
test
Dependencies: PwmInRC USBDevice USBJoystick
main.cpp
00001 #include "mbed.h" 00002 #include "USBJoystick.h" 00003 00004 USBJoystick joystick; 00005 DigitalOut myled1(LED1); 00006 DigitalOut myled2(LED2); 00007 DigitalOut myled3(LED3); 00008 DigitalOut myled4(LED4); 00009 00010 InterruptIn mybutton(USER_BUTTON); 00011 00012 double tempo = 0.3; // LED blinking delay 00013 PinName pin = D7; 00014 // Change LEDs blinking frequency 00015 void change_blinking_frequency() { 00016 if (tempo == 0.3) // If leds have low frequency 00017 tempo = 0.1; // Set the fast frequency 00018 else // If leds have fast frequency 00019 tempo = 0.3; // Set the low frequency 00020 } 00021 00022 InterruptIn interrupt(pin); 00023 Timer timer; 00024 00025 uint32_t interval; 00026 uint32_t channels[9]; 00027 uint32_t channel; 00028 bool done=false; 00029 00030 void rise() 00031 { 00032 interval=timer.read_us(); 00033 if(interval > 3000) 00034 { 00035 done = true; 00036 channel=0; 00037 return; 00038 } 00039 int length = interval - 1000; 00040 if(length < 0) 00041 length = 0; 00042 else if(length>1023) 00043 length=1023; 00044 length /= 4; 00045 length -= 127; 00046 00047 channels[channel] = length; 00048 channel++; 00049 } 00050 00051 int main() { 00052 // All LEDs are OFF 00053 myled1 = 0; 00054 myled2 = 0; 00055 myled3 = 0; 00056 myled4 = 0; 00057 00058 // Change LEDs blinking frequency when button is pressed 00059 mybutton.fall(&change_blinking_frequency); 00060 timer.start(); 00061 interrupt.rise(&rise); 00062 // interrupt.fall(this, &RadioChannel::fall); 00063 uint8_t count=0; 00064 while(1) { 00065 if(done) 00066 { 00067 joystick.update(count, 0, channels[0], 0, 0, 0); 00068 done = false; 00069 } 00070 joystick.update(count, 0, channels[0], 0, 0, 0); 00071 count++; 00072 wait(0.001); 00073 } 00074 }
Generated on Fri Aug 19 2022 21:02:26 by
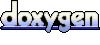