
Proyecto ABInBev para la tarjeta Guaria 1/2.
Embed:
(wiki syntax)
Show/hide line numbers
playlist.cpp
Go to the documentation of this file.
00001 /** 00002 * @file playlist.cpp 00003 * @author Felícito Manzano (felicito.manzano@detektor.com.sv) 00004 * @brief 00005 * @version 0.1 00006 * @date 2020-11-19 00007 * 00008 * @copyright Copyright (c) 2020 00009 * 00010 */ 00011 00012 #include "playlist.hpp" 00013 extern int items_queue; 00014 00015 // Constructor to initialize queue 00016 queue::queue(int size) 00017 { 00018 arr = new int[size]; 00019 capacity = size; 00020 front = 0; 00021 rear = -1; 00022 count = 0; 00023 } 00024 00025 // Destructor to free memory allocated to the queue 00026 queue::~queue() 00027 { 00028 delete[] arr; 00029 } 00030 00031 // Utility function to remove front element from the queue 00032 void queue::dequeue() 00033 { 00034 // check for queue underflow 00035 if (isEmpty()) 00036 { 00037 //cout << "UnderFlow\nProgram Terminated\n"; 00038 exit(EXIT_FAILURE); 00039 } 00040 00041 //cout << "Removing " << arr[front] << '\n'; 00042 00043 front = (front + 1) % capacity; 00044 count--; 00045 items_queue--; 00046 } 00047 00048 // Utility function to add an item to the queue 00049 void queue::enqueue(int item) 00050 { 00051 // check for queue overflow 00052 if (isFull()) 00053 { 00054 // cout << "OverFlow\nProgram Terminated\n"; 00055 exit(EXIT_FAILURE); 00056 } 00057 00058 //cout << "Inserting " << item << '\n'; 00059 00060 rear = (rear + 1) % capacity; 00061 arr[rear] = item; 00062 count++; 00063 items_queue++; 00064 } 00065 00066 // Utility function to return front element in the queue 00067 int queue::peek() 00068 { 00069 if (isEmpty()) 00070 { 00071 //cout << "UnderFlow\nProgram Terminated\n"; 00072 exit(EXIT_FAILURE); 00073 } 00074 return arr[front]; 00075 } 00076 00077 // Utility function to return the size of the queue 00078 int queue::size() 00079 { 00080 return count; 00081 } 00082 00083 // Funcion agregada para limpiar items directamente 00084 /* 00085 void queue::erase() 00086 { 00087 memset(arr, '\0', sizeof(arr)); 00088 front = 0; 00089 rear = -1; 00090 count = 0; 00091 00092 } 00093 */ 00094 00095 // Utility function to check if the queue is empty or not 00096 bool queue::isEmpty() 00097 { 00098 return (size() == 0); 00099 } 00100 00101 // Utility function to check if the queue is full or not 00102 bool queue::isFull() 00103 { 00104 return (size() == capacity); 00105 }
Generated on Thu Jul 28 2022 19:27:02 by
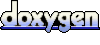