
Example application using the RM3100 magnetometer
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "Rm3100.hpp" 00003 00004 int main(void) 00005 { 00006 Serial pc(USBTX, USBRX); 00007 pc.baud(115200); 00008 00009 printf("### Hello RM3100 ###\r\n"); 00010 00011 int addr = ((Rm3100::RM3100_ADDR | Rm3100::RM3100_ADDR_SSN) << 1); 00012 struct Rm3100::Status status = { 0 }; 00013 struct Rm3100::Sample sample = { 0 }; 00014 00015 Rm3100 sensor(I2C_SDA, I2C_SCL, addr); 00016 00017 sensor.Begin(); 00018 osDelay(1); 00019 00020 sensor.SetCycleCounts(200); 00021 osDelay(1); 00022 00023 sensor.SetRate(100.0f); 00024 osDelay(1); 00025 00026 sensor.SetContinuousMeasurementMode(true); 00027 osDelay(1); 00028 00029 while (true) { 00030 sensor.GetStatus(&status); 00031 if (status.drdy) { 00032 sensor.GetSample(&sample); 00033 printf("x: %f, y: %f, z: %f\r\n", sample.x, sample.y, sample.z); 00034 } 00035 osDelay(10); 00036 } 00037 }
Generated on Wed Jul 13 2022 17:38:45 by
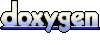