An mbed library for the Embedded Artists QVGA OLED 2.8 inch panel
EAQVGAOLED Class Reference
Uses SPI to drive the Embedded Artists 2.8" QVGA OLED panel. More...
#include <EAQVGAOLED.h>
Inherits GraphicsDisplay.
Public Member Functions | |
EAQVGAOLED (PinName mosi, PinName miso, PinName sclk, PinName cs, PinName reset, PinName bl) | |
The driver constructor. | |
virtual void | pixel (int x, int y, int colour) |
Set a pixel to the specified colour. | |
virtual int | width (void) |
Accessor to obtain the display width. | |
virtual int | height (void) |
Accessor to obtain the display height. | |
virtual void | cls () |
Clear the screen. | |
void | hLine (const uint16_t x0, const uint16_t y0, const uint16_t x1, const uint16_t colour) |
Draw a horizontal line. | |
void | vLine (const uint16_t x0, const uint16_t y0, const uint16_t y1, const uint16_t colour) |
Draw a vertical line. | |
void | rectangle (const uint16_t x0, const uint16_t y0, const uint16_t x1, const uint16_t y1, uint16_t colour) |
Draw a rectangle in the specified colour. | |
void | fillRectangle (const uint16_t x0, const uint16_t y0, const uint16_t x1, const uint16_t y1, uint16_t colour) |
Draw a filled rectangle in the specified colour. | |
void | backlightControl (bool on) |
Control the backlight. | |
Protected Member Functions | |
virtual int | _putc (int value) |
Print a character, overridden to handle control characters. |
Detailed Description
Uses SPI to drive the Embedded Artists 2.8" QVGA OLED panel.
- See also:
- http://mbed.org/cookbook/EAQVGAOLED
- example.cpp
- API
EAQVGAOLED defines a library to drive the Embedded Artists QVGA 2.8" OLED panel. Currently the library uses SPI to drive the panel.
Standard Example:
#include "mbed.h" #include "EAQVGAOLED.h" int main() { // Create an instance of the display driver EAQVGAOLED display = EAQVGAOLED(p5, p6, p7, p8, p9, p10); display.background(BLACK); display.foreground(DARK_GRAY); display.cls(); display.printf("Hello from mbed"); }
Definition at line 78 of file EAQVGAOLED.h.
Constructor & Destructor Documentation
EAQVGAOLED | ( | PinName | mosi, |
PinName | miso, | ||
PinName | sclk, | ||
PinName | cs, | ||
PinName | reset, | ||
PinName | bl | ||
) |
The driver constructor.
Create an EA QVGA OLED object connected using the specified SPI port, and two output pins; the Chip Select pin and the Backlight pin.
- Parameters:
-
mosi SPI data output pin miso SPI data input pin sclk SPI Clock pin cs Pin to drive the display CS reset Pin to drive the display reset bl Pin to drive the display backlight
Definition at line 62 of file EAQVGAOLED.cpp.
Member Function Documentation
int _putc | ( | int | value ) | [protected, virtual] |
Print a character, overridden to handle control characters.
- Parameters:
-
value The character value to print
- Returns:
- The character value printed
Definition at line 186 of file EAQVGAOLED.cpp.
void backlightControl | ( | bool | on ) |
Control the backlight.
ingroup API
- Parameters:
-
on Enable the backlight
Definition at line 200 of file EAQVGAOLED.h.
virtual void cls | ( | ) | [virtual] |
Clear the screen.
Definition at line 129 of file EAQVGAOLED.h.
void fillRectangle | ( | const uint16_t | x0, |
const uint16_t | y0, | ||
const uint16_t | x1, | ||
const uint16_t | y1, | ||
uint16_t | colour | ||
) |
Draw a filled rectangle in the specified colour.
- Parameters:
-
x0 Starting x position y0 Starting y position x1 Ending x position y1 Ending y position colour Filled colour of rectangle
Definition at line 167 of file EAQVGAOLED.cpp.
virtual int height | ( | void | ) | [virtual] |
Accessor to obtain the display height.
- Returns:
- the height of the display in pixels
Definition at line 122 of file EAQVGAOLED.h.
void hLine | ( | const uint16_t | x0, |
const uint16_t | y0, | ||
const uint16_t | x1, | ||
const uint16_t | colour | ||
) |
Draw a horizontal line.
- Parameters:
-
x0 Starting x position y0 Starting y position x1 End x position colour Colour of line
Definition at line 92 of file EAQVGAOLED.cpp.
void pixel | ( | int | x, |
int | y, | ||
int | colour | ||
) | [virtual] |
Set a pixel to the specified colour.
- Parameters:
-
x Pixel x position y Pixel y position colour Pixel colour
Definition at line 79 of file EAQVGAOLED.cpp.
void rectangle | ( | const uint16_t | x0, |
const uint16_t | y0, | ||
const uint16_t | x1, | ||
const uint16_t | y1, | ||
uint16_t | colour | ||
) |
Draw a rectangle in the specified colour.
- Parameters:
-
x0 Starting x position y0 Starting y position x1 Ending x position y1 Ending y position colour Outline colour of rectangle
Definition at line 147 of file EAQVGAOLED.cpp.
void vLine | ( | const uint16_t | x0, |
const uint16_t | y0, | ||
const uint16_t | y1, | ||
const uint16_t | colour | ||
) |
Draw a vertical line.
- Parameters:
-
x0 Starting x position y0 Starting y position y1 End y position colour Colour of line
Definition at line 119 of file EAQVGAOLED.cpp.
virtual int width | ( | void | ) | [virtual] |
Accessor to obtain the display width.
- Returns:
- the width of the display in pixels
Definition at line 115 of file EAQVGAOLED.h.
Generated on Mon Jul 18 2022 01:01:25 by
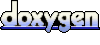