
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 00002 * Honeywell Pressure Sensor 00003 * 00004 */ 00005 00006 #include <math.h> 00007 00008 #include "mbed.h" 00009 00010 DigitalOut myled(LED1); 00011 00012 // http://sensing.honeywell.com/index.cfm/ci_id/157750/la_id/1/document/1/re_id/0 00013 // http://sensing.honeywell.com/index.cfm/ci_id/156988/la_id/1/document/1/re_id/0 00014 00015 #define HSC_2A 0x28 00016 00017 int main() { 00018 00019 // BMP085 bmp085(p28, p27); //bmp085(p9, p10); // sda, scl 00020 00021 printf("\rhsc\n\r"); 00022 00023 I2C i2c(p28, p27); 00024 00025 int id = HSC_2A; 00026 00027 i2c.frequency(100000); // 100k - 400k 00028 00029 while(1) { 00030 00031 #if 0 00032 i2c.start(); 00033 i2c.write((id<<1) | 1); 00034 int b1 = i2c.read(1); 00035 int b2 = i2c.read(1); 00036 int t1 = i2c.read(1); 00037 int t2 = i2c.read(0); 00038 i2c.stop(); 00039 printf("%02X %02X %02X %02X\n\r", b1, b2, t1, t2); 00040 #else 00041 unsigned char data[4]; 00042 i2c.read((id<<1)|1, (char*)data, sizeof(data)); 00043 //printf("%02X %02X %02X %02X\n\r", data[0], data[1], data[2], data[3]); 00044 00045 int st = data[0]>>6; // status 00046 int bd = ((data[0] & 0x3f) << 8) | data[1]; // bridge data 00047 int td = ((data[2]<<8) | data[3]) >> 5; // temp data 00048 00049 // P: 14 bits 00050 // Cmin=2^14-1*.1 Cmax=2^14-1*.9 00051 // Pmin=0 Pmax=15 00052 float p = (((float)bd-1638)*(15-0)/(14745-1638))+0; // convert raw data to psi 00053 p = p * 68.9475729; // convert psi to hPa 00054 00055 // T: 11 bits (Cmax=2047=2^11-1) 00056 float t = (((float)td*200)/2047)-50; // convert raw data to deg C 00057 printf("%d: %.2f hPa %.2f C\n\r", st, p, t); 00058 00059 wait(1); 00060 #endif 00061 00062 myled = 1; 00063 wait(0.2); 00064 myled = 0; 00065 wait(0.2); 00066 00067 } // while 00068 00069 } // main 00070 00071 00072 // http://en.wikipedia.org/wiki/Barometric_formula 00073 const float layers[][4] = { 00074 // Height[m], Pressure[Pa], Temp[K], Lapse[K/m], Subscript 00075 0, 101325.0, 288.15, -0.0065, // 0 00076 11000, 22632.1, 216.65, 0.0, // 1 00077 20000, 5474.89, 216.65, 0.001, // 2 00078 32000, 868.019, 228.65, 0.0028, // 3 00079 47000, 110.906, 270.65, 0.0, // 4 00080 51000, 66.9389, 270.65, -0.0028, // 5 00081 71000, 3.95642, 214.65, -0.002 // 6 00082 }; 00083 00084 enum { L_HEIGHT=0, L_PRESS, L_TEMP, L_LAPSE }; 00085 00086 const float g = 9.80665; 00087 const float M = 0.0289644; 00088 const float R = 8.31432; 00089 const float Ta = -273.15; // Absolute zero [deg C] 00090 00091 // Search for subscript which matches pressure range 00092 // Choose appropriate formula and plug in values 00093 // Lookup derived h in table and check subscript is still same, otherwise recalculate 00094 // Add height correction 00095 00096 // Pass in pressure and temperature from sensor 00097 // Return height[m] 00098 float pressureToHeight(float p, float t) { 00099 float h = -999; // error 00100 int b; 00101 int tries; 00102 00103 // Search backwards thru layers for our pressure 00104 for(b = 6; b >= 0; --b) { 00105 if(p <= layers[b][1]) break; 00106 } 00107 if(b < 0) b = 0; // adjust so can have -ve heights 00108 int b1 = b; 00109 00110 // Only retry limited times 00111 for(tries = 10; tries; --tries) { 00112 00113 float Hb = layers[b][0]; 00114 float Pb = layers[b][1]; 00115 float Tb = layers[b][2]; //??? Tb = t - Ta; 00116 float Lb = layers[b][3]; 00117 00118 if(Lb != 0.0) 00119 //h = (log(g*M/R/Lb)/log(p/Pb)*Tb - Tb)/Lb + Hb; // Equation 1 (non-zero lapse rate) 00120 h = (Tb/exp(R*Lb/g/M*log(p/Pb))-Tb)/Lb + Hb; 00121 else 00122 h = -log(p/Pb)*R*Tb/g/M + Hb; // Equation 2 (zero lapse rate) 00123 00124 // Search again for derived height 00125 for(b = 6; b >= 0; --b) { 00126 if(h <= layers[b][0]) break; 00127 } 00128 00129 if(b == b1) break; // gave same subscript, so we can stop 00130 b1 = b; 00131 00132 } // for - tries 00133 00134 if(tries <= 0) h = -999; // error 00135 00136 00137 return h; 00138 00139 } // pressureToHeight 00140 00141 /*end*/ 00142
Generated on Mon Jul 18 2022 10:30:10 by
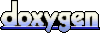