
A minimal library for the DHT11.
Dependents: EXP10_DHT11_LCD Sushil_MODSERIAL Core1000_SmartFarm idd_summer17_hw3_evey_jenny_seiyoung ... more
Dht11.cpp
00001 #include "Dht11.h" 00002 00003 Dht11::Dht11(PinName const &p) : _pin(p) { 00004 // Set creation time so we can make 00005 // sure we pause at least 1 second for 00006 // startup. 00007 _timer.start(); 00008 00009 _temperature = 0; 00010 _humidity = 0; 00011 } 00012 00013 int Dht11::read() 00014 { 00015 // BUFFER TO RECEIVE 00016 uint8_t bits[5]; 00017 uint8_t cnt = 7; 00018 uint8_t idx = 0; 00019 00020 // EMPTY BUFFER 00021 for (int i=0; i< 5; i++) bits[i] = 0; 00022 00023 // Verify sensor settled after boot 00024 while(_timer.read_ms() < 1500) {} 00025 _timer.stop(); 00026 00027 // Notify it we are ready to read 00028 _pin.output(); 00029 _pin = 0; 00030 wait_ms(18); 00031 _pin = 1; 00032 wait_us(40); 00033 _pin.input(); 00034 00035 // ACKNOWLEDGE or TIMEOUT 00036 unsigned int loopCnt = 10000; 00037 while(_pin == 0) 00038 if (loopCnt-- == 0) return DHTLIB_ERROR_TIMEOUT; 00039 00040 loopCnt = 10000; 00041 while(_pin == 1) 00042 if (loopCnt-- == 0) return DHTLIB_ERROR_TIMEOUT; 00043 00044 // READ OUTPUT - 40 BITS => 5 BYTES or TIMEOUT 00045 for (int i=0; i<40; i++) 00046 { 00047 loopCnt = 10000; 00048 while(_pin == 0) 00049 if (loopCnt-- == 0) return DHTLIB_ERROR_TIMEOUT; 00050 00051 //unsigned long t = micros(); 00052 Timer t; 00053 t. start(); 00054 00055 loopCnt = 10000; 00056 while(_pin == 1) 00057 if (loopCnt-- == 0) return DHTLIB_ERROR_TIMEOUT; 00058 00059 if (t.read_us() > 40) bits[idx] |= (1 << cnt); 00060 if (cnt == 0) // next byte? 00061 { 00062 cnt = 7; // restart at MSB 00063 idx++; // next byte! 00064 } 00065 else cnt--; 00066 } 00067 00068 // WRITE TO RIGHT VARS 00069 // as bits[1] and bits[3] are allways zero they are omitted in formulas. 00070 _humidity = bits[0]; 00071 _temperature = bits[2]; 00072 00073 uint8_t sum = bits[0] + bits[2]; 00074 00075 if (bits[4] != sum) return DHTLIB_ERROR_CHECKSUM; 00076 return DHTLIB_OK; 00077 } 00078 00079 float Dht11::getFahrenheit() { 00080 return((_temperature * 1.8) + 32); 00081 } 00082 00083 int Dht11::getCelsius() { 00084 return(_temperature); 00085 } 00086 00087 int Dht11::getHumidity() { 00088 return(_humidity); 00089 }
Generated on Wed Jul 13 2022 01:42:32 by
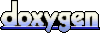