
This program is an example of an attempt at a Real Time Clock on the nRF51-DK.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 00002 This example code shows one way of creating a real time clock for the nRF51 without interfering with the mbed library functions 00003 00004 Two methods are created to replace the similarly named C routines: rtc.time and rtc.set_time. 00005 If the rtc.time method isn't called frequently (< 512 seconds between calls), a ticker (from the mbed library) is required 00006 to keep the time updated. 00007 00008 In this example, LED2 toggles and the time is printed when button1 is pushed. LED1 will toggle when the time is periodically updated. 00009 00010 references: 00011 -- see NRF_RTC_Type in nrf51.h 00012 (e.g.: http://developer.mbed.org/users/mbed_official/code/mbed-src/file/12a9a5f8fea0/targets/cmsis/TARGET_NORDIC/TARGET_MCU_NRF51822/nrf51.h) 00013 -- see "nRF51 Series Reference Manual" chapter on Real Time Counter (chapter 19 in V3.0) 00014 Note: RTC2 does not exist, despite what the nrf51 reference manual (V3.0) claims. The other RTCs (0/1) are used by various other libraries, 00015 so you can read, but don't write! 00016 00017 */ 00018 00019 #include "mbed.h" 00020 #include "nrf51_rtc.h" 00021 00022 // used for the example only, not required for rtc use 00023 DigitalOut rtc_update_led(LED1); // toggle when update_rtc is called 00024 DigitalOut button_push_led(LED2); // toggle when button1 is pushed 00025 DigitalIn button1(BUTTON1); // used to trigger the time report 00026 InterruptIn button1Press(BUTTON1); 00027 Serial pc(USBTX,USBRX); 00028 00029 time_t example_time() { 00030 // set an intial time 00031 // ...not really necessary for this example, but it beats setting it to 0 or some non-obvious large integer (# of seconds since 1/1/1970) 00032 time_t rawtime=0; 00033 00034 struct tm * init_timeinfo; 00035 00036 // initialize time 00037 init_timeinfo = localtime(&rawtime); // note: must initialize the struct with this before trying to set components 00038 // ...else code goes into the weeds!! 00039 init_timeinfo->tm_sec = 0; 00040 init_timeinfo->tm_min = 10; 00041 init_timeinfo->tm_hour = 17; 00042 init_timeinfo->tm_mon = 1; 00043 init_timeinfo->tm_mday = 7; 00044 init_timeinfo->tm_year = 2015 - 1900; 00045 00046 char date[24]; 00047 strftime(date,sizeof(date),"%H:%M:%S on %m/%d/%G",init_timeinfo); 00048 pc.printf("Initial time set is %s.\r\n",date); 00049 00050 // compute the proper value for time in time_t type 00051 rawtime = mktime(init_timeinfo); 00052 return rawtime; 00053 } 00054 void print_time() { 00055 // called when a button is pushed, this prints the current time to the USB-connected console 00056 button_push_led = !button_push_led; 00057 00058 time_t rawtime=rtc.time(); 00059 00060 // massage the time into a human-friendly format for printing 00061 struct tm * timeinfo; 00062 timeinfo = localtime(&rawtime); 00063 char date[24]; 00064 strftime(date,sizeof(date),"%H:%M:%S on %m/%d/%G",timeinfo); 00065 pc.printf("The current time is %s.\r\n",date); 00066 } 00067 00068 // (probably) required for rtc use 00069 void update_rtc() { 00070 // for use as interrupt routine, to insure that RTC is updated periodically 00071 // ...if rtc is not read before the underlying counter rolls over (typically 512 seconds), the RTC value will be wrong 00072 // ...ideally this would be done as part of the nrf51_rtc method, but I couldn't get it to behave (see nrf51_rtc.cpp for details) 00073 rtc.time(); 00074 rtc_update_led = !rtc_update_led; 00075 } 00076 00077 00078 int main(void) 00079 { 00080 rtc_update_led=0; 00081 button_push_led=0; 00082 00083 // user selectable, any time < 512 seconds is OK 00084 #define PERIODIC_UPDATE 1 00085 Ticker rtc_ticker; 00086 rtc_ticker.attach(&update_rtc, PERIODIC_UPDATE); // update the time regularly 00087 00088 time_t initial_time = example_time(); 00089 rtc.set_time(initial_time); 00090 00091 button1Press.fall(&print_time); // when button1 is pressed, this calls rtc.time() and prints it 00092 00093 while (true) { 00094 sleep(); 00095 } 00096 } 00097
Generated on Wed Jul 13 2022 16:29:50 by
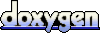