
Simple Thing for DJ
Dependencies: WIZnet_Library mbed
Fork of SimpleThing_DJ by
main.cpp
00001 #include "mbed.h" 00002 #include "WIZnetInterface.h" 00003 00004 unsigned char MAC_Addr[6] = {0x00,0x08,0xDC,0x12,0x07,0x07}; 00005 00006 char* Public_Key = "g1WVqxqKqNtr2AVG6424TVvoyopy"; 00007 char* Private_Key = "dRbwA0AeAWf7DqyMOdDdHzlA9Av9"; 00008 char* ServerIP = "192.168.44.70"; 00009 int Count = 15; 00010 00011 Serial pc(USBTX, USBRX); 00012 SPI spi(PTD2,PTD3,PTD1); 00013 WIZnetInterface ethernet(&spi,PTD0,PTA20); 00014 AnalogIn temp(PTC1); 00015 00016 00017 int main() 00018 { 00019 //Set serial port baudrate speed: 115200 00020 pc.baud(115200); 00021 pc.printf("Start\r\n"); 00022 00023 while(1) { 00024 int ret = ethernet.init(MAC_Addr); 00025 00026 if (!ret) { 00027 pc.printf("Initialized, MAC: %s\r\n", ethernet.getMACAddress()); 00028 ret = ethernet.connect(); 00029 if (!ret) { 00030 pc.printf("IP: %s, MASK: %s, GW: %s\r\n", 00031 ethernet.getIPAddress(), ethernet.getNetworkMask(), ethernet.getGateway()); 00032 } else { 00033 pc.printf("Error ethernet.connect() - ret = %d\r\n", ret); 00034 exit(0); 00035 } 00036 } else { 00037 pc.printf("Error ethernet.init() - ret = %d\r\n", ret); 00038 exit(0); 00039 } 00040 00041 TCPSocketConnection sock; 00042 sock.connect(ServerIP, 8080); 00043 if(sock.is_connected()) 00044 printf("Socket Connected\n\r"); 00045 else 00046 printf("Socket NoT Connected\n\r"); 00047 00048 char buffer[300]; 00049 int ret_t; 00050 00051 char http_cmd[256]; 00052 00053 sprintf(http_cmd,"GET /input/%s?private_key=%s&counter=%d HTTP/1.0\n\n",Public_Key,Private_Key,Count); 00054 printf("Running - %s\r\n",http_cmd); 00055 sock.send_all(http_cmd, sizeof(http_cmd)-1); 00056 00057 ret_t = sock.receive(buffer, sizeof(buffer)-1); 00058 buffer[ret_t] = '\0'; 00059 printf("Received %d chars from server:\n%s\r\n", ret_t, buffer); 00060 00061 sock.close(); 00062 00063 ethernet.disconnect(); 00064 printf("Socket Closed"); 00065 00066 while(1) {} 00067 }}
Generated on Tue Jul 12 2022 20:58:07 by
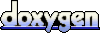