
Proyecto ABInBev para la tarjeta Guaria 1/2.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
Go to the documentation of this file.
00001 /** 00002 * @file main.cpp 00003 * @author Felícito Manzano (felicito.manzano@detektor.com.sv) 00004 * @brief 00005 * Proyecto para ABInBev Cervecería Nacional de Panamá, incluye el soporte 00006 * para para 5 entradas digitales, conexión vía Bluetooth SPP con equipo 00007 * Teltonika FMU130 para el envío y recepción de mensajes al servidor 00008 * del ecosistema Detektor, también incluye un puerto serial TTL para 00009 * controlar el módulo de voz JQ8400, puerto serial para el lector de 00010 * huellas GT521Fx y puerto serial RS232 con el sensor de lluvia RG9. 00011 * @version 0.1 00012 * @date 2020-09-26 00013 * 00014 * @copyright Copyright (c) 2020 00015 * 00016 */ 00017 00018 #include "mbed.h" 00019 //#include "platform/mbed_thread.h" 00020 #include "stm32f4xx_hal_iwdg.h" 00021 #include "BufferedSerial.h" 00022 #include "ics5_pinout.hpp" 00023 #include "jq8400_voice.hpp " 00024 #include "voice_cn_pa.hpp" 00025 #include "New_GT521Fx.hpp " 00026 #include "gpio_exe.hpp " 00027 #include "fmu130_exe.hpp " 00028 #include "fingerprint_exe.hpp " 00029 #include "playlist_exe.hpp " 00030 #include "playlist.hpp" 00031 #include "teltonika_fmu130.hpp " 00032 #include "fireup_exe.hpp " 00033 #include "safety_tip_exe.hpp " 00034 #include "eeprom.h" 00035 #include "flash_eeprom.h" 00036 #include "eeprom_exe.hpp" 00037 #include "flash_ee_exe.hpp " 00038 #include "USBSerial.h" 00039 #include "custom_def.hpp " 00040 #include "flash_eeprom.h" 00041 #include <ctype.h> 00042 00043 // Las funciones de FLASH son arreglos 00044 uint16_t VirtAddVarTab[NB_OF_VAR] = {ADDR_FINGERPRINT, 00045 ADDR_OVERRIDE_FP, 00046 ADDR_OVERRIDE_QT, 00047 ADDR_IDLE_SHUTDOWN, 00048 ADDR_IDLE_REMINDER, 00049 ADDR_IDLE_S_TIME, 00050 ADDR_IDLE_R_TIME, 00051 ADDR_SEATBELT_READ, 00052 ADDR_ENFORCE_SB, 00053 ADDR_PILOT_BTYPE, 00054 ADDR_COPILOT_BTYPE, 00055 ADDR_CREW_BTYPE, 00056 ADDR_RAINSENSOR, 00057 ADDR_RS_SILENTMODE, 00058 ADDR_RS_SAMPLE_T, 00059 ADDR_WET_LIMIT, 00060 ADDR_WET_WARNING, 00061 ADDR_DRY_LIMIT, 00062 ADDR_DRY_WARNING, 00063 ADDR_SAFETY_TIP, 00064 ADDR_SAFETY_TIP_T, 00065 ADDR_GEOZONE, 00066 ADDR_VOLUME, 00067 ADDR_BLE_RESET, 00068 ADDR_BLE_RESET_T}; 00069 uint16_t VarDataTab[NB_OF_VAR] = {0}; 00070 00071 /** 00072 * INTERFACES: 00073 * Se utiliza la librería ICS5_Pinout para hacer el cambio de pines 00074 * en base a la tarjeta para la que se compilará. Se tiene soporte para 00075 * las tarjetas NUCLEO F303K8, NUCLEO F091RC, ICS3 e ICS5. 00076 */ 00077 #define M_DEBUG 1 00078 #define FP_READER_GT521 1 00079 extern const bool external_eeprom; 00080 00081 #if (ICS_BOARD_ID == 11) 00082 // Serial 00083 BufferedSerial avl_uart(BLE_TX, BLE_RX, 1024, 2); //! UART TTL para enlace con el equipo Teltonika FMU130. 00084 BufferedSerial voice_uart(TTL_VOICE_TX, TTL_VOICE_RX); //! UART TTL para comunicación con el módulo de voz JQ8400. 00085 00086 #if FP_READER_GT521 == 1 00087 BufferedSerial fingerprint(TTL_TX, TTL_RX); 00088 #else 00089 00090 #endif 00091 #ifdef M_DEBUG 00092 USBSerial myPC_debug; 00093 #endif 00094 00095 // PCB Support 00096 InterruptIn mybutton(USER_BUTTON); 00097 DigitalIn bluetooth_state(BT_STATE); 00098 DigitalOut myled(USER_LED1); 00099 DigitalOut flashLED(USER_LED2); 00100 DigitalOut out2_ble_reset(BT_RESET); 00101 00102 // Outputs 00103 DigitalOut out1_fingerprint(OUTPUT1_NEGATIVE); 00104 DigitalOut out3_gt521fx(OUTPUT2_NEGATIVE); 00105 DigitalOut out4_gt521fx(OUTPUT3_NEGATIVE); 00106 00107 // Inputs 00108 DigitalIn in1_ignition(INPUT1_POSITIVE); 00109 DigitalIn in2_pilot(INPUT2_NEGATIVE); 00110 DigitalIn in3_copilot(INPUT3_NEGATIVE); 00111 DigitalIn in4_crew(INPUT4_NEGATIVE); 00112 DigitalIn in5_rain_sensor(INPUT5_NEGATIVE); 00113 #endif 00114 00115 // EEPROM 00116 EEPROM ep(I2C_SDA_EE, I2C_SCL_EE, 0x0, EEPROM::T24C32); 00117 int32_t eeprom_size,max_size; 00118 #define EEPROM_ADDR 0x0 00119 #define MIN(X,Y) ((X) < (Y) ? (X) : (Y)) 00120 #define MAX(X,Y) ((X) > (Y) ? (X) : (Y)) 00121 typedef struct _MyData { 00122 int16_t sdata; 00123 int32_t idata; 00124 float fdata; 00125 } MyData; 00126 static void myerror(std::string msg) { 00127 printf("Error %s\n",msg.c_str()); 00128 exit(1); 00129 } 00130 00131 // FLASH 00132 /* Start @ of user Flash area */ 00133 #define FLASH_USER_START_ADDR ADDR_FLASH_SECTOR_2 00134 /* End @ of user Flash area : sector start address + sector size -1 */ 00135 #define FLASH_USER_END_ADDR ADDR_FLASH_SECTOR_7 + GetSectorSize(ADDR_FLASH_SECTOR_7) -1 00136 #define DATA_32 ((uint32_t)0x12345678) 00137 00138 // VOICE 00139 queue colaPlaylist(10); 00140 00141 // HAL BASED 00142 static IWDG_HandleTypeDef my_iwdg; 00143 00144 00145 // FUNCIONALIDADES 00146 // Nuevas banderas para habilitar/deshabilitar funciones. 00147 bool functionality_idle_shutdown; 00148 bool functionality_idle_reminder; 00149 bool functionality_seatbelt_reading; 00150 bool functionality_force_driver_buclke; 00151 bool functionality_rain_sensor; 00152 bool functionality_rainSensor_silentMode; 00153 bool functionality_fingerprint_reader; 00154 bool functionality_safety_tip; 00155 bool functionality_geo_warning; 00156 bool functionality_ble_autoreset; 00157 bool fingerprint_remotly_disable; 00158 bool fingerprint_override; 00159 bool finterprint_flag_working = false; 00160 bool fingerprint_flag_poweroff = false; 00161 00162 /** 00163 * BANDERAS: 00164 * Se utiliza un esquema de banderas para indicar el cambio de un estado 00165 * y que se debe realizar un procesamiento especial (transmitir un 00166 * evento, activar un accesorio, etc.) 00167 */ 00168 00169 float time_safety_tip; // 15 minutos = 900 00170 float time_sample_rain; // muestras a 9 equivalen a 90 segundos o minuto y medio. 00171 float time_ble_autoreset; 00172 bool flag_rain_no_answer = false; 00173 bool flag_idle_force_shutdown = false; 00174 bool flag_idle_reminder = false; 00175 bool flag_fingerprint_Sleep = false; 00176 bool flag_driver_loggin = false; 00177 bool flag_playingSound = false; 00178 bool flag_safety_tip = false; 00179 bool rain_sensor_type = false; // True = RS232, False = DIN 00180 bool flag_query_rain_sensor; 00181 bool flag_notify_rain_sensor; 00182 bool flag_read_inputs; 00183 bool flag_fingerprint_turOn; 00184 bool flag_fri_ics; 00185 int flag_fingerprint_query; 00186 int flag_ignition; 00187 int flag_pilot_seatbelt; 00188 int flag_copilot_seatbelt; 00189 int flag_crew_pilot_seatbelt; 00190 int flag_ble_connection_state; 00191 int flag_rainsensorDigInput; 00192 00193 /** 00194 * ENTRADAS: 00195 * Para la lectura de entradas digitales se utiliza un estructura de 4 00196 * variables que incluyen el estado actual de la entrada, el estado previo 00197 * de la entrada, un arreglo de cuatro posiciones para tomar muestras y 00198 * realizar la función de debug y un contador para indicar la posición en 00199 * el arreglo para capturar la muestra de la entrada. 00200 */ 00201 bool ignition; 00202 bool estado_actual_ignicion; 00203 bool ignition_prev; 00204 bool ignition_samples[4]; 00205 int ignition_counter; 00206 00207 bool pilot_seatbelt; 00208 bool pilot_seatbelt_prev; 00209 bool pilot_seatbelt_samples[4]; 00210 int pilot_seatbelt_c; 00211 00212 bool copilot_seatbelt; 00213 bool copilot_seatbelt_prev; 00214 bool copilot_seatbelt_samples[4]; 00215 int copilot_seatbelt_c; 00216 00217 bool crew_seatbelt; 00218 bool crew_seatbelt_prev; 00219 bool crew_seatbelt_samples[4]; 00220 int crew_seatbelt_c; 00221 00222 bool ble_connection_state; 00223 bool ble_connection_state_prev; 00224 bool ble_connection_state_samples[4]; 00225 int ble_connection_state_samples_c; 00226 00227 bool rg9_raining; 00228 bool rg9_raining_prev; 00229 bool rg9_raining_samples[10]; 00230 int rg9_raining_samples_c; 00231 00232 00233 /** 00234 * FMU130: 00235 * Se definen las variables para recepción de datos del equipo FMU130 vía 00236 * enlace Bluetooth SPP 00237 */ 00238 char fmu130_payload[1024]; 00239 int fmu130_payload_type; 00240 int incoming_bytes; 00241 int temp_JQ8400_Volume; 00242 00243 /** 00244 * HUELLAS: 00245 * Se definen las variables para el procesamiento del módulo lector de 00246 * huellas conectado a un UART-TTL 00247 */ 00248 int bluetooth_cmd_id; 00249 int fingerprint_id; 00250 int fingerprint_login; 00251 char fingerprint_hex[997]; 00252 char fingerprint_asc[499]; 00253 char fingerprint_cmd[4]; 00254 00255 /** 00256 * EVENTOS_TELTONIKA: 00257 * Se definen las variables para el procesamiento de Tramas del equipo 00258 * Teltonika FMU130 con eventos como los hábitos de manejo. 00259 */ 00260 char avl_fmu130_header[2]; 00261 char avl_fmu130_imei[16]; 00262 int avl_fmu130_id; 00263 int avl_fmu130_status; 00264 00265 // Inicialización de variables de Velocidad 00266 int wet_Speed_Warning; 00267 int wet_Speed_Limit; 00268 int dry_Speed_Warning; 00269 int dry_Speed_Limit; 00270 char wet_Speed_CMD[30]; 00271 char dry_Speed_CMD[30]; 00272 00273 // Fingerprint Override 00274 int fp_override_limit; // Limite de lecturas fallidas antes de autorizar 00275 int fp_unauthorized_count = 0; 00276 int items_queue = 0; 00277 00278 // Inicialización de Entradas Digitales para Cinturón de Seguridad 00279 // Detalle de entradas por cada cinturon 00280 bool pilot_buckle_type; 00281 int pilot_buckleUp; 00282 int pilot_unfasten; 00283 bool copilot_buckle_type; 00284 int copilot_buckleUp; 00285 int copilot_unfasten; 00286 bool crew_buckle_type; 00287 int crew_buckleUp; 00288 int crew_unfasten; 00289 00290 /** 00291 * TICKER DE mbed: 00292 * Definición de eventos gestionados por eventos temporizados. 00293 */ 00294 Ticker tick_blink; 00295 Ticker tick_rain_sensor; // para comunicación vía UART 00296 Ticker tick_readInputs; 00297 Ticker tick_fingerprint; 00298 Ticker tick_idle_shutdown; 00299 Ticker tick_idle_reminder; 00300 Ticker tick_safety_tip; 00301 Ticker tick_playingAudio; 00302 Ticker tick_fri_ics; 00303 Ticker tick_autoreset_ble; 00304 00305 /** 00306 * @brief Función para hacer titilar el LED de usuario. 00307 * 00308 */ 00309 void blink_led() { 00310 myled = !myled; 00311 } 00312 00313 /** 00314 * @brief Activación de la bandera para leer entradas digitales. 00315 * 00316 */ 00317 void readInputs() { 00318 flag_read_inputs = true; 00319 } 00320 00321 // Función al presionar boton 00322 void pressed() { 00323 if (external_eeprom) { 00324 eeprom_Default(); 00325 } else { 00326 flash_Default(); 00327 } 00328 wait_ms(100); 00329 NVIC_SystemReset(); 00330 } 00331 00332 int main () { 00333 00334 /** 00335 * @brief INICIAR INTERFACES 00336 * Se define el baudrate para las interfaces USART, y el modo para 00337 * las entradas digitales. También se envía a tierra el pin para la 00338 * interface Bluetooth. 00339 */ 00340 avl_uart.baud(115200); 00341 #if FP_READER_GT521 == 1 00342 fingerprint.baud(9600); 00343 #else 00344 00345 #endif 00346 voice_uart.baud(9600); 00347 in1_ignition.mode(PullDown); 00348 in2_pilot.mode(PullDown); 00349 in3_copilot.mode(PullDown); 00350 in4_crew.mode(PullDown); 00351 in5_rain_sensor.mode(PullDown); 00352 wait_us(1000000); 00353 out1_fingerprint = 0; 00354 out2_ble_reset = 0; 00355 out3_gt521fx = 0; 00356 out4_gt521fx = 0; 00357 mybutton.fall(&pressed); 00358 00359 //Load Flash 00360 boot_message(); 00361 if (external_eeprom) { 00362 eeprom_size = ep.getSize(); 00363 load_eepromVar(); 00364 } else { 00365 readFlash_Booting(); 00366 } 00367 00368 // Configuración Inicial de Nivel de volumen. 00369 jq8400_iniVol (temp_JQ8400_Volume, &voice_uart); 00370 00371 // Iniciar comandos variables de cambio de velocidad 00372 sprintf(dry_Speed_CMD, "setparam 11104:%d;50092:%d", dry_Speed_Warning, dry_Speed_Limit); 00373 sprintf(wet_Speed_CMD, "setparam 11104:%d;50092:%d", wet_Speed_Warning, wet_Speed_Limit); 00374 00375 /** 00376 * @brief Se asignan las funciones para los eventos temporizados de titilar 00377 * el LED de usuario, realizar el muestreo de las entradas digitales 00378 * y la consulta al sensor de lluvia. 00379 */ 00380 tick_blink.attach(&blink_led, 2.0); 00381 tick_readInputs.attach(&readInputs, 0.250); 00382 if (functionality_rain_sensor) { 00383 tick_rain_sensor.attach(&queryRG9, time_sample_rain); 00384 } 00385 if (functionality_ble_autoreset) { 00386 tick_autoreset_ble.attach(&BLE_reset, time_ble_autoreset); 00387 } 00388 00389 /** 00390 * @brief INICIAR WATCHDOG 00391 * Se crea una instancia para el Watchdog, se define el pre-escaler 00392 * y el valor máximo en caso que no se actualice se genera el reinicio. 00393 */ 00394 my_iwdg.Instance = IWDG; 00395 my_iwdg.Init.Prescaler = IWDG_PRESCALER_32; 00396 my_iwdg.Init.Reload = 0x0FFF; 00397 HAL_IWDG_Init(&my_iwdg); 00398 00399 while (true) { 00400 00401 //! Procesamiento del puerto serial vinculado al FMU130 vía Bluetooth. 00402 process_FMU130 (); 00403 00404 //! Verificar si deben efectuarse la lectura de entradas digitales. 00405 if(flag_read_inputs) { 00406 process_Inputs (); 00407 } 00408 00409 //! Verificar si debe activarse el lector de huellas. 00410 if (flag_fingerprint_query) { 00411 HAL_IWDG_Refresh(&my_iwdg); 00412 #if FP_READER_GT521 == 1 00413 identify_fingerPrint(); 00414 #else 00415 00416 #endif 00417 } 00418 00419 //! Verificar si se debe apagar el lector de huellas. 00420 if (fingerprint_flag_poweroff) { 00421 out3_gt521fx = out4_gt521fx = finterprint_flag_working = fingerprint_flag_poweroff = false; 00422 #ifdef M_DEBUG 00423 myPC_debug.printf("[MAIN] - OUT3:FALSE\r\n"); 00424 #endif 00425 } 00426 00427 //! Verificar si debe consultarse el estado del sensor de lluvia. 00428 if (flag_query_rain_sensor) { 00429 //process_rainSensor(); 00430 process_inputRainSensor(); 00431 } 00432 00433 //! verificar si debe ejecutarse un evento de apagado por ralentí. 00434 if (flag_idle_force_shutdown) { 00435 exe_idle_shutdown(); 00436 } 00437 00438 if (flag_idle_reminder) { 00439 exe_idle_reminder(); 00440 } 00441 00442 if (flag_safety_tip) { 00443 exe_SafetyTip(); 00444 } 00445 00446 if (flag_fri_ics) { 00447 tx_fri_ics(); 00448 } 00449 00450 if (!(flag_playingSound)) { 00451 processPlaylist(); 00452 } 00453 00454 //! Actualizar Watchdog 00455 HAL_IWDG_Refresh(&my_iwdg); 00456 } 00457 }
Generated on Thu Jul 28 2022 19:27:02 by
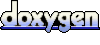