
Proyecto ABInBev para la tarjeta Guaria 1/2.
Embed:
(wiki syntax)
Show/hide line numbers
fingerprint_frame.hpp
Go to the documentation of this file.
00001 /** 00002 * @file fingerprint_frame.hpp 00003 * @author Felícito Manzano (felicito.manzano@detektor.com.sv) 00004 * @brief 00005 * @version 0.1 00006 * @date 2020-09-28 00007 * 00008 * @copyright Copyright (c) 2020 00009 * 00010 */ 00011 00012 #ifndef __FINGERPRINT_FRAME_HPP 00013 #define __FINGERPRINT_FRAME_HPP 00014 00015 00016 // Definiciones 00017 /** 00018 * @brief 00019 * 00020 */ 00021 00022 // Tipo de trama 00023 #define TRAMA_AVL_FMU130 1 00024 #define TRAMA_SERVIDOR 2 00025 00026 // Eventos AVL 00027 #define AVLID_OVERSPEED 24 00028 #define AVLID_TOWING 246 00029 #define AVLID_JAMMING 249 00030 #define AVLID_IDLE 251 00031 #define AVLID_HARSH_BEHAVIOUR 253 00032 #define AVLID_WARNING_SPEED 255 00033 #define AVLID_CRASH 317 00034 00035 // ID Comandos Lector de Huellas 00036 #define CMD_FINGER_ADD 1 00037 #define CMD_FINGER_DELETE 2 00038 #define CMD_FINGER_DISABLE 3 00039 #define CMD_FINGER_ENABLE 4 00040 #define CMD_FINGERPRINT_QUERY 5 00041 #define CMD_FINGER_ENROLL_CNT 6 00042 #define CMD_CHECK_ENROLLED_ID 7 00043 00044 // ID Comandos Anulación de Lector de Huella 00045 #define CMD_FP_OVERRIDE_ENABLE 8 00046 #define CMD_FP_OVERRIDE_DISABLE 9 00047 #define CMD_FP_OVERRIDE_QUERY 10 00048 #define CMD_FP_OVERRIDE_COUNT 11 00049 00050 // ID Comandos de Parlante 00051 #define CMD_SET_VOLUME 12 00052 #define CMD_QUERY_VOLUME_LEVEL 13 00053 00054 // ID Comandos de Sensor de Lluvia 00055 #define CMD_RAIN_ENABLE 14 00056 #define CMD_RAIN_DISABLE 15 00057 #define CMD_RAIN_QUERY 16 00058 #define CMD_CHANGE_WSL 17 00059 #define CMD_CHANGE_WSW 18 00060 #define CMD_CHANGE_DSL 19 00061 #define CMD_CHANGE_DSW 20 00062 #define CMD_QUERY_SPEED_LIMIT 21 00063 #define CMD_CHANGE_RAIN_TIMER 22 00064 #define CMD_QUERY_RAIN_TIMER 23 00065 #define CMD_RAIN_SILENT_MODE_ENA 24 00066 #define CMD_RAIN_SILENT_MODE_DIS 25 00067 #define CMD_RAIN_SILENT_MODE_Q 26 00068 00069 00070 // ID Comandos de Cinturones 00071 #define CMD_SEATBELT_ENABLE 27 00072 #define CMD_SEATBELT_DISABLE 28 00073 #define CMD_SEATBELT_QUERY 29 00074 #define CMD_FORCE_BUCLKE_ENABLE 30 00075 #define CMD_FORCE_BUCLKE_DISABLE 31 00076 #define CMD_QUERY_FORCE_SEATBELT 32 00077 #define CMD_SET_INPUT_NORMAL_O 33 00078 #define CMD_SET_INPUT_NORMAL_C 34 00079 #define CMD_QUERY_DIN_CONFIG 35 00080 #define CMD_PILOT_BUCKLE_NO 36 00081 #define CMD_PILOT_BUCKLE_NC 37 00082 #define CMD_COPILOT_BUCKLE_NO 38 00083 #define CMD_COPILOT_BUCKLE_NC 39 00084 #define CMD_CREW_BUCKLE_NO 40 00085 #define CMD_CREW_BUCKLE_NC 41 00086 #define CMD_QUERY_PILOT_BT 42 00087 #define CMD_QUERY_COPILOT_BT 43 00088 #define CMD_QUERY_CREW_BT 44 00089 00090 00091 // ID Comandos de Apagado por Ralenti 00092 #define CMD_IDLE_SHUTDOWN_ENABLE 45 00093 #define CMD_IDLE_SHUTDOWN_DISABLE 46 00094 #define CMD_IDLE_SHUTDOWN_QUERY 47 00095 #define CMD_QUERY_IDLE_SHUTDOWN_T 48 00096 #define CMD_CHANGE_IDLE_SHUTDOWN_T 49 00097 00098 // ID Comandos de Recordatorio por Ralenti 00099 #define CMD_IDLE_REMINDER_ENABLE 50 00100 #define CMD_IDLE_REMINDER_DISABLE 51 00101 #define CMD_IDLE_REMINDER_QUERY 52 00102 #define CMD_CHANGE_IDLE_REMINDER_T 53 00103 #define CMD_QUERY_IDLE_REMINDER_T 54 00104 00105 // ID Comandos de Tip de Seguridad 00106 #define CMD_SAFETY_TIP_ENABLE 55 00107 #define CMD_SAFETY_TIP_DISABLE 56 00108 #define CMD_SAFETY_TIP_QUERY 57 00109 #define CMD_QUERY_SAFETY_TIP_TIME 58 00110 #define CHANGE_SAFETY_TIP_TIME 59 00111 00112 // ID Comandos de información ICS 00113 #define CMD_QUERY_HARDWARE_VER 60 00114 #define CMD_QUERY_FIRMWARE_VER 61 00115 00116 // ID Comandos para Notificacion de Geocercas 00117 #define CMD_GEO_WARNING_ENABLE 62 00118 #define CMD_GEO_WARNING_DISABLE 63 00119 #define CMD_GEO_WARNING_QUERY 64 00120 #define CMD_GEO_WARNING_GETIN 65 00121 #define CMD_GEO_WARNING_GETOUT 66 00122 #define CMD_GEO_WARNING_EVENT 67 00123 00124 // ID Comandos para Autoreset de Bluetooth 00125 #define CMD_BLE_AUTORESET_ENABLE 68 00126 #define CMD_BLE_AUTORESET_DISABLE 69 00127 #define CMD_BLE_AUTORESET_TIME 70 00128 #define CMD_BLE_AUTORESET_QUERY 71 00129 00130 // Cadenas de comandos 00131 extern const char SERVER_SEPARATOR[2]; 00132 extern const char FINGER_CMD_BEGIN[2]; 00133 extern const char FINGER_CMD_EOF[2]; 00134 00135 // Cadenas CMD de Lector de Huella 00136 extern const char ADD_CMD[4]; 00137 extern const char DEL_CMD[4]; 00138 extern const char FINGERPRINT_DIS_CMD[4]; 00139 extern const char FINGERPRINT_ENA_CMD[4]; 00140 extern const char FINGERPRINT_QRY_CMD[4]; 00141 extern const char FP_OVERRIDE_ENABLE[4]; 00142 extern const char FP_OVERRIDE_DISABLE[4]; 00143 extern const char FP_OVERRIDE_COUNT[4]; 00144 extern const char Q_FP_OVERRIDE_CNT[4]; 00145 00146 // Cadenas CMD de Sensor de Lluvia 00147 extern const char RAIN_ENA_CMD[4]; 00148 extern const char RAIN_DIS_CMD[4]; 00149 extern const char CHANGE_RAIN_TIMER[4]; 00150 extern const char Q_RAIN_TIMER[4]; 00151 extern const char RAIN_SILENT_ENABLE[4]; 00152 extern const char RAIN_SILENT_DISABLE[4]; 00153 extern const char RAIN_SILENT_QUERY[4]; 00154 00155 // Cadenas CMD para Limites de Velocidad 00156 extern const char CHANGE_WSL_TXT[4]; 00157 extern const char CHANGE_WSW_TXT[4]; 00158 extern const char CHANGE_DSL_TXT[4]; 00159 extern const char CHANGE_DSW_TXT[4]; 00160 extern const char QUERY_SPEED_LIMIT[4]; 00161 00162 // Cadenas CMD Cinturones de Seguridad 00163 extern const char SEATBELT_ENA_CMD[4]; 00164 extern const char SEATBELT_DIS_CMD[4]; 00165 extern const char SEATBELT_QUERY_CMD[4]; 00166 extern const char FORCE_BUCLKE_ENA_CMD[4]; 00167 extern const char FORCE_BUCLKE_DIS_CMD[4]; 00168 extern const char INPUT_NORMALLY_OPEN[4]; 00169 extern const char INPUT_NORMALLY_CLOSE[4]; 00170 extern const char Q_DIGITAL_INPUT_CONF[4]; 00171 extern const char PILOT_BUCKLE_N_OPEN[4]; 00172 extern const char PILOT_BUCKLE_N_CLOSE[4]; 00173 extern const char Q_PILOT_BUCLE_TYPE[4]; 00174 extern const char COPILOT_BUCKLE_N_OPEN[4]; 00175 extern const char COPILOT_BUCKLE_N_CLOSE[4]; 00176 extern const char Q_COPILOT_BUCLE_TYPE[4]; 00177 extern const char CREW_BUCKLE_N_OPEN[4]; 00178 extern const char CREW_BUCKLE_N_CLOSE[4]; 00179 extern const char Q_CREW_BUCLE_TYPE[4]; 00180 00181 // Cadenas CMD Apagado por Ralentí 00182 extern const char IDLE_SHUTDOWN_ENA_CMD[4]; 00183 extern const char IDLE_SHUTDOWN_DIS_CMD[4]; 00184 extern const char IDLE_SHUTDOWN_QUERY[4]; 00185 extern const char CHANGE_IDLE_TIMER[4]; 00186 extern const char QUERY_IDLE_TIMER[4]; 00187 00188 // Cadenas CMD Recordatorio por Ralentí 00189 extern const char IDLE_REMINDER_ENABLE[4]; 00190 extern const char IDLE_REMINDER_DISABLE[4]; 00191 extern const char QUERY_IDLE_REMINDER[4]; 00192 extern const char IDLE_REMINDER_TIMER[4]; 00193 extern const char QUERY_IDLE_REMINDER_T[4]; 00194 00195 // Cadenas CMD de Volumen 00196 extern const char VOL_CMD[4]; 00197 00198 // Cadenas CMD para Geocercas 00199 extern const char GEO_WARNING_ENABLE[4]; 00200 extern const char GEO_WARNING_DISABLE[4]; 00201 extern const char Q_GEO_WARNING_CONF[4]; 00202 extern const char GEO_WARNING_GETIN[4]; 00203 extern const char GEO_WARNING_GETOUT[4]; 00204 extern const char GEO_WARNING_EVENT[4]; 00205 00206 // Cadenas CMD para Autoreset de BLE 00207 extern const char BLE_AUTORESET_ENABLE[4]; 00208 extern const char BLE_AUTORESET_DISABLE[4]; 00209 extern const char BLE_AUTORESET_TIME[4]; 00210 extern const char BLE_AUTORESET_QUERY[4]; 00211 00212 // Tramas 00213 extern const int FINGER_CMD_START; 00214 extern const int FINGER_CMD_END; 00215 extern const int FINGER_ID_BEGIN; 00216 extern const int FINGER_ID_END; 00217 extern const int FINGER_PAYLOAD; 00218 extern const int FINGER_PAYLOAD_EOL; 00219 extern const int FINGER_PAYLOAD_START; 00220 extern const int FINGER_PAYLOAD_END; 00221 00222 // Funciones 00223 int strID_to_intID (char cadena[5]); 00224 bool verify_finger_payload (char payload[1011]); 00225 int strID_to_intID (char cadena[5]); 00226 bool parse_finger_payload (char payload[1011], char comando[4], int *id_huella, char huella_hex[997]); 00227 bool fingerprint_HEX2CHAR (char huella_hex[997], char huella_ascii[499]); 00228 int identify_server_cmd (char comando[4]); 00229 00230 #endif // __FINGERPRINT_FRAME_HPP
Generated on Thu Jul 28 2022 19:27:02 by
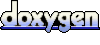