SparkFun SerLCD v2.5 controller; Copied from the Arduino.cc SerLCD library; http://playground.arduino.cc/Code/SerLCD
Dependents: ForEhab Serial_HelloWorld_Mbed ForOfek
Fork of LCDSerial by
SerialLCD.h
00001 #ifndef SerialLCD_h 00002 #define SerialLCD_h 00003 00004 #include "mbed.h" 00005 00006 #define LCD_BAUD 9600 00007 00008 // Commands 00009 #define LCD_BACKLIGHT 0x80 00010 #define LCD_CLEARDISPLAY 0x01 00011 #define LCD_CURSORSHIFT 0x10 00012 #define LCD_DISPLAYCONTROL 0x08 00013 #define LCD_ENTRYMODESET 0x04 00014 #define LCD_FUNCTIONSET 0x20 00015 #define LCD_SETCGRAMADDR 0x40 00016 #define LCD_SETDDRAMADDR 0x80 00017 #define LCD_SETSPLASHSCREEN 0x0A 00018 #define LCD_SPLASHTOGGLE 0x09 00019 #define LCD_RETURNHOME 0x02 00020 00021 // Flags for display entry mode 00022 #define LCD_ENTRYRIGHT 0x00 00023 #define LCD_ENTRYLEFT 0x02 00024 00025 // Flags for display on/off control 00026 #define LCD_BLINKON 0x01 00027 #define LCD_CURSORON 0x02 00028 #define LCD_DISPLAYON 0x04 00029 00030 // Flags for display size 00031 #define LCD_2LINE 0x02 00032 #define LCD_4LINE 0x04 00033 #define LCD_16CHAR 0x10 00034 #define LCD_20CHAR 0x14 00035 00036 // Flags for setting display size 00037 #define LCD_SET2LINE 0x06 00038 #define LCD_SET4LINE 0x05 00039 #define LCD_SET16CHAR 0x04 00040 #define LCD_SET20CHAR 0x03 00041 00042 // LCD Types 00043 #define LCD_2X16 3 00044 #define LCD_2X20 4 00045 #define LCD_4X16 5 00046 #define LCD_4X20 6 00047 00048 /** 00049 * SparkFun SerLCD v2.5 Controller 00050 * 00051 * Copied from the Arduino.cc SerLCD library. 00052 * @see http://playground.arduino.cc/Code/SerLCD 00053 */ 00054 class SerialLCD : public Serial 00055 { 00056 public: 00057 /** Constructor. 00058 * @param tx Connected to rx pin of LCD 00059 * @param type Type of LCD (Optional default 16x2) 00060 */ 00061 SerialLCD(PinName tx, uint8_t type = 3); 00062 00063 /** Blinks the cursor. This blinks the whole 00064 * box or just the cursor bar. 00065 */ 00066 void blink(); 00067 00068 /** Clears the whole LCD and sets the 00069 * cursor to the first row, first column. 00070 */ 00071 void clear(); 00072 /** Clears the current line and resets 00073 * the cursor to the beginning of the 00074 * line. 00075 */ 00076 void clearLine(uint8_t); 00077 /** Creates a custom character for the 00078 * LCD. 00079 * @param location Location to store the char (1-8) 00080 * @param data Byte array containing the enabled pixel bits 00081 * @code 00082 * #include <NewSoftSerial.h> 00083 * #include <serLCD.h> 00084 * 00085 * // Set pin to the LCD's rxPin 00086 * int pin = 2; 00087 * 00088 * serLCD lcd(pin); 00089 * 00090 * byte bars[8][8] = { 00091 * {B00000,B00000,B00000,B00000,B00000,B00000,B00000,B11111}, 00092 * {B00000,B00000,B00000,B00000,B00000,B00000,B11111,B11111}, 00093 * {B00000,B00000,B00000,B00000,B00000,B11111,B11111,B11111}, 00094 * {B00000,B00000,B00000,B00000,B11111,B11111,B11111,B11111}, 00095 * {B00000,B00000,B00000,B11111,B11111,B11111,B11111,B11111}, 00096 * {B00000,B00000,B11111,B11111,B11111,B11111,B11111,B11111}, 00097 * {B00000,B11111,B11111,B11111,B11111,B11111,B11111,B11111}, 00098 * {B11111,B11111,B11111,B11111,B11111,B11111,B11111,B11111} 00099 * }; 00100 * 00101 * void main() { 00102 * for (int i=1; i < 9; i++){ 00103 * lcd.createChar(i, bars[i-1]); 00104 * } 00105 * for (int i=1; i < 9; i++){ 00106 * lcd.printCustomChar(i); 00107 * } 00108 * while(1); 00109 * } 00110 * 00111 * @endcode 00112 */ 00113 void createChar(uint8_t, uint8_t[]); 00114 /** Display the LCD cursor: an underscore (line) at the 00115 * position to which the next character will be written. 00116 * This does not define if it blinks or not. 00117 */ 00118 void cursor(); 00119 /** Turns on the LCD display, after it's been turned off 00120 * with noDisplay(). This will restore the text (and 00121 * cursor) that was on the display. 00122 */ 00123 void display(); 00124 /** Positions the cursor in the upper-left of the LCD. 00125 */ 00126 void home(); 00127 /** Set the direction for text written to the LCD to 00128 * left-to-right, the default. This means that subsequent 00129 * characters written to the display will go from left to 00130 * right, but does not affect previously-output text. 00131 */ 00132 void leftToRight(); 00133 /** Turns off the blinking LCD cursor. 00134 */ 00135 void noBlink(); 00136 /** Hides the LCD cursor. 00137 */ 00138 void noCursor(); 00139 /** Turns off the LCD display, without losing the text 00140 * currently shown on it. 00141 */ 00142 void noDisplay(); 00143 /** Print a custom character (gylph) on to the LCD. Up 00144 * to eight characters of 5x8 pixels are supported 00145 * (numbered 1 to 8). The custom characters are designed 00146 * by the createChar() command. 00147 * @param location Which character to print (1 to 8), created by createChar() 00148 */ 00149 void printCustomChar(uint8_t); 00150 /** Set the direction for text written to the LCD to 00151 * right-to-left (the default is left-to-right). This 00152 * means that subsequent characters written to the 00153 * display will go from right to left, but does not 00154 * affect previously-output text. 00155 */ 00156 void rightToLeft(); 00157 /** Scrolls the text on the LCD one position to the left. 00158 */ 00159 void scrollLeft(); 00160 /** Scrolls the text on the LCD one position to the right. 00161 */ 00162 void scrollRight(); 00163 /** Moves the cursor to the beginning of selected line. 00164 * Line numbers start with 1. Valid line numbers are 1-4. 00165 * @param line Line number to go to 00166 */ 00167 void selectLine(uint8_t line); 00168 /** Sets the backlight brightness based on input value. 00169 * Values range from 1-30. 00170 * @params brightness 1 = Off -> 30 = Full Brightness 00171 */ 00172 void setBrightness(uint8_t num); 00173 /** Position the LCD cursor. 00174 * @param row Row position of the cursor (1 being the first row) 00175 * @param col Column position the cursor (1 being the first column) 00176 */ 00177 void setCursor(uint8_t row, uint8_t col); 00178 /** Saves the first 2 lines of text that are currently 00179 * printed on the LCD screen to memory as the new splash screen. 00180 * @code 00181 * void setup() 00182 * { 00183 * lcd.print(" New "); 00184 * lcd.print(" Splash Screen! "); 00185 * lcd.setSplash(); 00186 * } 00187 * @endcode 00188 */ 00189 void setSplash(); 00190 /** The SerLCD firmware v2.5 supports setting different types 00191 * of LCD's. This function allows to easily set the type of LCD. 00192 * 00193 * The function expects a parameter value of 3 - 6: 00194 * Defines: 00195 * LCD_2X16(3) - 2x16 LCD 00196 * LCD_2X20(4) - 2x20 LCD 00197 * LCD_4X16(5) - 4x16 LCD 00198 * LCD_4X20(6) - 4x20 LCD 00199 * 00200 * After changing the settings, the value is written to the EEPROM 00201 * so the SerialLCD will remember it even after a power-off. 00202 */ 00203 void setType(uint8_t type); 00204 /** Toggles the splash screen on/off. 00205 */ 00206 void toggleSplash(); 00207 00208 private: 00209 void command(uint8_t); 00210 void specialCommand(uint8_t); 00211 00212 uint8_t _displayfunction; 00213 uint8_t _displaycontrol; 00214 uint8_t _displaymode; 00215 uint8_t _numlines; 00216 uint8_t _numchars; 00217 uint8_t _rowoffset; 00218 }; 00219 00220 #endif
Generated on Tue Jul 12 2022 19:12:00 by
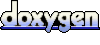