFO has data 00038 if (this->head == this->tail) 00039 { 00040 return 1; // FIFO empty 00041 } 00042 00043 next = (this->tail + 1) % FIFO_SIZE; 00044 00045 *data = this->buffer[this->tail]; 00046 this->tail = next; 00047 00048 return 0; 00049 }
Generated on Wed Jul 13 2022 00:33:14 by
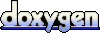