
Konacan kod
Dependencies: BSP_DISCO_F469NIa LCD_DISCO_F469NIa SD_DISCO_F469NI mbed
draw_print_library.cpp
00001 #include "draw_print_library.h" 00002 #include "gears.h" 00003 #include "font100.h" 00004 #include "font50.h" 00005 00006 extern LCD_DISCO_F469NI lcd; 00007 extern SD_DISCO_F469NI sd; 00008 extern Serial pc; 00009 00010 extern uint8_t lvdtref; 00011 extern int FL_LVDT_Ref,FR_LVDT_Ref,RL_LVDT_Ref,RR_LVDT_Ref; 00012 00013 //Gears 00014 GEAR Idle{'0',256,384,idleBitmap}; 00015 GEAR Gear1{'1',256,384,gear1Bitmap}; 00016 GEAR Gear2{'2',256,384,gear2Bitmap}; 00017 GEAR Gear3{'3',256,384,gear3Bitmap}; 00018 GEAR Gear4{'4',256,384,gear4Bitmap}; 00019 GEAR Gear5{'5',256,384,gear5Bitmap}; 00020 GEAR Gear6{'6',256,384,gear6Bitmap}; 00021 GEAR *Gears[7]={&Idle,&Gear1,&Gear2,&Gear3,&Gear4,&Gear5,&Gear6}; //Gears array 00022 IMAGE LogoBig{800,480,LOGOBIG_START_ADDR}; //Big Logo 00023 IMAGE LogoSmall{160,64,LOGOSMALL_START_ADDR}; //Small Logo 00024 IMAGE Branko{250,480,1576960}; //Branko 00025 IMAGE NewYearCongat{800,480,NEW_YEAR_CONGAT_START_ADDR}; 00026 //IMAGE Miljana[250,480,---}; 00027 00028 void DrawSpeedMeter(){ 00029 uint16_t x1,y1,x2,y2,x3,y3,x4,y4; 00030 double THETA1=PHI,THETA2; 00031 00032 //Set starting point for drawing 00033 x1=(int)(400-530*cos(THETA1)); 00034 y1=(int)(550-530*sin(THETA1)); 00035 x2=(int)(400-500*cos(THETA1)); 00036 y2=(int)(550-500*sin(THETA1)); 00037 00038 lcd.SetTextColor(LCD_COLOR_BLACK); 00039 for (int V=0;V<=150;V++){ //Draw from 0 to 150 00040 //Set angle for next speed scale value 00041 THETA2=THETA1+ALPHA/Vmax; 00042 x3=(int)(400-530*cos(THETA2)); 00043 y3=(int)(550-530*sin(THETA2)); 00044 x4=(int)(400-500*cos(THETA2)); 00045 y4=(int)(550-500*sin(THETA2)); 00046 if(V<150){ //Draw arch section 00047 lcd.DrawLine(x1,y1,x3,y3); 00048 lcd.DrawLine(x2,y2,x4,y4); 00049 }; 00050 lcd.SetFont(&Font20); 00051 if(V%10==0){ //Print round values (0,10,20,...) 00052 lcd.DrawLine(x1,y1,x2,y2); //Draw round scale value 00053 char Vchar[3]; 00054 sprintf(Vchar,"%d",(int)V); 00055 uint16_t xnum=x2,ynum=y2; 00056 if (V==0 | V==10 | V==20 | V==30){ //Position of printed value 00057 xnum=x2,ynum=y2+5; 00058 }else if (V==40 | V==50 | V==60){ 00059 xnum=x2-3,ynum=y2+5; 00060 }else if (V==70 | V==80){ 00061 xnum=x2-14,ynum=y2+5; 00062 }else if (V==90){ 00063 xnum=x2-20,ynum=y2+5; 00064 }else if (V==100 | V==110 | V==120) { 00065 xnum=x2-35,ynum=y2+4; 00066 }else if (V==130 | V==140 | V==150){ 00067 xnum=x2-35,ynum=y2+5; 00068 }; 00069 lcd.DisplayStringAt(xnum,ynum,(uint8_t*)Vchar,LEFT_MODE); 00070 }; 00071 x1=x3,y1=y3,x2=x4,y2=y4; //Increment position 00072 THETA1=THETA2; 00073 }; 00074 }; 00075 00076 void PrintChar(CHAR Char,uint16_t StartXPos,uint16_t StartYPos,uint32_t TextColor){ 00077 uint16_t width=Char.width; 00078 uint16_t height=Char.height; 00079 uint16_t horpos,vertpos; 00080 uint16_t bitloc; 00081 uint32_t DrawColor; 00082 char pos[9]; 00083 //uint8_t dbg[50]; 00084 for(horpos=0;horpos<width;horpos++){ //Bitmaps are row after row 00085 for(vertpos=0;vertpos<height/8;vertpos++){ 00086 sprintf(pos,BYTE_TO_BINARY_PATTERN,BYTE_TO_BINARY(Char.bitmap[horpos*height/8+vertpos])); //Convert uint8_t from hex to binary. 1 to fill, 0 to skip 00087 //pc.printf("%d. %s\n",horpos*height/8+vertpos,pos); 00088 for(bitloc=0;bitloc<8;bitloc++) { 00089 if (pos[bitloc]=='1') { 00090 DrawColor=TextColor; 00091 } 00092 else{ 00093 DrawColor=lcd.GetBackColor(); 00094 }; 00095 lcd.DrawPixel(StartXPos+horpos,StartYPos+vertpos*8+bitloc,DrawColor); 00096 //pc.printf("%d,%d\n",StartXPos+horpos,StartYPos+vertpos*8+bitloc); 00097 } 00098 } 00099 } 00100 lcd.SetTextColor(LCD_COLOR_BLACK); 00101 }; 00102 00103 void PrintString(char str[],int font,uint16_t StartXPos,uint16_t StartYPos,uint32_t TextColor){ 00104 //pc.printf("Function activated.\n"); 00105 char *a=str; 00106 int p=0; 00107 while(a[p]){ 00108 //pc.printf("Searching char: %c\n",a[p]); 00109 for(int k=0;k<68;k++){ 00110 //pc.printf("Character %c\n",(*font50[k]).name); 00111 if((*font50[k]).name==a[p]){ 00112 // pc.printf("Found!\n"); 00113 PrintChar(*font50[k],StartXPos,StartYPos,TextColor); 00114 StartXPos+=(*font50[k]).width; 00115 k=100; 00116 }; 00117 }; 00118 //pc.printf("End.\n"); 00119 p++; 00120 }; 00121 }; 00122 00123 00124 00125 00126 void ChangeNumber(int num,int num0,int Font,uint16_t StartXPos,uint16_t StartYPos, int digits, int dec_point, int sign){ 00127 //Function only changes the decimals that change. If number changes from 146 to 147, it only changes 6 to 7. 00128 int digit[digits],digit0[digits]; 00129 int sum=0,sum0=0,k,aux=0; 00130 CHAR **font; 00131 if(abs(num)<pow(float(10),digits)){ 00132 digit[0]=abs(num)/pow((float)10,(float)(digits-1)); 00133 digit0[0]=abs(num0)/pow((float)10,(float)(digits-1)); 00134 for(k=1;k<digits;k++){ 00135 sum=(sum+digit[k-1])*10; 00136 digit[k]=abs(num)/pow((float)10,(float)(digits-1-k))-sum; 00137 sum0=(sum0+digit0[k-1])*10; 00138 digit0[k]=abs(num0)/pow(10,(float)(digits-1-k))-sum0; 00139 }; 00140 00141 if (Font==50){ 00142 font=font50; 00143 }else if (Font==100){ 00144 font=font100; 00145 }; 00146 00147 lcd.SetTextColor(LCD_COLOR_BLACK); 00148 uint8_t char_width=(*font[0]).width; 00149 00150 if (sign==1){ 00151 if(num<0 & num0>=0){ //Print minus if number lower than 0 00152 PrintChar(*font[10],StartXPos,StartYPos,lcd.GetTextColor()); 00153 }else if(num>=0 & num0<0){ //Print blank if number lower than 0 00154 lcd.SetTextColor(LCD_COLOR_WHITE); 00155 lcd.FillRect(StartXPos,StartYPos,(*font[10]).width,(*font[10]).height); 00156 lcd.SetTextColor(LCD_COLOR_BLACK); 00157 }; 00158 aux+=(*font[10]).width; 00159 }; 00160 00161 for(k=0;k<digits;k++){ 00162 if(dec_point==k & dec_point>0){ //Default is for font50. This is not an universal solution, but it is in use because all font 100 infos are integers 00163 PrintChar(Chardot_50,StartXPos+dec_point*char_width,StartYPos,lcd.GetTextColor()); 00164 aux+=Chardot_50.width; 00165 }; 00166 if(digit[k]!=digit0[k]){ 00167 PrintChar(*font[digit[k]],StartXPos+k*char_width+aux,StartYPos,lcd.GetTextColor()); 00168 }; 00169 }; 00170 }; 00171 }; 00172 00173 void SetNumber(int num,int Font,uint16_t StartXPos,uint16_t StartYPos, int digits, int dec_point, int sign){ 00174 int digit[3],sum=0,k,aux=0; 00175 CHAR **font; 00176 00177 if (Font==50){ 00178 font=font50; 00179 }else if (Font==100){ 00180 font=font100; 00181 }; 00182 00183 lcd.SetTextColor(LCD_COLOR_BLACK); 00184 uint8_t char_width=(*font[0]).width; 00185 00186 if(num<pow(float(10),digits)){ 00187 digit[0]=abs(num)/pow((float)10,(float)(digits-1)); 00188 for(k=1;k<digits;k++){ 00189 sum=(sum+digit[k-1])*10; 00190 digit[k]=abs(num)/pow((float)10,(float)(digits-1-k))-sum; 00191 }; 00192 00193 if(sign==1){ 00194 if(num<0){ //Print minus if number lower than 0 00195 PrintChar(*font[10],StartXPos,StartYPos,lcd.GetTextColor()); 00196 }else if(num>=0){ //Print blank if number lower than 0 00197 lcd.SetTextColor(LCD_COLOR_WHITE); 00198 lcd.FillRect(StartXPos,StartYPos,(*font[10]).width,(*font[10]).height); 00199 lcd.SetTextColor(LCD_COLOR_BLACK); 00200 }; 00201 aux+=(*font[10]).width; 00202 }; 00203 00204 for(k=0;k<digits;k++){ 00205 if(dec_point==k & dec_point>0){ //Default is for font50. This is not an universal solution, but it is in use because all font 100 infos are integers 00206 PrintChar(Chardot_50,StartXPos+dec_point*char_width,StartYPos,lcd.GetTextColor()); 00207 aux+=Chardot_50.width; 00208 }; 00209 PrintChar(*font[digit[k]],StartXPos+k*char_width+aux,StartYPos,lcd.GetTextColor()); 00210 }; 00211 }; 00212 }; 00213 00214 void DrawRGBImage(IMAGE Image,uint16_t StartXPos,uint16_t StartYPos){ 00215 uint32_t p,q; 00216 uint32_t BlockBuffer[128]; 00217 uint16_t xpos=0,ypos=0; 00218 for(p=0;p<Image.width*Image.height/128;p++){ 00219 sd.ReadBlocks(BlockBuffer,Image.START_ADDR+p*512,1,SD_DATATIMEOUT); 00220 for(q=0;q<128;q++){ 00221 if(ypos<Image.height){ 00222 lcd.DrawPixel(StartXPos+xpos,StartYPos+ypos,BlockBuffer[q]); 00223 } 00224 else{ 00225 xpos++; 00226 ypos=0; 00227 lcd.DrawPixel(StartXPos+xpos,StartYPos+ypos,BlockBuffer[q]); 00228 } 00229 ypos++; 00230 }; 00231 }; 00232 }; 00233 00234 00235 void UpdateSpeedMeter(int V, int dV){ 00236 //Prednost koda je sto se docrtava samo onaj deo koji se menja. Tako ako sa 55 kmh prelazimo na 57 khm on obradjuje samo polja 55 i 56. 00237 //Kada bi se samo brisala stara i crtala nova vrednost na baru ukupno bi morali da obradimo 55+57=112 umesto samo 2 bara. 00238 uint32_t OldColor,RewriteColor; 00239 double THETA1,THETA2; 00240 uint16_t x1,y1,x2,y2,x3,y3,x4,y4; 00241 uint16_t startx,starty,leftupx,rightupx,leftdownx,rightdownx,ypos; 00242 00243 //Obradjivanje polje po polje. Svako polje prestavlja odredjenu vrednost kmh. 00244 for(int k=1;k<=abs(dV);k++){ 00245 //Uokviravanje polja koje se trenutno obradjuje. Granice su crne kako bi se jasno razgranicilo polje od ostatka bara. 00246 lcd.SetTextColor(LCD_COLOR_BLACK); 00247 THETA1=PHI+((double)V)*ALPHA/Vmax; 00248 x1=(int)(400-530*cos(THETA1)); 00249 y1=(int)(550-530*sin(THETA1)); 00250 x2=(int)(400-500*cos(THETA1)); 00251 y2=(int)(550-500*sin(THETA1)); 00252 THETA2=PHI+((double)(V+dV/abs(dV)))*ALPHA/Vmax; 00253 x3=(int)(400-530*cos(THETA2)); 00254 y3=(int)(550-530*sin(THETA2)); 00255 x4=(int)(400-500*cos(THETA2)); 00256 y4=(int)(550-500*sin(THETA2)); 00257 lcd.DrawLine(x1,y1,x2,y2); 00258 lcd.DrawLine(x3,y3,x4,y4); 00259 lcd.DrawLine(x1,y1,x3,y3); 00260 lcd.DrawLine(x2,y2,x4,y4); 00261 00262 //Odredjivanje pocetnih koordinata i kojom bojom ce se polje bojiti. 00263 if(dV>0){ 00264 startx=(x2+x3)/2; 00265 starty=(y2+y3)/2; 00266 if(V+1<=50){ 00267 RewriteColor=LCD_COLOR_GREEN; 00268 }else if (V+1>50 & V+1<=100){ 00269 RewriteColor=LCD_COLOR_BLUE; 00270 }else{ 00271 RewriteColor=LCD_COLOR_RED; 00272 }; 00273 OldColor=LCD_COLOR_WHITE; 00274 }else{ 00275 startx=(x1+x4)/2; 00276 starty=(y1+y4)/2; 00277 if(V<=50){ 00278 OldColor=LCD_COLOR_GREEN; 00279 }else if (V>50 & V<=100){ 00280 OldColor=LCD_COLOR_BLUE; 00281 }else{ 00282 OldColor=LCD_COLOR_RED; 00283 }; 00284 RewriteColor=LCD_COLOR_WHITE; 00285 }; 00286 lcd.SetTextColor(RewriteColor); 00287 00288 //Odredjivanje pocetnog piksela odakle ce bojenje poceti. Kako bi se svaki piksel obojio pocetni se postavlja u centru polja i krece se najpre 00289 //na gore pa zatim od sredine na dole. 00290 leftupx=startx; 00291 rightupx=startx+1; 00292 ypos=starty; 00293 while(leftupx<rightupx){ 00294 00295 ypos--; 00296 if(lcd.ReadPixel(leftupx,ypos)==LCD_COLOR_BLACK){ 00297 while(lcd.ReadPixel(leftupx,ypos)==LCD_COLOR_BLACK){ 00298 leftupx++; 00299 } 00300 } else { 00301 while(lcd.ReadPixel(leftupx,ypos)==OldColor){ 00302 leftupx--; 00303 }; 00304 leftupx++; 00305 }; 00306 if(lcd.ReadPixel(rightupx,ypos)==LCD_COLOR_BLACK){ 00307 while(lcd.ReadPixel(rightupx,ypos)==LCD_COLOR_BLACK){ 00308 rightupx--; 00309 } 00310 } else { 00311 while(lcd.ReadPixel(rightupx,ypos)==OldColor){ 00312 rightupx++; 00313 }; 00314 rightupx--; 00315 }; 00316 00317 if (leftupx<=rightupx){ 00318 lcd.DrawLine(leftupx,ypos,rightupx,ypos); 00319 }; 00320 }; 00321 00322 leftdownx=startx; 00323 rightdownx=startx+1; 00324 ypos=starty-1; 00325 while(leftdownx<rightdownx){ 00326 ypos++; 00327 if(lcd.ReadPixel(leftdownx,ypos)==LCD_COLOR_BLACK){ 00328 while(lcd.ReadPixel(leftdownx,ypos)==LCD_COLOR_BLACK){ 00329 leftdownx++; 00330 } 00331 } else { 00332 while(lcd.ReadPixel(leftdownx,ypos)==OldColor){ 00333 leftdownx--; 00334 }; 00335 leftdownx++; 00336 }; 00337 if(lcd.ReadPixel(rightdownx,ypos)==LCD_COLOR_BLACK){ 00338 while(lcd.ReadPixel(rightdownx,ypos)==LCD_COLOR_BLACK){ 00339 rightdownx--; 00340 } 00341 } else { 00342 while(lcd.ReadPixel(rightdownx,ypos)==OldColor){ 00343 rightdownx++; 00344 }; 00345 rightdownx--; 00346 }; 00347 00348 if (leftdownx<=rightdownx){ 00349 lcd.DrawLine(leftdownx,ypos,rightdownx,ypos); 00350 }; 00351 }; 00352 00353 //Brisanje granicnika. Vodi se racuna ako je granica neki od dekadnih podeoka koji treba da ostane crn. 00354 if(V%10!=0){ 00355 lcd.DrawLine(x1,y1,x2,y2); 00356 if (dV<0){ 00357 lcd.DrawPixel(x1,y1,LCD_COLOR_BLACK); 00358 lcd.DrawPixel(x2,y2,LCD_COLOR_BLACK); 00359 }; 00360 }; 00361 V+=dV/abs(dV); 00362 }; 00363 }; 00364 00365 void ChangeCrank(int Crank){ 00366 PrintString(" ",50,350,400,LCD_COLOR_GREEN); 00367 if (Crank){ 00368 PrintString("ERROR",50,CrankXPos,CrankYPos,LCD_COLOR_RED); 00369 lcd.SetTextColor(LCD_COLOR_BLACK); 00370 } else { 00371 PrintString("OK",50,CrankXPos,CrankYPos,LCD_COLOR_GREEN); 00372 lcd.SetTextColor(LCD_COLOR_BLACK); 00373 }; 00374 }; 00375 00376 int UpdateLVDTScale(int H,int H0, uint16_t StartXPos, uint16_t StartYPos){ 00377 uint16_t BarWidth=80,BarHeight=8; 00378 int YPos; 00379 int D,D0,dD; 00380 00381 switch( ((H>=0)<<1)+(H0>0)){ 00382 case(0): 00383 D=5-abs(H)/20; 00384 D0=5-abs(H0)/20; 00385 break; 00386 case(1): 00387 D=5-abs(H)/20; 00388 D0=H0/20+5; 00389 break; 00390 case(2): 00391 D=H/20+5; 00392 D0=5-abs(H0)/20; 00393 break; 00394 case(3): 00395 D=H/20+5; 00396 D0=H0/20+5; 00397 break; 00398 }; 00399 dD=D-D0; 00400 00401 if (dD>0){ 00402 lcd.SetTextColor(LCD_COLOR_BLACK); 00403 YPos=StartYPos-10*(D0+1); 00404 }else{ 00405 lcd.SetTextColor(LCD_COLOR_WHITE); 00406 YPos=StartYPos-D0*10; 00407 }; 00408 for (int k=1;k<=abs(dD);k++){ 00409 lcd.FillRect(StartXPos,YPos,BarWidth,BarHeight); 00410 YPos-=10*(dD/abs(dD)); 00411 }; 00412 lcd.SetTextColor(LCD_COLOR_BLACK); 00413 return 1; 00414 }; 00415 00416 00417 void BrakeSignal(int brake){ 00418 if (brake){ 00419 lcd.SetTextColor(LCD_COLOR_RED); 00420 lcd.SetBackColor(LCD_COLOR_RED); 00421 lcd.FillRect(560,340,230,68); 00422 PrintString("BRAKE",50,575,350,LCD_COLOR_BLACK); 00423 }else { 00424 lcd.SetTextColor(LCD_COLOR_DARKRED); 00425 lcd.SetBackColor(LCD_COLOR_DARKRED); 00426 lcd.FillRect(560,340,230,68); 00427 PrintString("BRAKE",50,575,350,LCD_COLOR_BLACK); 00428 }; 00429 lcd.SetBackColor(LCD_COLOR_WHITE); 00430 }; 00431 00432 00433 void TestFont(){ 00434 uint16_t XPos=0,YPos=120; 00435 for(int k=0;k<68;k++){ 00436 PrintChar(*font50[k],XPos,YPos,lcd.GetTextColor()); 00437 XPos=XPos+(*font50[k]).width; 00438 wait(0.1); 00439 //pc.printf("%d",k); 00440 }; 00441 };
Generated on Thu Jul 14 2022 09:25:33 by
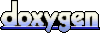