
Konacan kod
Dependencies: BSP_DISCO_F469NIa LCD_DISCO_F469NIa SD_DISCO_F469NI mbed
draw_print_library.h
00001 #include "LCD_DISCO_F469NI.h" 00002 #include "SD_DISCO_F469NI.h" 00003 00004 #define PI 3.14159265358979323846 00005 #define BYTE_TO_BINARY_PATTERN "%c%c%c%c%c%c%c%c" //Pattern which converts uint8_t to binary(array of 8 chars) 00006 #define BYTE_TO_BINARY(byte)\ 00007 (byte & 0x80 ? '1' : '0'),\ 00008 (byte & 0x40 ? '1' : '0'),\ 00009 (byte & 0x20 ? '1' : '0'),\ 00010 (byte & 0x10 ? '1' : '0'),\ 00011 (byte & 0x08 ? '1' : '0'),\ 00012 (byte & 0x04 ? '1' : '0'),\ 00013 (byte & 0x02 ? '1' : '0'),\ 00014 (byte & 0x01 ? '1' : '0') 00015 00016 #define LOGOBIG_START_ADDR 0 //Address for Big Logo in SD Card used in Intro 00017 #define LOGOSMALL_START_ADDR 1536000 //Address for Small Logo in SD Card used as header 00018 #define BRANKO_START_ADDR 1576960 //Branko start address 00019 #define NEW_YEAR_CONGAT_START_ADDR 2056960 //New Year start address 00020 00021 const double PHI=53.13010235*PI/180; //Angles used in drawing Speedmeter. 00022 const double ALPHA=73.73979529*PI/180; // 00023 const double Vmax=150; 00024 00025 //Positions of Informations on screen 00026 const uint16_t GearXPos=272,GearYPos=95; //Gear in Main 00027 const uint16_t OilTempXPos=15,OilTempYPos=200; //Oil Temperature in Main 00028 const uint16_t LogoSmallXPos=635,LogoSmallYPos=5; //Small Logo, all three screens 00029 const uint16_t WaterTempXPos=15,WaterTempYPos=350; //Water Temperature in Main 00030 const uint16_t TPSXPos=569,TPSYPos=200; //TPS in Main 00031 const uint16_t OilPXPos=330,OilPYPos=25; //Oil Pressure in First Auxiliary 00032 const uint16_t MAPXPos=330,MAPYPos=100; //MAP in First Auxiliary 00033 const uint16_t AirTempXPos=330,AirTempYPos=175; //Air Temperature in First Auxiliary 00034 const uint16_t LambdaXPos=330,LambdaYPos=250; //Lambda Sensor value in First Auxiliary 00035 const uint16_t VoltsXPos=330,VoltsYPos=325; //Volts in Accumulator in First Auxiliary 00036 const uint16_t CrankXPos=330,CrankYPos=400; //Crank Error Message in First Auxiliary 00037 //YPos in bottom position for LVDTs 00038 const uint16_t FLLVDTBarXPos=280,FLLVDTBarYPos=200; //Front Left Bar LVDT in Second Auxiliary 00039 const uint16_t FRLVDTBarXPos=440,FRLVDTBarYPos=200; //Front Right Bar LVDT in Second Auxiliary 00040 const uint16_t RLLVDTBarXPos=280,RLLVDTBarYPos=410; //Rear Left Bar LVDT in Second Auxiliary 00041 const uint16_t RRLVDTBarXPos=440,RRLVDTBarYPos=410; //Rear Right Bar LVDT in Second Auxiliary 00042 const uint16_t FLLVDTXPos=10,FLLVDTYPos=100; //Front Left LVDT in Second Auxiliary 00043 const uint16_t FRLVDTXPos=534,FRLVDTYPos=100; //Front Right LVDT in Second Auxiliary 00044 const uint16_t RLLVDTXPos=10,RLLVDTYPos=310; //Rear Left LVDT in Second Auxiliary 00045 const uint16_t RRLVDTXPos=534,RRLVDTYPos=310; //Rear Right LVDT in Second Auxiliary 00046 00047 00048 typedef struct BWImage{ //Black-White Image Structure. These are stored on the controller 00049 char name; 00050 uint16_t width; 00051 uint16_t height; 00052 uint8_t *bitmap; 00053 } GEAR,CHAR; 00054 00055 typedef struct RGBImage{ //RGB Coloured Image Structure. These are stored on SD Card 00056 uint16_t width; 00057 uint16_t height; 00058 uint32_t START_ADDR; 00059 }IMAGE; 00060 00061 void DrawSpeedMeter(); //Draw Speedmeter function 00062 void PrintChar(CHAR Char,uint16_t StartXPos,uint16_t StartYPos,uint32_t TextColor); //Print Char function 00063 void PrintString(char str[],int font,uint16_t StartXPos,uint16_t StartYPos,uint32_t TextColor); //Print String function 00064 void ChangeNumber(int num,int num0,int Font,uint16_t StartXPos, uint16_t StartYPos, int digits, int dec_point, int sign); //Update number function 00065 void SetNumber(int num,int Font,uint16_t StartXPos,uint16_t StartYPos, int digits, int dec_point, int sign); //Set number to specific value function 00066 void DrawRGBImage(IMAGE Image,uint16_t StartXPos,uint16_t StartYPos); //Draw RGB Coloured image function 00067 void UpdateSpeedMeter(int V,int dV); //Update Speedmeter function 00068 void ChangeCrank(int Crank); //Update Crank error message function 00069 int UpdateLVDTScale(int H,int H0, uint16_t StartXPos, uint16_t StartYPos); //Update LVDT Bar function 00070 void BrakeSignal(int brake); //Set Brake signal function 00071 void TestFont(); //Test font 50 function
Generated on Thu Jul 14 2022 09:25:33 by
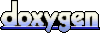