
Proyecto ABInBev para la tarjeta Guaria 1/2.
Embed:
(wiki syntax)
Show/hide line numbers
gpio_exe.cpp
Go to the documentation of this file.
00001 /** 00002 * @file gpio_exe.cpp 00003 * @author Felícito Manzano (felicito.manzano@detektor.com.sv) 00004 * @brief 00005 * @version 0.1 00006 * @date 2020-10-01 00007 * 00008 * @copyright Copyright (c) 2020 00009 * 00010 */ 00011 00012 #include "mbed.h" 00013 #include "inputDebounce.hpp " 00014 #include "BufferedSerial.h" 00015 #include "New_GT521Fx.hpp " 00016 #include "jq8400_voice.hpp " 00017 #include "voice_cn_pa.hpp" 00018 #include "teltonika_fmu130.hpp " 00019 #include "gpio_exe.hpp " 00020 #include "fireup_exe.hpp " 00021 #include "safety_tip_exe.hpp " 00022 #include "USBSerial.h" 00023 00024 00025 //Objetos Entradas Digitales / Seriales 00026 /** 00027 * @brief 00028 * 00029 */ 00030 extern BufferedSerial avl_uart; 00031 extern BufferedSerial voice_uart; 00032 extern BufferedSerial fingerprint; 00033 extern DigitalIn in1_ignition; 00034 extern DigitalIn in2_pilot; 00035 extern DigitalIn in3_copilot; 00036 extern DigitalIn in4_crew; 00037 extern DigitalIn in5_rain_sensor; 00038 extern DigitalIn bluetooth_state; 00039 extern USBSerial myPC_debug; 00040 extern Ticker tick_fingerprint; 00041 extern Ticker tick_safety_tip; 00042 extern Ticker tick_fri_ics; 00043 00044 00045 // Variables Externas 00046 /** 00047 * @brief 00048 * 00049 */ 00050 extern bool ignition; 00051 extern bool ignition_prev; 00052 extern bool ignition_samples[4]; 00053 extern int ignition_counter; 00054 00055 extern bool pilot_seatbelt; 00056 extern bool pilot_seatbelt_prev; 00057 extern bool pilot_seatbelt_samples[4]; 00058 extern int pilot_seatbelt_c; 00059 00060 extern bool copilot_seatbelt; 00061 extern bool copilot_seatbelt_prev; 00062 extern bool copilot_seatbelt_samples[4]; 00063 extern int copilot_seatbelt_c; 00064 00065 extern bool crew_seatbelt; 00066 extern bool crew_seatbelt_prev; 00067 extern bool crew_seatbelt_samples[4]; 00068 extern int crew_seatbelt_c; 00069 00070 extern bool ble_connection_state; 00071 extern bool ble_connection_state_prev; 00072 extern bool ble_connection_state_samples[4]; 00073 extern int ble_connection_state_samples_c; 00074 00075 extern bool rg9_raining; 00076 extern bool rg9_raining_prev; 00077 extern bool rg9_raining_samples[4]; 00078 extern int rg9_raining_samples_c; 00079 00080 extern int flag_fingerprint_turOn; 00081 extern int flag_fingerprint_Sleep; 00082 extern int flag_ignition; 00083 extern int flag_pilot_seatbelt; 00084 extern int flag_copilot_seatbelt; 00085 extern int flag_crew_pilot_seatbelt; 00086 extern int flag_fingerprint_query; 00087 extern int flag_ble_connection_state; 00088 00089 extern bool functionality_fingerprint_reader; 00090 extern bool fingerprint_remotly_disable; 00091 extern bool flag_driver_loggin; 00092 extern bool finterprint_flag_working; 00093 extern bool fingerprint_flag_poweroff; 00094 extern bool functionality_force_driver_buclke; 00095 extern bool functionality_seatbelt_reading; 00096 extern bool functionality_safety_tip; 00097 extern bool flag_read_inputs; 00098 extern bool flag_query_rain_sensor; 00099 extern bool functionality_rain_sensor; 00100 extern bool functionality_rainSensor_silentMode; 00101 extern int flag_rainsensorDigInput; 00102 00103 extern char wet_Speed_CMD[30]; 00104 extern char dry_Speed_CMD[30]; 00105 extern int pilot_buckleUp; 00106 extern int pilot_unfasten; 00107 extern int copilot_buckleUp; 00108 extern int copilot_unfasten; 00109 extern int crew_buckleUp; 00110 extern int crew_unfasten; 00111 00112 // Variables locales 00113 /** 00114 * @brief 00115 * 00116 */ 00117 char gpio_buffer[64]; 00118 bool estado_actual_cinturon_piloto; 00119 bool estado_actual_cinturon_copiloto; 00120 bool estado_actual_cinturon_tripulante; 00121 bool estado_actual_sensor_lluvia; 00122 extern bool estado_actual_ignicion; 00123 const char TCA_ID_PILOTO[] = "TCA|101|"; 00124 const char TCA_ID_COPILOTO[] = "TCA|102|"; 00125 const char TCA_ID_TRIPULANTE[] = "TCA|103|"; 00126 const char TCA_ID_LECTOR_HUE[] = "TCA|111|"; 00127 const char TCA_ID_BLUETOOTH[] = "TCA|124|"; 00128 const char TCA_ID_WEATHER[] = "TCA|120|"; 00129 const char IO_ENGINE_LOCK[] = "setdigout 0?? ? ? ? ? ? ?"; 00130 const char IO_ENGINE_UNLOCK[] = "setdigout 1?? ? ? ? ? ? ?"; 00131 00132 #define DEBUG_GPIO_CPP 00133 #define IO_FP_READER_GT521 1 00134 00135 /** 00136 * @brief 00137 * 00138 */ 00139 void process_Inputs () { 00140 flag_read_inputs = false; 00141 memset(gpio_buffer, '\0', sizeof(gpio_buffer)); 00142 00143 00144 /** 00145 * @brief 00146 * 00147 */ 00148 flag_ignition = exeIgnition (&in1_ignition, ignition_samples, &ignition, &ignition_prev, &ignition_counter); 00149 00150 /** 00151 * @brief Construct a new if object 00152 * 00153 */ 00154 if (flag_ignition == 1) { 00155 #ifdef DEBUG_GPIO_CPP 00156 myPC_debug.printf("\r\nGPIO - Ign ON\r\n"); 00157 #endif 00158 estado_actual_ignicion = true; 00159 tick_fri_ics.detach(); 00160 tick_fri_ics.attach(&send_fri_ics, 300.0); 00161 process_Engine_FireUp(); 00162 if (rg9_raining) { 00163 if (!(functionality_rainSensor_silentMode)) { 00164 jq8400_addQueue (VOICE_TRACK_CONDITION_WET); 00165 } 00166 } 00167 00168 } else if (flag_ignition == -1) { 00169 jq8400_addQueue (VOICE_DOOR_SAFETY_REMINDER); 00170 tick_fri_ics.detach(); 00171 tick_fri_ics.attach(&send_fri_ics, 1800.0); 00172 if (functionality_fingerprint_reader) { 00173 #if IO_FP_READER_GT521 == 1 00174 FP_Close(); 00175 #else 00176 #endif 00177 wait_us(1000000); 00178 #ifdef DEBUG_GPIO_CPP 00179 myPC_debug.printf("GPIO - Pendiente apagar FP GT521Fx...\r\n"); 00180 #endif 00181 fingerprint_flag_poweroff = true; 00182 } 00183 #ifdef DEBUG_GPIO_CPP 00184 myPC_debug.printf("GPIO - Ign OFF\r\n"); 00185 #endif 00186 estado_actual_ignicion = false; 00187 flag_driver_loggin = false; 00188 if (functionality_force_driver_buclke || functionality_fingerprint_reader) { 00189 tx_fmu130_command(IO_ENGINE_LOCK, &avl_uart); // No se debe enviar a desactivar la entrada. 00190 #ifdef DEBUG_GPIO_CPP 00191 myPC_debug.printf("%s\r\n", IO_ENGINE_LOCK); 00192 #endif 00193 } 00194 flag_fingerprint_turOn = false; 00195 tick_fingerprint.detach(); 00196 if (functionality_safety_tip) { 00197 tick_safety_tip.detach(); 00198 #ifdef DEBUG_GPIO_CPP 00199 myPC_debug.printf("GPIO - Ticker de Tip de Seguridad Detenido\r\n"); 00200 #endif 00201 } 00202 sprintf(gpio_buffer, "%s3", TCA_ID_LECTOR_HUE); 00203 tx_fmu130_message (gpio_buffer, &avl_uart); 00204 #ifdef DEBUG_GPIO_CPP 00205 myPC_debug.printf("%s\r\n", gpio_buffer); 00206 #endif 00207 // Se deja activado el lector para la siguiente interacción 00208 if (!(fingerprint_remotly_disable)) { 00209 #ifdef DEBUG_GPIO_CPP 00210 myPC_debug.printf("GPIO - FP Activado automaticamente\r\n"); 00211 myPC_debug.printf("%s\r\n", IO_ENGINE_LOCK); 00212 #endif 00213 functionality_fingerprint_reader = true; 00214 tx_fmu130_command(IO_ENGINE_LOCK, &avl_uart); // No se debe enviar a desactivar la entrada. 00215 } else { 00216 #ifdef DEBUG_GPIO_CPP 00217 myPC_debug.printf("GPIO - FP Desactivado remotamente\r\n"); 00218 #endif 00219 } 00220 } else { 00221 #ifdef DEBUG_GPIO_CPP 00222 myPC_debug.printf("GPIO - Ign sin cambio:%d\r\n", ignition); 00223 #endif 00224 } 00225 00226 00227 /** 00228 * @brief 00229 * 00230 */ 00231 flag_ble_connection_state = exeDigInput (&bluetooth_state, ble_connection_state_samples, &ble_connection_state, &ble_connection_state_prev, &ble_connection_state_samples_c); 00232 if (flag_ble_connection_state == 1) { 00233 //jq8400_addQueue(VOICE_BLE_FMU130_CONNECTED); 00234 flag_ble_connection_state = 0; 00235 sprintf(gpio_buffer, "%s1", TCA_ID_BLUETOOTH); 00236 tx_fmu130_message (gpio_buffer, &avl_uart); 00237 #ifdef DEBUG_GPIO_CPP 00238 myPC_debug.printf("GPIO - Bluetooth Conectado\r\n"); 00239 myPC_debug.printf("%s\r\n", gpio_buffer); 00240 #endif 00241 } else if (flag_ble_connection_state == -1) { 00242 //jq8400_addQueue(VOICE_BLE_FMU130_DISCONNECTED); 00243 flag_ble_connection_state = 0; 00244 sprintf(gpio_buffer, "%s0", TCA_ID_BLUETOOTH); 00245 tx_fmu130_message (gpio_buffer, &avl_uart); 00246 #ifdef DEBUG_GPIO_CPP 00247 myPC_debug.printf("GPIO - Bluetooth Desconectado\r\n"); 00248 myPC_debug.printf("%s\r\n", gpio_buffer); 00249 #endif 00250 } 00251 00252 if (functionality_seatbelt_reading) { 00253 /** 00254 * @brief 00255 * CAMIONES: INTER, KENWORK, FREIGHT 00256 * Normalmente Cerrado 00257 * Abrochado = -1 (buckleUp) 00258 * Desabrochado = 1 (unfasten) 00259 * 00260 * Livianos y cinturones Chinos: 00261 * Normalmente Abierto 00262 * Abrochado = 1 00263 * Desabrochado = -1 00264 */ 00265 00266 00267 /** 00268 * @brief 00269 * Lectura del Cinturón del piloto 00270 * 00271 */ 00272 flag_pilot_seatbelt = exeDigInput (&in2_pilot, pilot_seatbelt_samples, &pilot_seatbelt, &pilot_seatbelt_prev, &pilot_seatbelt_c); 00273 if (flag_pilot_seatbelt == pilot_buckleUp) { 00274 estado_actual_cinturon_piloto = true; 00275 #ifdef DEBUG_GPIO_CPP 00276 myPC_debug.printf("GPIO - Piloto Abrochado\r\n"); 00277 #endif 00278 if (estado_actual_ignicion) { 00279 jq8400_addQueue (VOICE_DRIVER_SEATBELT_FASTENED); 00280 } 00281 flag_pilot_seatbelt = 0; 00282 process_SeatBelt_FireUp(); 00283 sprintf(gpio_buffer, "%s1", TCA_ID_PILOTO); 00284 tx_fmu130_message (gpio_buffer, &avl_uart); 00285 #ifdef DEBUG_GPIO_CPP 00286 myPC_debug.printf("%s\r\n", gpio_buffer); 00287 #endif 00288 00289 } else if (flag_pilot_seatbelt == pilot_unfasten) { 00290 estado_actual_cinturon_piloto = false; 00291 #ifdef DEBUG_GPIO_CPP 00292 myPC_debug.printf("GPIO - Piloto Desabrochado\r\n"); 00293 #endif 00294 if (estado_actual_ignicion) { 00295 jq8400_addQueue (VOICE_DRIVER_SEATBELT_UNFASTENED); // Evaluar si se corta el audio. 00296 } 00297 flag_pilot_seatbelt = 0; 00298 sprintf(gpio_buffer, "%s0", TCA_ID_PILOTO); 00299 #ifdef DEBUG_GPIO_CPP 00300 myPC_debug.printf("%s\r\n", gpio_buffer); 00301 #endif 00302 tx_fmu130_message (gpio_buffer, &avl_uart); 00303 if (functionality_fingerprint_reader && functionality_force_driver_buclke) { ///// PENDIENTE 00304 #if IO_FP_READER_GT521 == 1 00305 FP_Close(); 00306 #else 00307 #endif 00308 wait_us(1000000); 00309 #ifdef DEBUG_GPIO_CPP 00310 myPC_debug.printf("GPIO - Pendiente apagar FP GT521Fx...\r\n"); 00311 #endif 00312 fingerprint_flag_poweroff = true; 00313 } 00314 } 00315 00316 00317 /** 00318 * @brief 00319 * 00320 */ 00321 flag_copilot_seatbelt = exeDigInput (&in3_copilot, copilot_seatbelt_samples, &copilot_seatbelt, &copilot_seatbelt_prev, &copilot_seatbelt_c); 00322 if (flag_copilot_seatbelt == copilot_buckleUp) { 00323 estado_actual_cinturon_copiloto = true; 00324 #ifdef DEBUG_GPIO_CPP 00325 myPC_debug.printf("GPIO - Copiloto Abrochado\r\n"); 00326 #endif 00327 if (estado_actual_ignicion) { 00328 jq8400_addQueue (VOICE_COPILOT_SEATBELT_FASTENED); 00329 } 00330 flag_copilot_seatbelt = 0; 00331 sprintf(gpio_buffer, "%s1", TCA_ID_COPILOTO); 00332 #ifdef DEBUG_GPIO_CPP 00333 myPC_debug.printf("%s\r\n", gpio_buffer); 00334 #endif 00335 tx_fmu130_message (gpio_buffer, &avl_uart); 00336 } else if (flag_copilot_seatbelt == copilot_unfasten) { 00337 estado_actual_cinturon_copiloto = false; 00338 #ifdef DEBUG_GPIO_CPP 00339 myPC_debug.printf("GPIO - copiloto Desabrochado\r\n"); 00340 #endif 00341 if (estado_actual_ignicion) { 00342 jq8400_addQueue (VOICE_COPILOT_SEATBELT_UNFASTENED); 00343 } 00344 flag_copilot_seatbelt = 0; 00345 sprintf(gpio_buffer, "%s0", TCA_ID_COPILOTO); 00346 tx_fmu130_message (gpio_buffer, &avl_uart); 00347 #ifdef DEBUG_GPIO_CPP 00348 myPC_debug.printf("%s\r\n", gpio_buffer); 00349 #endif 00350 } 00351 00352 00353 /** 00354 * @brief 00355 * 00356 */ 00357 flag_crew_pilot_seatbelt = exeDigInput (&in4_crew, crew_seatbelt_samples, &crew_seatbelt, &crew_seatbelt_prev, &crew_seatbelt_c); 00358 if (flag_crew_pilot_seatbelt == crew_buckleUp) { 00359 estado_actual_cinturon_tripulante = true; 00360 #ifdef DEBUG_GPIO_CPP 00361 myPC_debug.printf("GPIO - Tripulante Abrochado\r\n"); 00362 #endif 00363 if (estado_actual_ignicion) { 00364 jq8400_addQueue (VOICE_CREW_SEATBELT_FASTENED); 00365 } 00366 flag_crew_pilot_seatbelt = 0; 00367 sprintf(gpio_buffer, "%s1", TCA_ID_TRIPULANTE); 00368 tx_fmu130_message (gpio_buffer, &avl_uart); 00369 #ifdef DEBUG_GPIO_CPP 00370 myPC_debug.printf("%s\r\n", gpio_buffer); 00371 #endif 00372 } else if (flag_crew_pilot_seatbelt == crew_unfasten) { 00373 estado_actual_cinturon_tripulante = false; 00374 #ifdef DEBUG_GPIO_CPP 00375 myPC_debug.printf("GPIO - Tripulante Desabrochado\r\n"); 00376 #endif 00377 if (estado_actual_ignicion) { 00378 jq8400_addQueue (VOICE_CREW_SEATBELT_UNFASTENED); 00379 } 00380 flag_crew_pilot_seatbelt = 0; 00381 sprintf(gpio_buffer, "%s0", TCA_ID_TRIPULANTE); 00382 #ifdef DEBUG_GPIO_CPP 00383 myPC_debug.printf("%s\r\n", gpio_buffer); 00384 #endif 00385 tx_fmu130_message (gpio_buffer, &avl_uart); 00386 } 00387 } 00388 } 00389 00390 00391 void process_inputRainSensor() { 00392 flag_query_rain_sensor = false; 00393 if (functionality_rain_sensor) { 00394 memset(gpio_buffer, '\0', sizeof(gpio_buffer)); 00395 flag_rainsensorDigInput = exeDigRain (&in5_rain_sensor, rg9_raining_samples, &rg9_raining, &rg9_raining_prev, &rg9_raining_samples_c); 00396 if (flag_rainsensorDigInput == 1) { 00397 estado_actual_sensor_lluvia = true; 00398 #ifdef DEBUG_GPIO_CPP 00399 myPC_debug.printf("GPIO - RG9 Lloviendo \r\n"); 00400 #endif 00401 flag_rainsensorDigInput = 0; 00402 sprintf(gpio_buffer, "%s%d", TCA_ID_WEATHER, 1); 00403 #ifdef DEBUG_GPIO_CPP 00404 myPC_debug.printf("%s\r\n", gpio_buffer); 00405 #endif 00406 tx_fmu130_message (gpio_buffer, &avl_uart); 00407 if (functionality_rainSensor_silentMode) { 00408 #ifdef DEBUG_GPIO_CPP 00409 myPC_debug.printf("GPIO - RG9 Modo silencioso no se notifica voz ni cambia velocidad\r\n"); 00410 #endif 00411 } else { 00412 if (estado_actual_ignicion) { 00413 #ifdef DEBUG_GPIO_CPP 00414 myPC_debug.printf("GPIO - RG9 - Condicion de pista mojada\r\n"); 00415 #endif 00416 jq8400_addQueue (VOICE_TRACK_CONDITION_WET); 00417 } 00418 tx_fmu130_var_command(wet_Speed_CMD, &avl_uart); 00419 } 00420 } else if (flag_rainsensorDigInput == -1) { 00421 estado_actual_sensor_lluvia = false; 00422 #ifdef DEBUG_GPIO_CPP 00423 myPC_debug.printf("GPIO - RG9 Seco\r\n"); 00424 #endif 00425 flag_rainsensorDigInput = 0; 00426 sprintf(gpio_buffer, "%s%d", TCA_ID_WEATHER, 0); 00427 tx_fmu130_message (gpio_buffer, &avl_uart); 00428 #ifdef DEBUG_GPIO_CPP 00429 myPC_debug.printf("%s\r\n", gpio_buffer); 00430 #endif 00431 if (functionality_rainSensor_silentMode) { 00432 #ifdef DEBUG_GPIO_CPP 00433 myPC_debug.printf("GPIO - RG9 Modo silencioso no se notifica voz ni cambia velocidad\r\n"); 00434 #endif 00435 } else { 00436 if (estado_actual_ignicion) { 00437 #ifdef DEBUG_GPIO_CPP 00438 myPC_debug.printf("GPIO - RG9 - Condicion de pista seca\r\n"); 00439 #endif 00440 jq8400_addQueue (VOICE_TRACK_CONDITION_DRY); 00441 } 00442 tx_fmu130_var_command(dry_Speed_CMD, &avl_uart); 00443 } 00444 } 00445 } 00446 }
Generated on Thu Jul 28 2022 19:27:02 by
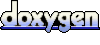