ROS Serial library for Mbed platforms for ROS Indigo Igloo. Check http://wiki.ros.org/rosserial_mbed/ for more information
Dependents: rosserial_mbed_hello_world_publisher rtos_base_control rosserial_mbed_F64MA ROS-RTOS ... more
SetPen.h
00001 #ifndef _ROS_SERVICE_SetPen_h 00002 #define _ROS_SERVICE_SetPen_h 00003 #include <stdint.h> 00004 #include <string.h> 00005 #include <stdlib.h> 00006 #include "ros/msg.h" 00007 00008 namespace turtlesim 00009 { 00010 00011 static const char SETPEN[] = "turtlesim/SetPen"; 00012 00013 class SetPenRequest : public ros::Msg 00014 { 00015 public: 00016 uint8_t r; 00017 uint8_t g; 00018 uint8_t b; 00019 uint8_t width; 00020 uint8_t off; 00021 00022 SetPenRequest(): 00023 r(0), 00024 g(0), 00025 b(0), 00026 width(0), 00027 off(0) 00028 { 00029 } 00030 00031 virtual int serialize(unsigned char *outbuffer) const 00032 { 00033 int offset = 0; 00034 *(outbuffer + offset + 0) = (this->r >> (8 * 0)) & 0xFF; 00035 offset += sizeof(this->r); 00036 *(outbuffer + offset + 0) = (this->g >> (8 * 0)) & 0xFF; 00037 offset += sizeof(this->g); 00038 *(outbuffer + offset + 0) = (this->b >> (8 * 0)) & 0xFF; 00039 offset += sizeof(this->b); 00040 *(outbuffer + offset + 0) = (this->width >> (8 * 0)) & 0xFF; 00041 offset += sizeof(this->width); 00042 *(outbuffer + offset + 0) = (this->off >> (8 * 0)) & 0xFF; 00043 offset += sizeof(this->off); 00044 return offset; 00045 } 00046 00047 virtual int deserialize(unsigned char *inbuffer) 00048 { 00049 int offset = 0; 00050 this->r = ((uint8_t) (*(inbuffer + offset))); 00051 offset += sizeof(this->r); 00052 this->g = ((uint8_t) (*(inbuffer + offset))); 00053 offset += sizeof(this->g); 00054 this->b = ((uint8_t) (*(inbuffer + offset))); 00055 offset += sizeof(this->b); 00056 this->width = ((uint8_t) (*(inbuffer + offset))); 00057 offset += sizeof(this->width); 00058 this->off = ((uint8_t) (*(inbuffer + offset))); 00059 offset += sizeof(this->off); 00060 return offset; 00061 } 00062 00063 const char * getType(){ return SETPEN; }; 00064 const char * getMD5(){ return "9f452acce566bf0c0954594f69a8e41b"; }; 00065 00066 }; 00067 00068 class SetPenResponse : public ros::Msg 00069 { 00070 public: 00071 00072 SetPenResponse() 00073 { 00074 } 00075 00076 virtual int serialize(unsigned char *outbuffer) const 00077 { 00078 int offset = 0; 00079 return offset; 00080 } 00081 00082 virtual int deserialize(unsigned char *inbuffer) 00083 { 00084 int offset = 0; 00085 return offset; 00086 } 00087 00088 const char * getType(){ return SETPEN; }; 00089 const char * getMD5(){ return "d41d8cd98f00b204e9800998ecf8427e"; }; 00090 00091 }; 00092 00093 class SetPen { 00094 public: 00095 typedef SetPenRequest Request; 00096 typedef SetPenResponse Response; 00097 }; 00098 00099 } 00100 #endif
Generated on Tue Jul 12 2022 18:39:41 by
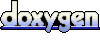