
Proyecto ABInBev para la tarjeta Guaria 1/2.
Embed:
(wiki syntax)
Show/hide line numbers
playlist.hpp
Go to the documentation of this file.
00001 /** 00002 * @file playlist.hpp 00003 * @author Felícito Manzano (felicito.manzano@detektor.com.sv) 00004 * @brief 00005 * https://www.techiedelight.com/queue-implementation-cpp/ 00006 * @version 0.1 00007 * @date 2020-11-19 00008 * 00009 * @copyright Copyright (c) 2020 00010 * 00011 */ 00012 00013 #ifndef __PLAYLIST_H 00014 #define __PLAYLIST_H 00015 00016 #include <iostream> 00017 #include <cstdlib> 00018 using namespace std; 00019 00020 // define default capacity of the queue 00021 #define SIZE 10 00022 00023 // Class for queue 00024 class queue 00025 { 00026 int *arr; // array to store queue elements 00027 int capacity; // maximum capacity of the queue 00028 int front; // front points to front element in the queue (if any) 00029 int rear; // rear points to last element in the queue 00030 int count; // current size of the queue 00031 00032 public: 00033 queue(int size = SIZE); // constructor 00034 ~queue(); // destructor 00035 void dequeue(); 00036 void enqueue(int x); 00037 int peek(); 00038 int size(); 00039 //void erase(); 00040 bool isEmpty(); 00041 bool isFull(); 00042 }; 00043 00044 #endif //__PLAYLIST_H
Generated on Thu Jul 28 2022 19:27:02 by
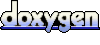