
Proyecto ABInBev para la tarjeta Guaria 1/2.
Embed:
(wiki syntax)
Show/hide line numbers
safety_tip_exe.cpp
Go to the documentation of this file.
00001 /** 00002 * @file safety_tip_exe.cpp 00003 * @author Felícito Manzano (felicito.manzano@detektor.com.sv) 00004 * @brief 00005 * @version 0.1 00006 * @date 2021-01-26 00007 * 00008 * @copyright Copyright (c) 2021 00009 * 00010 */ 00011 00012 #include "mbed.h" 00013 #include "BufferedSerial.h" 00014 #include "playlist.hpp" 00015 #include "voice_cn_pa.hpp" 00016 #include "jq8400_voice.hpp " 00017 #include "teltonika_fmu130.hpp " 00018 #include "safety_tip_exe.hpp " 00019 #include "USBSerial.h" 00020 00021 #define SAFETY_TIP_ID_BEGIN 27 // Del archivo de definición de audios 00022 #define SAFETY_TIP_ID_END 40 // Del archivo de definición de audios 00023 00024 // Variables de Tip de Seguridad 00025 const char TCA_ID_SAFETY_TIP[] = "TCA|134|2"; 00026 const char TCA_ID_FRI_ICS5[] = "TCA|150|1"; 00027 const char TCA_ID_OFF_ICS5[] = "TCA|150|0"; 00028 const char TCA_ID_GPIO_UPDATE[] = "TCA|159|"; 00029 00030 const int safety_tip_min = SAFETY_TIP_ID_BEGIN; 00031 const int safety_tip_max = SAFETY_TIP_ID_END; 00032 int safety_tip_id = SAFETY_TIP_ID_BEGIN; 00033 char safety_tip_buffer[64]; 00034 00035 // Variables externas 00036 extern BufferedSerial avl_uart; 00037 extern USBSerial myPC_debug; 00038 extern bool estado_actual_ignicion; 00039 extern bool functionality_safety_tip; 00040 extern bool flag_safety_tip; 00041 extern bool flag_fri_ics; 00042 00043 // Entradas digitales externas 00044 extern bool pilot_seatbelt; 00045 extern bool copilot_seatbelt; 00046 extern bool crew_seatbelt; 00047 extern bool rg9_raining; 00048 00049 /** 00050 * @brief 00051 * 00052 */ 00053 void play_SafetyTip() { 00054 flag_safety_tip = true; 00055 } 00056 00057 00058 /** 00059 * @brief 00060 * 00061 */ 00062 void exe_SafetyTip() { 00063 flag_safety_tip = false; 00064 if (functionality_safety_tip) { 00065 //myPC_debug.printf("\r\nTIP - Reproducir Tip de Seguridad\r\n"); 00066 jq8400_addQueue (safety_tip_id); 00067 sprintf(safety_tip_buffer, "%s", TCA_ID_SAFETY_TIP); 00068 //myPC_debug.printf("%s\r\n", safety_tip_buffer); 00069 tx_fmu130_message (safety_tip_buffer, &avl_uart); 00070 safety_tip_id++; 00071 // Mantenerse dentro de la lista de opciones. 00072 if (safety_tip_id >= SAFETY_TIP_ID_END) { 00073 safety_tip_id = SAFETY_TIP_ID_BEGIN; 00074 } 00075 } 00076 } 00077 00078 00079 00080 /** 00081 * @brief 00082 * 00083 */ 00084 void send_fri_ics() { 00085 flag_fri_ics = true; 00086 } 00087 00088 00089 00090 /** 00091 * @brief 00092 * 00093 */ 00094 extern bool estado_actual_cinturon_piloto; 00095 extern bool estado_actual_cinturon_copiloto; 00096 extern bool estado_actual_cinturon_tripulante; 00097 extern bool estado_actual_sensor_lluvia; 00098 00099 void tx_fri_ics() { 00100 flag_fri_ics = false; 00101 // TCA|159|4,101:0,102:1,103:0,120:0 00102 //myPC_debug.printf("\r\nFRI - TX Actualizacion GPIO\r\n"); 00103 sprintf(safety_tip_buffer, "%s4,101:%d,102:%d,103:%d,120:%d", 00104 TCA_ID_GPIO_UPDATE, 00105 estado_actual_cinturon_piloto, estado_actual_cinturon_copiloto, 00106 estado_actual_cinturon_tripulante, estado_actual_sensor_lluvia); 00107 //myPC_debug.printf("%s\r\n", safety_tip_buffer); 00108 tx_fmu130_message (safety_tip_buffer, &avl_uart); 00109 }
Generated on Thu Jul 28 2022 19:27:02 by
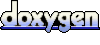