
Sample code for using the Xively peripheral board.
Dependencies: MMA7660 PinDetect ST7565R TriLED mbed-rtos mbed
main.cpp
00001 /** 00002 * My custom Xively code. This program will use most of the peripherals 00003 * on the board. If you need anything else let me know and I can probably 00004 * make some task for it to do. 00005 * 00006 * Eric Fossum 00007 */ 00008 #include "mbed.h" 00009 #include "MMA7660.h" 00010 #include "PinDetect.h" 00011 #include "rtos.h" 00012 #include "ST7565R.h" 00013 #include "tricolor.h" 00014 00015 /// Updates the potentiometers and prints their values to the LCD 00016 void pot_update(void const *args); 00017 /// Updates the devices on the I2C bus and prints their values to the LCD 00018 void i2c_update(void const *args); 00019 /// Function call for when down on the joystick is pressed 00020 void down(); 00021 /// Function call for when left on the joystick is pressed 00022 void left(); 00023 /// Function call for when the joystick is clicked 00024 void click(); 00025 /// Function call for when up on the joystick is pressed 00026 void up(); 00027 /// Function call for when right on the joystick is pressed 00028 void right(); 00029 00030 Serial pc(USBTX, USBRX); 00031 ST7565R lcd(p11, p8, p7, p5, p6, false); 00032 Tricolor led(p23, p24, p25); 00033 I2C i2c(p28, p27); 00034 MMA7660 accl(p28, p27); 00035 00036 const int temp_addr = 0x90; 00037 00038 Mutex lcd_mutex; 00039 00040 AnalogIn pot1(p19); 00041 AnalogIn pot2(p20); 00042 00043 DigitalOut led1(LED1); 00044 DigitalOut led2(LED2); 00045 DigitalOut led3(LED3); 00046 DigitalOut led4(LED4); 00047 00048 PinDetect joy_down(p12); 00049 PinDetect joy_left(p13); 00050 PinDetect joy_click(p14); 00051 PinDetect joy_up(p15); 00052 PinDetect joy_right(p16); 00053 00054 /** Main 00055 * Sets up the hardware and threads, then toggles the LED every half a second. 00056 */ 00057 int main() { 00058 joy_left.mode(PullDown); 00059 joy_right.mode(PullDown); 00060 joy_click.mode(PullDown); 00061 joy_up.mode(PullDown); 00062 joy_down.mode(PullDown); 00063 00064 joy_left.attach_asserted(&left); 00065 joy_right.attach_asserted(&right); 00066 joy_click.attach_asserted(&click); 00067 joy_up.attach_asserted(&up); 00068 joy_down.attach_asserted(&down); 00069 00070 wait(0.2); 00071 00072 joy_left.setSampleFrequency(); 00073 joy_right.setSampleFrequency(); 00074 joy_click.setSampleFrequency(); 00075 joy_up.setSampleFrequency(); 00076 joy_down.setSampleFrequency(); 00077 00078 led.SetLEDColor(0, 60, 0); 00079 00080 pc.printf("Debug out\n"); 00081 00082 lcd.moveto(0,0); 00083 lcd.printf("Custom Xively!"); 00084 // Create Tasks - task, argument, priority, stack, stk_ptr 00085 Thread pot_thread(pot_update); 00086 Thread i2c_thread(i2c_update); 00087 00088 while(1) { 00089 wait(0.5); 00090 led.Toggle(); 00091 } 00092 } 00093 00094 void pot_update(void const *args) { 00095 char str[lcd.columns()+1]; 00096 00097 while(true) { 00098 lcd_mutex.lock(); 00099 lcd.moveto(0,1); 00100 sprintf(str, "Pot1: %00.2f Pot2: %00.2f", pot1.read(), pot2.read()); 00101 lcd.printf(str); 00102 lcd_mutex.unlock(); 00103 } 00104 } 00105 00106 void i2c_update(void const *args) { 00107 char str[lcd.columns()+1]; 00108 char cmd[2]; 00109 float temp; 00110 00111 while(true) { 00112 // Temp Sensor 00113 cmd[0] = 0x01; 00114 cmd[1] = 0x00; 00115 i2c.write(temp_addr, cmd, 2); 00116 wait(0.5); 00117 cmd[0] = 0x00; 00118 i2c.write(temp_addr, cmd, 1); 00119 i2c.read(temp_addr, cmd, 2); 00120 temp = ((float((cmd[0]<<8)|cmd[1]) / 256.0)*1.8) + 32; 00121 00122 lcd_mutex.lock(); 00123 lcd.moveto(0,2); 00124 sprintf(str, "Temp: %2.1f, Z: % 2.1f", temp, lcd.rows()); 00125 lcd.printf(str); 00126 00127 // Accelerometer 00128 lcd.moveto(0,3); 00129 sprintf(str, "X: % 2.1f Y: % 2.1f", accl.x(), accl.y()); 00130 lcd.printf(str); 00131 lcd_mutex.unlock(); 00132 } 00133 } 00134 00135 void down() { 00136 led3 = !led3; 00137 } 00138 00139 void left() { 00140 led1 = !led1; 00141 } 00142 00143 void click() { 00144 led1 = !led1; 00145 led2 = !led2; 00146 led3 = !led3; 00147 led4 = !led4; 00148 } 00149 00150 void up() { 00151 led2 = !led2; 00152 } 00153 00154 void right() { 00155 led4 = !led4; 00156 }
Generated on Tue Jul 12 2022 19:17:03 by
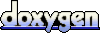