
Mine, not yours justin
Dependencies: HMC6352 USBHost mbed-rtos mbed
Fork of Project by
PositionSystem.h
00001 #include "USBHostMouse.h" 00002 #include "HMC6352.h" 00003 00004 #define PFLAG_ON 0 00005 #define PFLAG_OFF 1 00006 #define PFLAG_CALIB 2 00007 #define PTURN_SPEED (0.5) 00008 00009 float PTURN_RIGHT = 0; 00010 00011 extern Serial pc(USBTX, USBRX); 00012 00013 // updates xy position if on, does nothing if off 00014 extern char PFlag = PFLAG_ON; 00015 00016 // whenever a turn is complete, this should store 00017 // the degrees facing (0, 90, 180, 270) in system 00018 extern int t_position = 0; 00019 00020 // variables to keep track of coordinate position 00021 float x_position = 0; 00022 float y_position = 0; 00023 00024 // variables to keep track of movement during turning 00025 float pturn_x = 0; 00026 float pturn_y = 0; 00027 00028 /* mouse event handler */ 00029 void onMouseEvent(uint8_t buttons, int8_t x_mickey, int8_t y_mickey, int8_t z) { 00030 00031 // calculate new position 00032 if(PFlag == PFLAG_ON) { 00033 00034 // mouse movements are in mickeys. 1 mickey = ~230 DPI = ~1/230th of an inch 00035 float temp = y_mickey / 232.6; 00036 00037 // determine direction we are facing and add to that direction 00038 if(t_position == 0) 00039 y_position += temp; 00040 else if(t_position == 90) 00041 x_position += temp; 00042 else if(t_position == 180) 00043 y_position -= temp; 00044 else 00045 x_position -= temp; 00046 } else if(PFlag == PFLAG_CALIB) { 00047 PTURN_RIGHT += x_mickey / 232.6; 00048 } else { 00049 pturn_x += x_mickey / 232.6; 00050 pturn_y += y_mickey / 232.6; 00051 } 00052 } 00053 00054 // intialize x and y variables for turning 00055 void turnInit() { 00056 pturn_x = 0; 00057 pturn_y = 0; 00058 } 00059 00060 /* positioning system thread function */ 00061 void PositionSystemMain(void const *) { 00062 00063 USBHostMouse mouse; 00064 00065 while(1) { 00066 // try to connect a USB mouse 00067 while(!mouse.connect()) 00068 Thread::wait(500); 00069 00070 // when connected, attach handler called on mouse event 00071 mouse.attachEvent(onMouseEvent); 00072 00073 // wait until the mouse is disconnected 00074 while(mouse.connected()) 00075 Thread::wait(500); 00076 } 00077 }
Generated on Thu Jul 14 2022 19:48:39 by
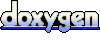