Modificación para implementar SoftSerial
Dependents: neurGAI_Seeed_BLUETOOTH TFG
Fork of BluetoothSerial by
BluetoothSerial.h
00001 /** 00002 * The library is for Bluetooth Shield from Seeed Studio 00003 */ 00004 00005 #ifndef __BLUETOOTH_SERIAL_H__ 00006 #define __BLUETOOTH_SERIAL_H__ 00007 00008 #include "mbed.h" 00009 #include "SoftSerial.h" 00010 00011 #define BLUETOOTH_SERIAL_DEFAULT_BAUD 38400 00012 #define BLUETOOTH_SERIAL_TIMEOUT 10000 00013 #define BLUETOOTH_SERIAL_EOL "\r\n" 00014 00015 00016 class BluetoothSerial : public Stream { 00017 public: 00018 BluetoothSerial(PinName tx, PinName rx); 00019 00020 /** 00021 * Setup bluetooth module(serial port baud rate) 00022 */ 00023 void setup(); 00024 00025 /** 00026 * Set bluetooth module as a master 00027 * \param name device name 00028 * \param autoc 1: auto-connection, 0 not 00029 */ 00030 void master(const char *name, uint8_t autoc = 0); 00031 00032 /** 00033 * Set bluetooth module as a slave 00034 * \param name device name 00035 * \param autoc 1: auto-connection, 0 not 00036 * \param oaut 1: permit paired device to connect, 0: not 00037 */ 00038 void slave(const char *name, uint8_t autoc = 0, uint8_t oaut = 1); 00039 00040 /** 00041 * Inquire bluetooth devices and connect the specified device 00042 */ 00043 int connect(const char *name); 00044 00045 /** 00046 * Make the bluetooth module inquirable and available to connect, used in slave mode 00047 */ 00048 int connect(); 00049 00050 int readable() { 00051 return _serial.readable(); 00052 } 00053 00054 int writeable() { 00055 return _serial.writeable(); 00056 } 00057 00058 00059 protected: 00060 virtual int _getc(); 00061 virtual int _putc(int c); 00062 00063 void clear(); 00064 int readline(uint8_t *buf, int len, uint32_t timeout = 0); 00065 00066 SoftSerial _serial; 00067 uint8_t _buf[64]; 00068 }; 00069 00070 #endif // __BLUETOOTH_SERIAL_H__
Generated on Thu Aug 4 2022 11:19:13 by
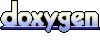